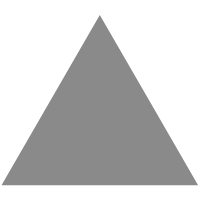
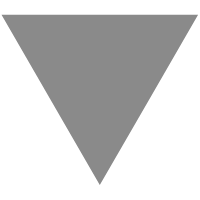
工厂模式
source link: https://youngxhui.top/2020/02/%E5%B7%A5%E5%8E%82%E6%A8%A1%E5%BC%8F/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
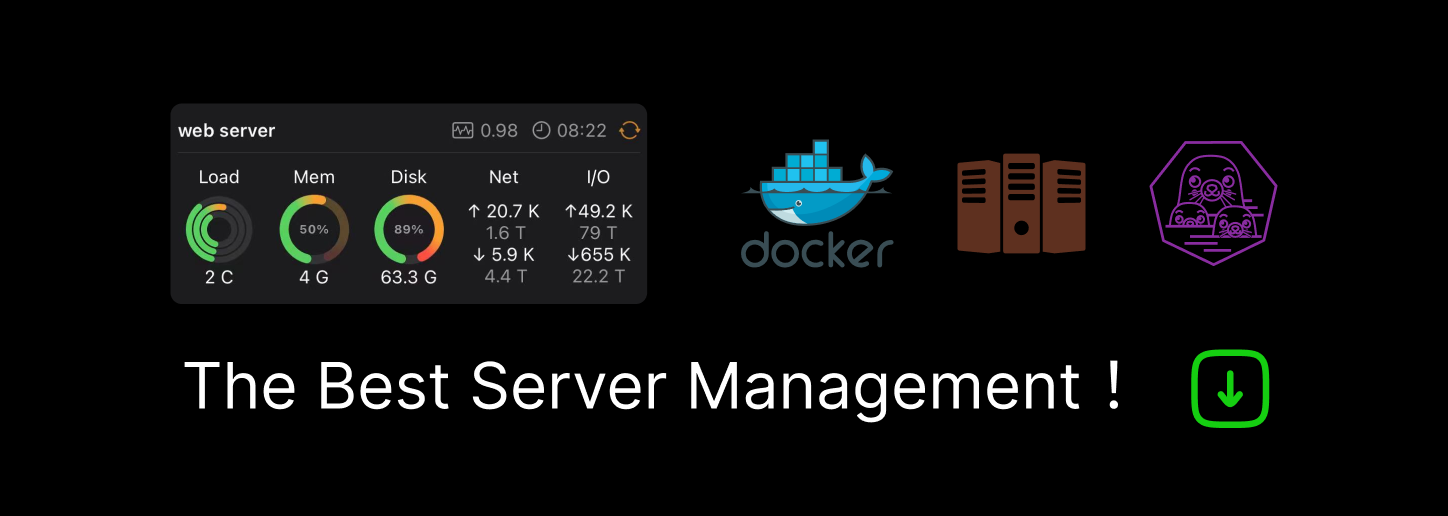
工厂模式是一种对象创造模式。定义一个用户创建对象的接口,让子类决定实例化那些类。
将对象的创建和使用过程分开,并且有时候创建一个对象并不只是单独的调用其类构造函数,我们可能需要进行很多初始化的设置,这样势必会出现代码重复的问题。以后进行修改时,需要多处修改,从而增加维护难度。
参数化工厂
有些地方也叫做个这个方法为简单工厂模式。该模式使得工厂方法可以创建多种产品。采用一个标识要被创建的对象种类,,通过标识来让工厂确定创造哪个类。
interface Product {
fun show();
}
class MyProduct : Product {
override fun show() {
println("my product")
}
}
class YourProduct : Product {
override fun show() {
println("your product")
}
}
class OtherProduct : Product {
override fun show() {
println("other product")
}
}
class ProductFactory {
fun create(name: String): Product {
return when (name) {
"my" -> {
MyProduct()
}
"your" -> {
YourProduct()
}
else -> {
OtherProduct()
}
}
}
}
fun main() {
val factory = ProductFactory()
val product = factory.create("my")
product.show()
}
那么如果之后需要继续增加或减少一个产品,那么就需要在 ProductFactory
中 when
语句进行更改。看似简单的操作,其实已经违反了我们的设计原则开闭原则,对修改应该是关闭的。所以说简单工厂模式是存在一定的缺陷的,并不能符合我们日后的扩展性。
针对上述问题,我们可以采取不同的方式去实现。来符合开闭原则。针对不同的 Product
采用不同的工厂方法。通过 Factory
接口来设定子类工厂。这样通过客户端的调用,便可以完成对象的产生。
interface Product {
fun show();
}
interface Factory {
fun create(): Product
}
class MyProduct : Product {
override fun show() {
println("my product")
}
}
class MyProductFactory : Factory {
override fun create(): Product {
val product = MyProduct()
// 其他初始化操作
return product
}
}
class YourProduct : Product {
override fun show() {
println("your product")
}
}
class YourProductFactory : Factory {
override fun create(): Product {
val product = YourProduct()
// 其他初始化操作
return product
}
}
class OtherProduct : Product {
override fun show() {
println("other product")
}
}
class OtherProductFactory : Factory {
override fun create(): Product {
val product = OtherProduct()
// 其他初始化操作
return product
}
}
fun main() {
val factory = YourProductFactory()
val product = factory.create()
product.show()
}
无论是参数化方法的工厂模式,还是通过单独的工厂去逐步生成,都做到了使用者和生产者的解耦。同时工厂模式还对开闭原则做了很好的完善。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK