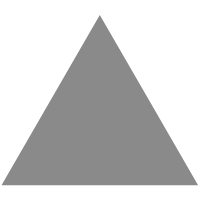
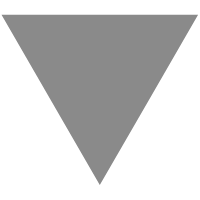
GitHub - googlemaps/android-maps-compose: Jetpack Compose components for the Map...
source link: https://github.com/googlemaps/android-maps-compose
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
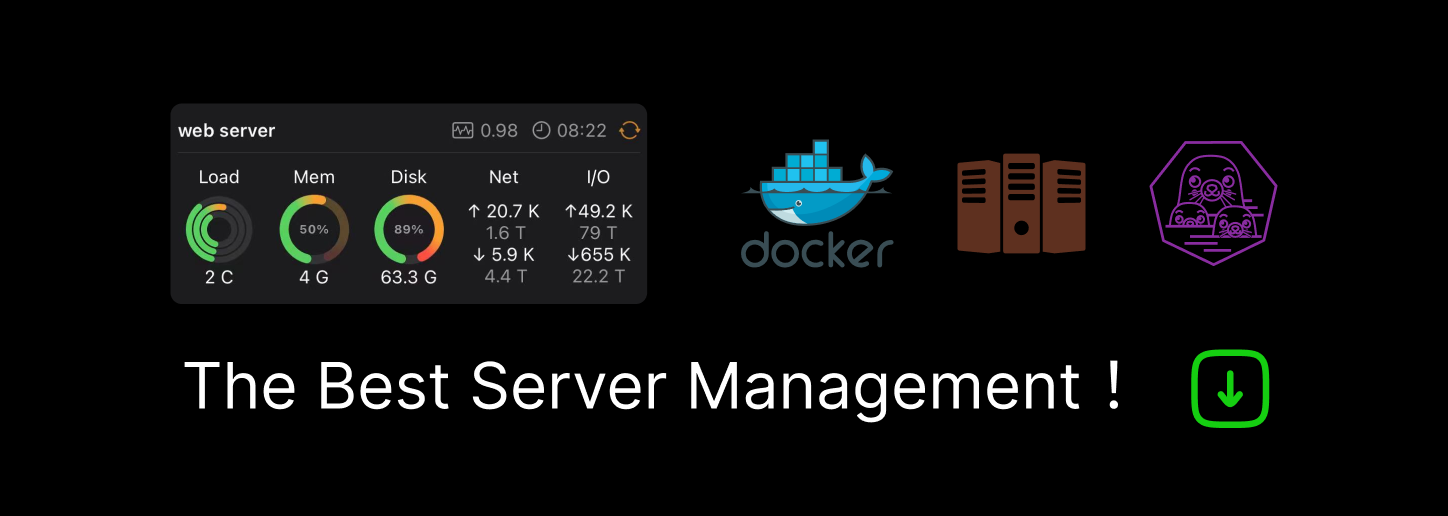
Maps Compose 
Description
This repository contains Jetpack Compose components for the Maps SDK for Android.
Requirements
- Kotlin-enabled project
- Jetpack Compose-enabled project
- An API key
- API level 21+
Usage
Adding a map to your app looks like the following:
val singapore = LatLng(1.35, 103.87) GoogleMap( modifier = Modifier.fillMaxSize(), googleMapOptionsFactory = { GoogleMapOptions().camera(CameraPosition.fromLatLngZoom(singapore, 10f)) } )
Creating and configuring a map
Configuring the map can be done either by passing a GoogleMapOptions
instance
to initialize the map, or by passing a MapProperties
object into the GoogleMap
composable.
// Initialize map by providing a googleMapOptionsFactory GoogleMap( googleMapOptionsFactory = { GoogleMapOptions().mapId("MyMapId") } ) // ...or set properties using MapProperties which you can use to recompose the map var mapProperties by remember { mutableStateOf( MapProperties(maxZoomPreference = 10f, minZoomPreference = 5f) ) } Box(Modifier.fillMaxSize()) { GoogleMap(mapProperties = mapProperties) Button(onClick = { mapProperties = mapProperties.copy( isBuildingEnabled = !mapProperties.isBuildingEnabled ) }) { Text(text = "Toggle isBuildingEnabled") } }
Controlling the map's camera
Camera changes and updates can be observed and controlled via CameraPositionState
.
val singapore = LatLng(1.35, 103.87) val cameraPositionState: CameraPositionState = rememberCameraPositionState { position = CameraPosition.fromLatLngZoom(singapore, 11f) } Box(Modifier.fillMaxSize()) { GoogleMap(cameraPositionState = cameraPositionState) Button(onClick = { // Move the camera to a new zoom level cameraPositionState.move(CameraUpdateFactory.zoomIn()) }) { Text(text = "Zoom In") } }
Drawing on a map
Drawing on the map, such as adding markers, can be accomplished by adding child
composable elements to the content of the GoogleMap
.
GoogleMap( //... ) { Marker(position = LatLng(-34, 151), title = "Marker in Sydney") Marker(position = LatLng(35.66, 139.6), title = "Marker in Tokyo") }
Sample App
This repository includes a sample app.
To run it, you'll have to:
- Get a Maps API key
- Add an entry in
local.properties
that looks likeMAPS_API_KEY=YOUR_KEY
- Build and run
Installation
dependencies { implementation 'com.google.maps.android:maps-compose:1.0.0' // Make sure to also include the latest version of the Maps SDK for Android implementation 'com.google.android.gms:play-services-maps:18.0.2' }
Documentation
You can learn more about all the extensions provided by this library by reading the reference documents.
Support
Encounter an issue while using this library?
If you find a bug or have a feature request, please file an issue. Or, if you'd like to contribute, send us a pull request and refer to our code of conduct.
You can also reach us on our Discord channel.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK