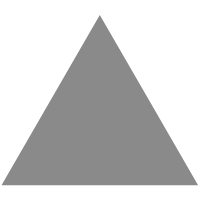
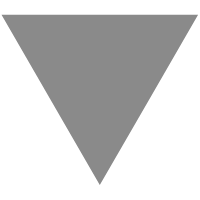
从零开发区块链应用(一)--golang配置文件管理工具viper
source link: https://learnblockchain.cn/article/3446
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
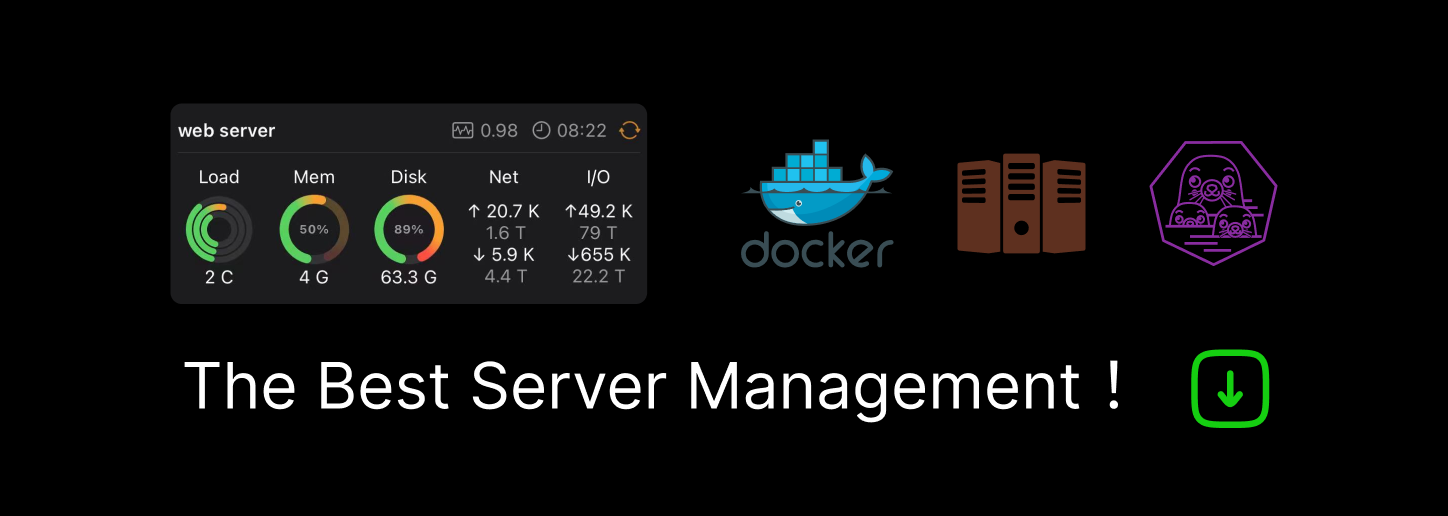
参考文件:https://github.com/spf13/viper
一、viper简介
viper是一个go 开发工具,主要是用于处理各种格式的配置文件,简化程序配置的读取问题,所以viper 是一个配置解决方案,它拥有丰富的特性:
- 支持 JSON/TOML/YAML/HCL/envfile/Java properties 等多种格式的配置文件;
- 可以设置监听配置文件的修改,修改时自动加载新的配置;
- 从环境变量、命令行选项和
io.Reader
中读取配置; - 从远程配置系统中读取和监听修改,如 etcd/Consul;
- 代码逻辑中显示设置键值。
二、viper 的基本方法
2.1 安装
go get github.com/spf13/viper
2.2 读取配置文件
Viper需要最少知道在哪里查找配置文件的配置。Viper支持JSON
、TOML
、YAML
、HCL
、envfile
和Java properties
格式的配置文件。Viper可以搜索多个路径,但目前单个Viper实例只支持单个配置文件。Viper不默认任何配置搜索路径,将默认决策留给应用程序。
下面是一个如何使用Viper搜索和读取配置文件的示例。不需要任何特定的路径,但是至少应该提供一个配置文件预期出现的路径。
viper.SetConfigFile("./config.yaml") // 指定配置文件路径
viper.SetConfigName("config") //配置文件名称(无扩展名)
viper.SetConfigType("yaml") // 如果配置文件的名称中没有扩展名,则必须要配置此项
viper.AddConfigPath("/etc/appname/") // 查找配置文件所在的路径
viper.AddConfigPath("$HOME/.appname") // 多次调用以添加多个搜索路径
viper.AddConfigPath(".") // 还可以在工作目录中查找配置
err := viper.ReadInConfig() // 查找并读取配置文件
if err != nil { // 处理读取配置文件的错误
panic(fmt.Errorf("Fatal error config file: %s \n", err))
}
在加载配置文件出错时,你可以像下面这样处理找不到配置文件的特定情况:
if err := viper.ReadInConfig(); err != nil {
if _, ok := err.(viper.ConfigFileNotFoundError); ok {
// 配置文件未找到错误;如果需要可以忽略
} else {
// 配置文件被找到,但产生了另外的错误
}
}
// 配置文件找到并成功解析
2.3 viper取值的函数
- Get(key string) : interface{} //自动判断类型
- GetBool(key string) : bool //获取bool类型
- GetFloat64(key string) : float64 //获取浮点
- GetInt(key string) : int //获取int类型
- GetString(key string) : string //获取string类型
- GetIntSlice(key string) : []int //获取slice类型(slice类型为int)
- GetStringSlice(key string) : []string //获取slice类型(slice类型为string)
- GetStringMap(key string) : map[string]interface{} // 获取map类型(map自动判断类型)
- GetStringMapString(key string) : map[string]string //获取map类型(map类型为string类型)
- GetTime(key string) : time.Time // 获取时间类型
- GetDuration(key string) : time.Duration //获取持续时间
- IsSet(key string) : bool //判断某个键值是否存在
- AllSettings() : map[string]interface{} // 获取所有配置
三、 viper使用举例
3.1 yaml配置文件
# 程序配置
console:
name: "token"
version: "v1.0"
port: ":9100"
# 日志配置
log: "yaml/log.json"
node:
bsc:
url: "https://data-seed-prebsc-1-s1.binance.org:8545/"
chainID: 97
heco:
url: "https://http-testnet.huobichain.com"
chainID: 256
mysql:
user: root
password: "123456"
host: 127.0.0.1
port: 3306
database: token
3.2 本地配置文件读取方式
- 将上述两个配置文件和下面的 main.go 放在统一目录之下,即可实现读取配置文件
package config
import (
"github.com/token/pkg/go-logger"
"github.com/spf13/viper"
)
var Conf *Config
// Initialize
func Initialize() {
// 加载配置文件
viper.SetConfigType("yaml")
viper.SetConfigName("config")
viper.AddConfigPath("./yaml/")
if err := viper.ReadInConfig(); err != nil {
logger.Fatal("ReadInConfig error:", err)
}
Conf = &Config{
LogCfg: viper.GetString("log"),
Console: &Console{
Name: viper.GetString("console.name"),
Version: viper.GetString("console.version"),
Port: viper.GetString("console.port"),
},
Mysql: &Mysql{
User: viper.GetString("mysql.user"),
Password: viper.GetString("mysql.password"),
Host: viper.GetString("mysql.host"),
Port: viper.GetString("mysql.port"),
Database: viper.GetString("mysql.database"),
},
Node: &Node{
BSC: &NodeUrl{
Url: viper.GetString("node.bsc.url"),
ChainID: viper.GetInt64("node.bsc.chainID"),
},
HECO: &NodeUrl{
Url: viper.GetString("node.heco.url"),
ChainID: viper.GetInt64("node.heco.chainID"),
},
},
}
// 初始化日志配置
logger.SetLogger("./yaml/log.json")
logger.Info("Successful configuration load")
}
3.3 将配置信息序列化为结构体对象
package config
// yaml配置结构体
type Config struct {
LogCfg string `json:"log"`
Console *Console `json:"console"`
Mysql *Mysql `json:"mysql"`
Node *Node `json:"node"`
}
// yaml配置结构体
type Console struct {
Name string `json:"name"`
Version string `json:"version"`
Port string `json:"port"`
}
type Mysql struct {
User string `json:"user"`
Password string `json:"password"`
Host string `json:"host"`
Port string `json:"port"`
Database string `json:"database"`
}
type Node struct {
BSC *NodeUrl `json:"bsc"`
HECO *NodeUrl `json:"heco"`
}
type NodeUrl struct {
Url string `json:"url"`
ChainID int64 `json:"chainID"`
}
3.4 配置读取
-
读取服务端口
config.Conf.Console.Port
-
读取BSC节点url
config.Conf.Node.BSC.Url
-
读取mysql主机IP
config.Conf.Mysql.Host
本系列文章:
从零开发区块链应用(一)--golang配置文件管理工具viper
从零开发区块链应用(二)--mysql安装及数据库表的安装创建
从零开发区块链应用(三)--mysql初始化及gorm框架使用
从零开发区块链应用(四)--自定义业务错误信息
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK