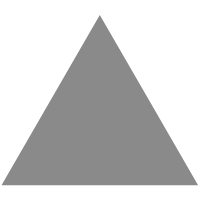
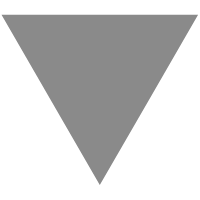
Testing a React App with Jest and react-testing-library
source link: https://hackernoon.com/testing-a-react-app-with-jest-and-react-testing-library
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
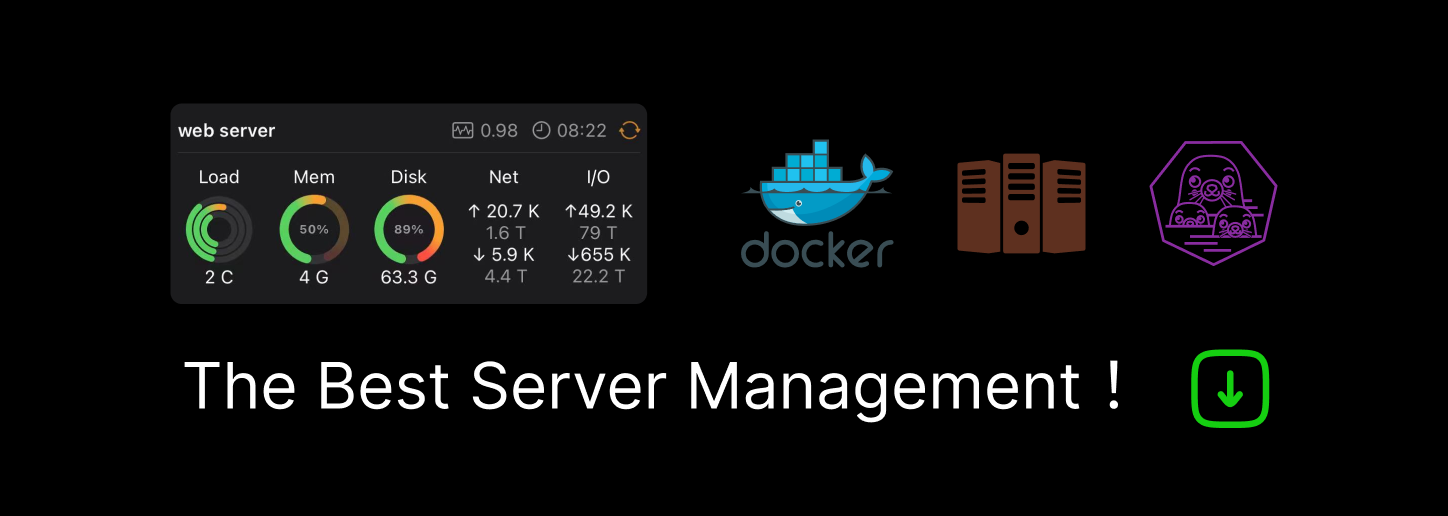
Prerequisites
An existing React app.
You can find the full source code @ GitHub: https://github.com/alexadam/project-templates/tree/master/projects/react-app-tests
Setup
Install Jest and react-testing-library
yarn add --dev jest @types/jest ts-jest @testing-library/react @testing-library/jest-dom
In the project's root folder, create a file named jest.config.js
and add:
module.exports = {
roots: ["./src"],
transform: {
"^.+\\.tsx?$": "ts-jest"
},
testEnvironment: 'jest-environment-jsdom',
setupFilesAfterEnv: [
"@testing-library/jest-dom/extend-expect"
],
testRegex: "^.+\\.(test|spec)\\.tsx?$",
moduleFileExtensions: ["ts", "tsx", "js", "jsx", "json", "node"]
};
Update package.json
by adding a new script entry:
"scripts": {
...
"test": "jest",
...
Create a Test
We'll test the basic React app created from scratch here: https://alexadam.dev/blog/create-react-project.html
To test the Numbers component, create a file named numbers.test.tsx
, in the src
folder:
import React, { Props } from "react";
import { fireEvent, render, screen } from "@testing-library/react";
import Numbers from "./numbers";
describe("Test <Numbers />", () => {
test("Should display 42", async () => {
render(<Numbers initValue={42} />);
const text = screen.getByText("Number is 42");
expect(text).toBeInTheDocument();
});
test("Should increment number", async () => {
const { container } = render(<Numbers initValue={42} />)
const incButton = screen.getByText('+')
fireEvent(
incButton,
new MouseEvent('click', {
bubbles: true,
cancelable: true,
}),
)
const text = screen.getByText("Number is 43");
expect(text).toBeInTheDocument();
});
test("Should decrement number", async () => {
const { container } = render(<Numbers initValue={42} />)
const decButton = screen.getByText('-')
fireEvent.click(decButton)
const text = screen.getByText("Number is 41");
expect(text).toBeInTheDocument();
});
});
Run the tests:
yarn test
See the results:
$> yarn test
yarn run v1.22.17
$ jest
PASS src/numbers.test.tsx
Test <Numbers />
✓ Should display 42 (29 ms)
✓ Should increment number (14 ms)
✓ Should decrement number (8 ms)
Test Suites: 1 passed, 1 total
Tests: 3 passed, 3 total
Snapshots: 0 total
Time: 2.893 s
Ran all test suites.
✨ Done in 3.88s.
First published here
Recommend
-
6
React Component Testing Guide: Jest and RTLMarch 14th 2021 5
-
8
React is an open-source framework for building reusable UI components and apps. It is used by thousands of developers around the world to create complex and multifaceted a...
-
7
Testing React app with JestIn this post we will look into how to write tests for react application using Jest Jest is open source testing framework built on top of JavaScript. It was majorly designed t...
-
17
Integrate Jest & React Testing Library in a React Vite Project. Integrate Jest & React Testing Library in a React Vite Project Install Dependencies...
-
2
Testing React Tracking with Jest and Enzyme By Matt Dole Apr 15, 2021 Recently, I needed to test a button that would make an analyt...
-
4
Not FoundYou just hit a route that doesn't exist... the sadness.LoginRadius empowers businesses to deliver a delightful customer experience and win customer trust. Using the LoginRadius Identity...
-
7
Testing React Native apps with JestHow to write unit and e2e tests for React Native apps using Jest in an Nx workspaceIn my previous
-
5
// Tutorial //How to Test a React App with Jest and React Testing LibraryPublished on May 9, 2022By Alyssa Holland
-
14
Instalación y configuracion de Jest + React Testing Library En proyectos de React + Vite Instalaciones: yarn add --dev jest babel-jest @babel/preset-env @babel/preset-react yarn add --dev @te...
-
4
Introduction React-Query is a powerful tool for fetching/caching the data on the frontend side, yet testing React-Query with Jest and React-testing-library might be a bit tricky, in this article we’re going to demonstrate it and see ho...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK