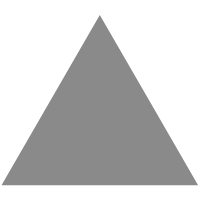
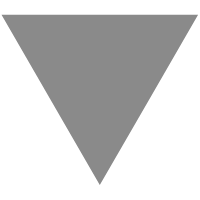
Setup Eslint, Prettier with Typescript and React
source link: https://dev.to/thatanjan/setup-eslint-prettier-with-typescript-and-react-lnj
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
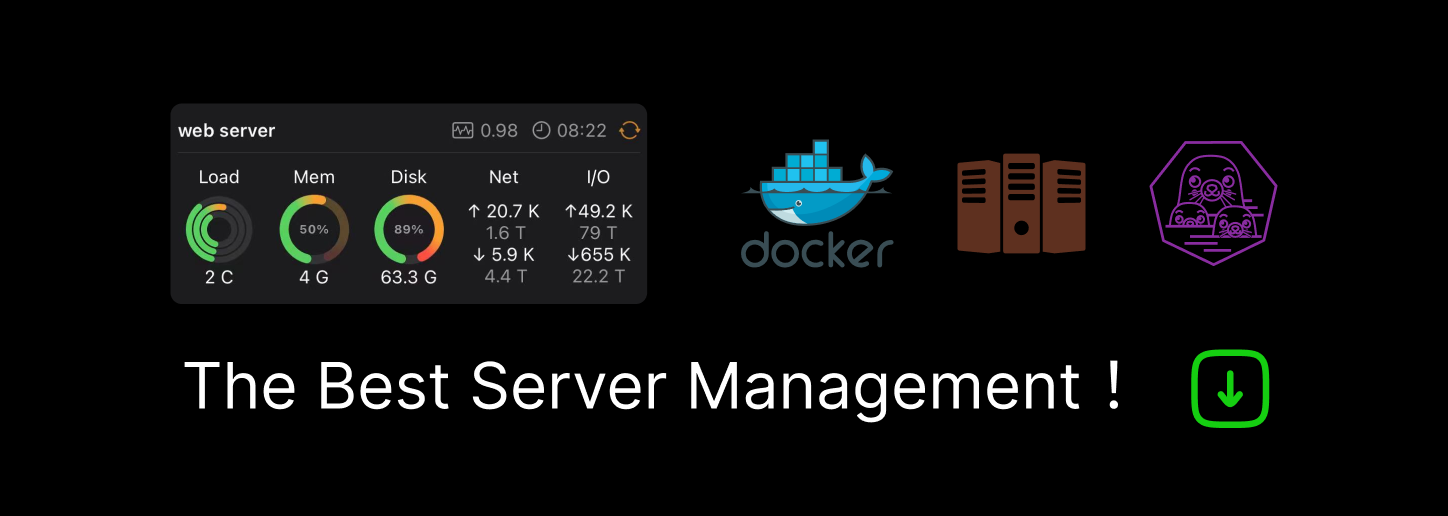
In this blog, I will teach you, how to set up eslint, prettier with TypeScript and React. Even if you are not using TypeScript or react you can still follow along.
In case if you don't know what eslint and prettier is:
Eslint is a linter which enforces developer to write good and consistent code all over Project. Prettier is a good formatter tool that automatically formats the source code.
Video tutorial
I have already created a video about it on my youtube channel. Check that out for more details.
If you like this video, please like share, and Subscribe to my channel.
For react, I will use Nextjs. Again the principles are the same. You can also use it with create-react-app.
Editor setup
You need to install eslint and prettier plugins for your editor. To learn more, visit these links.
Eslint
Prettier
Setup
yarn create-next-app
Enter fullscreen mode
Exit fullscreen mode
Then put your app name. I am going to call it eslint-prettier-typescript-react
After that, it will set everything for you.
Now change the directory to the folder.
cd eslint-prettier-typescript-react
Enter fullscreen mode
Exit fullscreen mode
TypeScript setup for Nextjs (optional)
Create a tsconfig.json
file.
touch tsconfig.json
Enter fullscreen mode
Exit fullscreen mode
Install typescript packages.
yarn add --dev typescript @types/react
Enter fullscreen mode
Exit fullscreen mode
Then start the server.
yarn dev
Enter fullscreen mode
Exit fullscreen mode
It will fill up the tsconfig.json
file. Now convert all the javascript files to typescript files.
By the way, this blog is originally published on cules coding website.. I would be glad if you give it a visit.
Setup Absolute import
Absolute Import vs Relative Import
Inside the tsconfig.json
file. create a new property baseUrl
and set the import point. I will create an src
folder and put all source code inside that.
So add this extra code:
{
"baseUrl": "src/"
"include": ["next-env.d.ts", "**/*.ts", "**/*.tsx", "src/"],
}
Enter fullscreen mode
Exit fullscreen mode
Setup eslint
Since Nextjs version 11.0.0, eslint is already configured out of the box. But that's not enough for me. I want more. So, I will customize that more.
- Install eslint globally on your computer.
yarn global add eslint
Enter fullscreen mode
Exit fullscreen mode
- Inside your project initialize eslint.
eslint --init
Enter fullscreen mode
Exit fullscreen mode
- Choose 3.
- Choose 1
- Choose your framework if you are using it. In my case
react
- If you are using TypeScript then
yes
. I am using TypeScript.
- Browser in my case.
- Use a popular style guide.
- I would like to use the Airbnb style guide. You can choose any style guide. But Airbnb is good and I recommend it.
- I will use json for my config file.
- It will ask you to install some packages to Install with
npm
. If you want to usenpm
then go ahead. But I will use yarn.
So For those who are using yarn like me, You can copy and paste package names and install them.
With typescript:
yarn add --dev eslint-plugin-react @typescript-eslint/eslint-plugin@latest eslint-config-airbnb@latest eslint eslint-plugin-import eslint-plugin-jsx-a11y eslint-plugin-react-hooks @typescript-eslint/parser@latest
Enter fullscreen mode
Exit fullscreen mode
Without typescript:
yarn add --dev eslint-plugin-react eslint-config-airbnb@latest eslint eslint-plugin-import eslint-plugin-jsx-a11y eslint-plugin-react-hooks
Enter fullscreen mode
Exit fullscreen mode
It will create an eslint config file .eslintrc
. It will be a hidden file.
Prettier Setup
- Let's install prettier.
yarn add --dev eslint-config-prettier prettier
Enter fullscreen mode
Exit fullscreen mode
- Create a prettier config file.
touch .prettierrc.json
Enter fullscreen mode
Exit fullscreen mode
Now you can put your config in the .prettierrc.json
file in json format. You can find the options from here
My basic config for prettier.
{
"singleQuote": true,
"useTabs": true,
"tabWidth": 1,
"semi": false,
"jsxSingleQuote": true,
"arrowParens": "avoid"
}
Enter fullscreen mode
Exit fullscreen mode
Now we are done with prettier. Let's set up eslint config.
ESlint config setup
Your .eslintrc
file should look like this.
{
"env": {
"browser": true,
"es2021": true
},
"extends": ["plugin:react/recommended", "airbnb"],
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaFeatures": {
"jsx": true
},
"ecmaVersion": 12,
"sourceType": "module"
},
"plugins": ["react", "@typescript-eslint"],
"rules": {}
}
Enter fullscreen mode
Exit fullscreen mode
We need to extend the eslint config with prettier and nextjs. So add prettier
and next/core-web-vitals
to extends
array.
{
"extends": [
"next/core-web-vitals",
"plugin:react/recommended",
"airbnb",
"prettier"
]
}
Enter fullscreen mode
Exit fullscreen mode
Explanation:
- Extends array takes configs. But configs need to be ordered. The last items will have more priority than previous configs. For example, if any rule gets conflict between
airbnb
andprettier
, the Airbnb config rule will be overridden by prettier.
Run eslint from the command line
For nextjs(11.0.0 +):
{
"scripts": {
"lint": "next lint"
}
}
Enter fullscreen mode
Exit fullscreen mode
For every other case:
{
"scripts": {
"lint": "eslint . --ext ts --ext tsx --ext js"
}
}
Enter fullscreen mode
Exit fullscreen mode
Let's fix some eslint errors
To turn any rule off
or on
, add the rules to the rules array. You can find the docs from here. Please watch my video to understand it well.
- allow jsx on other extensions.
{
"rules": {
"react/jsx-filename-extension": [
1,
{ "extensions": [".js", ".jsx", ".ts", ".tsx"] }
]
}
}
Enter fullscreen mode
Exit fullscreen mode
- File extension on import statement.
{
"rules": {
"import/extensions": [
"error",
"always",
{
"js": "never",
"jsx": "never",
"ts": "never",
"tsx": "never"
}
]
}
}
Enter fullscreen mode
Exit fullscreen mode
- Import unresolved error for absolute import(if you are using).
{
"settings": {
"import/resolver": {
"node": {
"extensions": [".js", ".jsx", ".ts", ".tsx"],
"moduleDirectory": ["node_modules", "src/"]
}
}
}
}
Enter fullscreen mode
Exit fullscreen mode
You can find my Eslint config from here
So, that's it for today. I hope I have covered everything that you need to know about how to set up.
Shameless Plug
I have made few project based videos with vanilla HTML, CSS, and JavaScript.
You will learn about:
- Javascript intersection observer to add cool effects
- DOM manipulation
- Aligning elements with CSS positions.
- How to make responsive websites.
- How to create slide based webpage.
These will be great projects to brush up on your front end skills.
If you are interested you can check the videos.
You can also demo the application from here:
Please like and subscribe to Cules Coding. It motivates me to create more content like this.
That's it for this blog. I have tried to explain things simply. If you get stuck, you can ask me questions.
By the way, I am looking for a new opportunity in a company where I can provide great value with my skills. If you are a recruiter, looking for someone skilled in full stack web development and passionate about revolutionizing the world, feel free to contact me. Also, I am open to talking about any freelance project.
About me
Why do I do what I do?
The Internet has revolutionized our life. I want to make the internet more beautiful and useful.
What do I do?
I ended up being a full-stack software engineer.
What can I do?
I can develop complex full-stack web applications like social media applications or e-commerce sites. See more of my work from here
What have I done?
I have developed a social media application called Confession. The goal of this application is to help people overcome their imposter syndrome by sharing our failure stories.
Screenshot
I also love to share my knowledge. So, I run a youtube channel called Cules Coding where I teach people full-stack web development, data structure algorithms, and many more. So, Subscribe to Cules Coding so that you don't miss the cool stuff.
Want to work with me?
I am looking for a team where I can show my ambition and passion and produce great value for them.
Contact me through my email or any social media as @thatanjan. I would be happy to have a touch with you.
Contacts
- Email: [email protected]
- linkedin: @thatanjan
- portfolio: anjan
- Github: @thatanjan
- Instagram (personal): @thatanjan
- Instagram (youtube channel): @thatanjan
- twitter: @thatanjan
Blogs you might want to read:
Videos might you might want to watch:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK