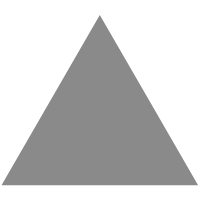
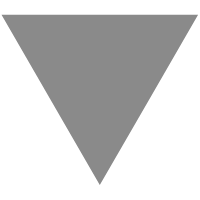
Python Keras | Transformation to multilabel classification
source link: https://dev.to/f1bot/python-keras-transformation-to-multilabel-classification-36go
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
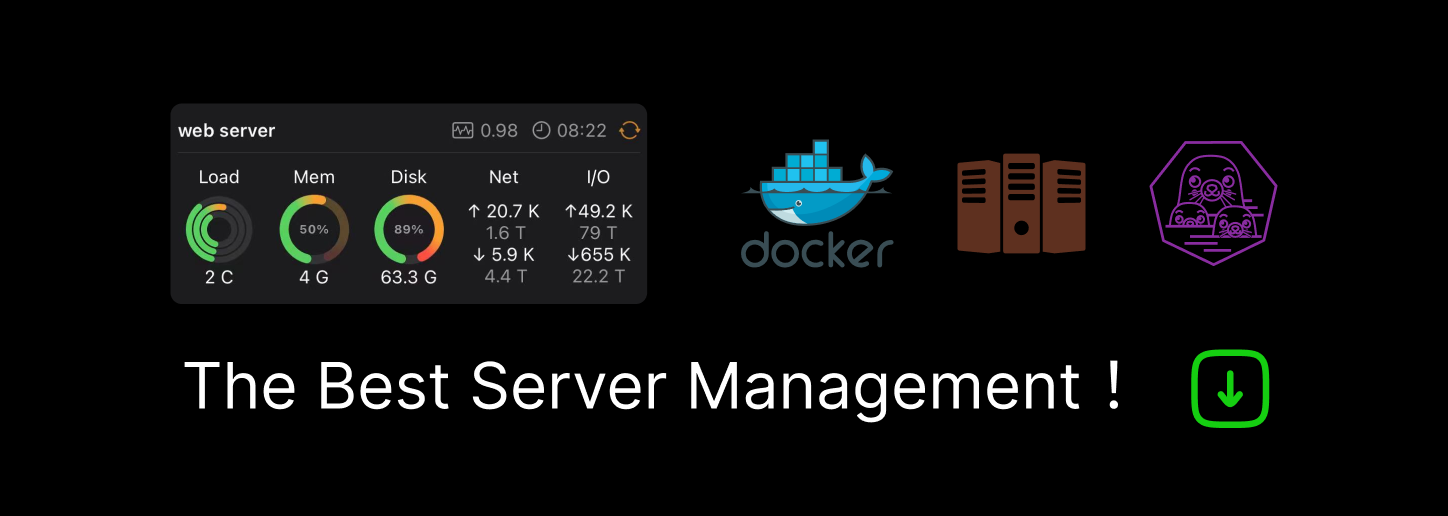

Posted on Dec 19
Python Keras | Transformation to multilabel classification
Hi. I'm trying to implement a neural network in Python (Keras) that will predict the probability of multiple outcomes. There is no need to delve into the essence of the classes themselves, so I reduced the code to 3 input and 3 outgoing, in fact there are more of them.
At the moment I have the following code:
import keras as k
import pandas as pd
import numpy as np
import matplotlib.pyplot as pltdata_frame = pd.read_csv("123.csv")
input_names = ["Sex", "Age", "IQ"]
output_names = ["OUTPUT1", "OUTPUT2", "OUTPUT3"]raw_input_data = data_frame[input_names]
raw_output_data = data_frame[output_names]max_age = 100
encoders = {"Age": lambda age: [age/max_age],
"Sex": lambda gen: {"male": [0], "female": [1]}.get(gen),
"IQ": lambda iq_value: [iq_value],
"OUTPUT1": lambda output1_value: [output1_value],
"OUTPUT2": lambda output2_value: [output2_value],
"OUTPUT3": lambda output3_value: [output3_value]}def dataframe_to_dict(df):
result = dict()
for column in df.columns:
values = data_frame[column].values
result[column] = values
return resultdef make_supervised(df):
raw_input_data = data_frame[input_names]
raw_output_data = data_frame[output_names]
return {"inputs": dataframe_to_dict(raw_input_data),
"outputs": dataframe_to_dict(raw_output_data)}def encode(data):
vectors = []
for data_name, data_values in data.items():
encoded = list(map(encoders[data_name], data_values))
vectors.append(encoded)
formatted = []
for vector_raw in list(zip(*vectors)):
vector = []
for element in vector_raw:
for e in element:
vector.append(e)
formatted.append(vector)
return formattedsupervised = make_supervised(data_frame)
encoded_inputs = np.array(encode(supervised["inputs"]))
encoded_outputs = np.array(encode(supervised["outputs"]))train_x = encoded_inputs[:300]
train_y = encoded_outputs[:300]test_x = encoded_inputs[300:]
test_y = encoded_outputs[300:]model = k.Sequential()
model.add(k.layers.Dense(units=5, activation="relu"))
model.add(k.layers.Dense(units=1, activation="sigmoid"))
model.compile(loss="mse", optimizer="sgd", metrics=["accuracy"])fit_results = model.fit(x=train_x, y=train_y, epochs=100, validation_split=0.2)
plt.title("Losses train/validation")
plt.plot(fit_results.history["loss"], label="Train")
plt.plot(fit_results.history["val_loss"], label="Validation")
plt.legend()
plt.show()plt.title("Accuracies train/validation")
plt.plot(fit_results.history["accuracy"], label="Train")
plt.plot(fit_results.history["val_accuracy"], label="Validation")
plt.legend()
plt.show()predicted_test = model.predict(test_x)
real_data = data_frame.iloc[300:][input_names+output_names]
real_data["POUTPUT1", "POUTPUT2", "POUTPUT3"] = predicted_test
print(real_data)
real_data.to_csv('C:/***/133.csv')
Help implement the output of probabilities for all 3 outcomes [POUTPUT1, POUTPUT2, POUTPUT3] to the console (currently outputs only 1 predicate). Saving to a new table should also save 3 new predicates.
Visually, I'm counting on this representation of the result
I will be very grateful for your help.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK