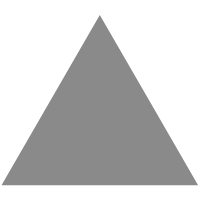
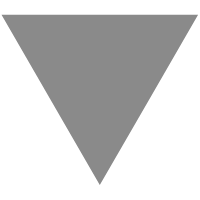
How to Detect Browser Window is Active or not
source link: https://www.laravelcode.com/post/how-to-detect-browser-window-is-active-or-not
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
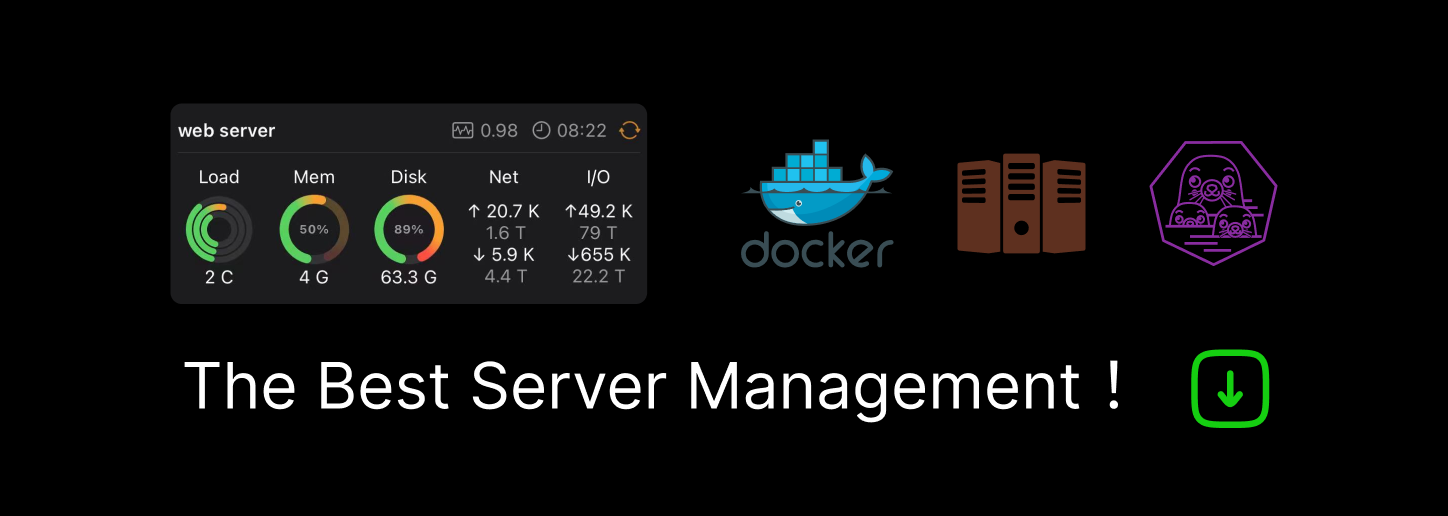
How to Detect Browser Window is Active or not
Javascript is great programming language to learn. You can do many funny things with Javascript. In the web browser, you can dynamically change in the HTML dom elements.
In this article, we will go through on how to check if user is active or inactive. For that we will check if is there any browser event inserted like mousemove, click event or scroll event.
There are many usage where user activity is required to check. Mostly this is useful to logout user after inactive or popup modal to confirm user.
Using Javascript
Bellow is the function on how to check user if user is active or inactive using Javascript. On these events trigger, resetTime() function will trigger and time will be cleared. So if none of these event happen, then alertUser() function will be trigger.
You can set any action in alertUser() method, that you want to do.
<script type="text/javascript">
function inactivityTime() {
var time;
// events
window.onload = resetTime;
window.onclick = resetTime;
window.onkeypress = resetTime;
window.ontouchstart = resetTime;
window.onmousemove = resetTime;
window.onmousedown = resetTime;
window.addEventListener('scroll', resetTime, true);
function alertUser() {
// do your task here
alert("User is inactive.");
}
function resetTime() {
clearTimeout(time);
time = setTimeout(alertUser, 1000 * 10); // 10 seconds
}
};
// run the function
inactivityTime();
</script>
Using JQuery
Same thing you can do with JQuery. Bellow code is how you can do this with JQuery.
<script src="https://code.jquery.com/jquery-3.5.0.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
var inactiveTime;
$('*').bind('mousemove click mouseup mousedown keydown keypress keyup submit change mouseenter scroll resize dblclick', function () {
function alertUser() {
// do your task here
alert("User is inactive.");
}
clearTimeout(inactiveTime);
inactiveTime = setTimeout(alertUser, 1000 * 10); // 10 seconds
});
$("body").trigger("mousemove");
});
</script>
After the defined time, if there is no event trigger, then alertUser() function calls, you can do your task in this function.
I hope you liked this article,

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK