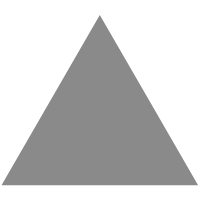
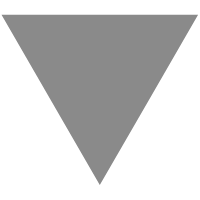
Common Python List Methods With Syntax And Examples
source link: https://dev.to/codingvidya/common-python-list-methods-with-syntax-and-examples-bfp
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
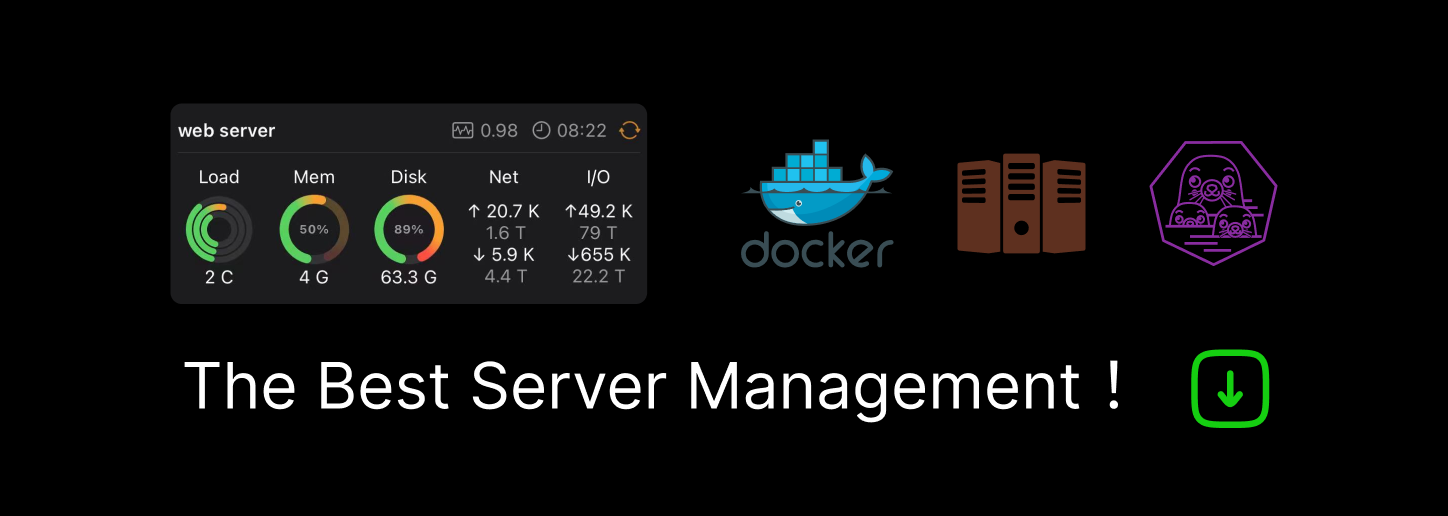
Posted on Dec 17
Common Python List Methods With Syntax And Examples
Python lists are not just used to store data. There are powerful data structures if used correctly. However, we can’t use them effectively if we don’t know about their methods.
Though Python has some built-in functions that can operate on lists, Python lists have methods that can be used to operate on its list object both in-place and out-of-place.
Common Python List Methods
Before we delve into the common List Methods, it’s important to understand a few characteristics of a Python list.
A Python list is a collection of zero to infinite(as many as your computer can allow) data types(numbers, Strings, booleans, tuples, lists, etc) surrounded by square brackets with each item separated by a comma (,).
A Python list is an ordered, mutable sequence and uses the zero-based numbering system i.e. all its items are uniquely identified by an index number that begins from 0 and ends at n-1, where n is the list’s length. Learn more from best python books
With that in mind, we can confidently delve into looking at Python list methods. It is important to know that Python List has many methods and Python has many built-in methods to work on list data for various purposes.
Method For Addition
Before starting to perform an operation on a list, we may first want to add data to it. Python list has the following common methods to add items into its list.
#1) list.append(x)
The append() method takes an item as an argument and adds it at the end of the list.
As seen above, Python lists are ordered. So, the position of each item in a list is important. If we are adding an item to a list and we want it to be the last item in that list, then append() is what we need.
Example 1: In the below code, our list holds a name(String) as the first item and age(number) as the second item. We want to add a third item salary(number) to the list such that we have
list(name, age, salary)
Below is a walkthrough of a Python shell.
name = "Enow Kevin" # define a name
age = 30 # define an age
details = [name, age] # create a list with name and age
details
['Enow Kevin', 30]
salary = 165000 # define a salary
details.append(salary) # add the salary to the end of the list
details
['Enow Kevin', 30, 165000]list.append(x) is similar to a[len(a):] = [x] .
Consider the example below:
Let’s add a fourth item gender(String) to our details list defined above.
gender = 'Male' # define a gender
details[len(details):] = [gender] # add gender to the end of the list
details
['Enow Kevin', 30, 165000, 'Male']Note: In the above example, we used len() which is a Python built-in function that can operate on a list. Learn more from best python programming books
2) list.extend(iterable)
extend(iterable) takes in an iterable as an argument, and append each item in that iterable into the list.
This method uses append() under the hood. This method is great if we have a collection of items that we want to append each item into another list.
Example 2: Let’s say we have two student’s academic grades gradeScience and gradeArt and we decide to perform a common operation on the grades. One way will be to join them together first.
Given below is a walkthrough of a Python shell.
gradeScience = [4,3,9,10] # grades in science
gradeArt = [9,11,7,5] # grades in art
gradeArt
[9, 11, 7, 5]
gradeArt.extend(gradeScience) # join grades
gradeArt # we can now perform the operation on the joined grades
[9, 11, 7, 5, 4, 3, 9, 10]
The same thing can be achieved with “append() in a loop”. Given that the extend() method takes in an iterable and adds each item in that iterable into the list, we can use a for loop to demonstrate how that works.
def extend_loop(extender, extended):
"""for each item in the extender list, append the item
into the extended list"""
for item in extender:
extended.append(item)
if name == "main":
gradeScience = [4,3,9,10] # grades in science
gradeArt = [9,11,7,5] # grades in art
print("gradeArt: {} before extended".format(gradeArt))
# join gradeScience and gradeArt into gradeArt
extend_loop(gradeScience, gradeArt)
print("gradeArt: {} after extended".format(gradeArt))
Enter fullscreen mode
Exit fullscreen mode
Output
append(x)
It is important to know the difference between append(x) and extend(iterable).
Difference between the Python list methods: append and iterable.
append(x) extend(iterable)
1 Takes in one argument and could be iterable Takes in one argument and should be iterable
2 Adds an item into a list Adds each item of the iterable into a list.
Example 3: To clearly see the difference between append and extend, let’s consider this example. We notice that both listApp and listExt take in an iterable as an argument, but what changes is the output.
listApp = [] # empty list
listExt = [] # empty list
listApp.append([1,2,3]) # append an iterable
listExt.extend([1,2,3]) # extend an iterable
listApp # check output
[[1, 2, 3]]
listExt # check output
[1, 2, 3]
Python method append() took the iterable, considered it as an item, and added it to the end of the list while Python method extend() took the iterable and added each item of that iterable into the list.Learn advanced programming skills with best book to learn python
#3) list.insert(i,x)
The Python list method insert() takes in two arguments(position, item) and inserts the item at the given position in the list.
The method insert() is useful when we want to add items into a list and have control over where precisely we want the items to be added to the list. Unlike append() and extend() that add items at the end of a list, insert() allows us to add items in whatever position we want.
Example 4: Say we have a list of names in order of excellence and we later find out that we left out a name and the name is supposed to be added to the list before a given name. In this case, both append() and extend() won’t help as we want to add the item in a given position.
The solution will be to get the index position of the name before which we want to add our new name and use insert() to add it at that position.
names = ['kevin','john','petter','tony','luke','andrew']# list of names
new_name = 'enow' # new name to be insert into the list of names
given_name = 'tony' # name before which to insert our new name
idx_given_name = names.index(given_name) # get index of given name
idx_given_name
3
names.insert(idx_given_name, new_name) # insert new name
names
['kevin', 'john', 'petter', 'enow', 'tony', 'luke', 'andrew']
Note: In the above example, we used the Python list method index() to get the index position of an item from a list(more on this later).
The concept of insert(index, x) is very important to understand. The first argument is the index position of the element before which to insert.
Which means that list.insert(0,x) will insert x in front of the item at index 0, and list.insert(len(list),x) will insert x at the end of the list(similar to list.append(x)) before the position at index len(list)[this index doesn’t exist for any list]
Method For Subtraction
In Python, as much as we can add items into a list, Python lists also permit us to remove or delete items from lists.
1) list.pop( [i] )
The pop() method removes and returns an item from a list. This method can take an optional index value as an argument(represented by the square bracket around i in the method’s signature which denotes “optional” and not a list), which denotes the index position from where to remove an item.
If none is given, then it defaults to -1 i.e. the last item of the list will be removed and returned.
Example 5: Given the list of cars below, we can decide to remove items from this list at any index position we wish to, thanks to pop()
cars = ['Toyota','Audi','BMW','Bugatti','Bently','Aston Martin'] #cars
cars
['Toyota', 'Audi', 'BMW', 'Bugatti', 'Bently', 'Aston Martin']
toyota = cars.pop(0) # remove and return car at index 0
cars
['Audi', 'BMW', 'Bugatti', 'Bently', 'Aston Martin']
toyota
'Toyota'
bugatti = cars.pop(2) # remove and return car at index 2
cars
['Audi', 'BMW', 'Bently', 'Aston Martin']
bugatti
'Bugatti'
aston_martin = cars.pop() # remove and return the last car in the list(at index -1)
cars
['Audi', 'BMW', 'Bently']
aston_martin
'Aston Martin'2) list.remove(x)
The Python list method remove(x) removes the first occurrence of an item that is equal to x.
The method remove() is useful when we want to remove an item from a list but we don’t care about the item and we don’t know where exactly it is positioned in the list.
Example 6: We have a list of books and we want to remove a specific book by name from the list as we don’t need it anymore.
books = ['python for beginners','python in a nutshell','python in action'] # list of books
books
['python for beginners', 'python in a nutshell', 'python in action']
book_to_remove = 'python in a nutshell' # book to be removed
books.remove(book_to_remove) # remove book
books
['python for beginners', 'python in action']
Note: If an item is given to remove() as an argument but it doesn’t exist in the list, a ValueError is raised. So, this method should always be called inside a try-except to handle the exception.
We can see some few differences between the methods pop() and remove() as shown below:
pop() remove()
1 Accepts an optional argument and should be an index position of the item to be removed. If index out of range, an IndexError is raised Accepts an argument and should be the item to be removed. If item not in list, a ValueError is raised
2 Removes the item from the list and returns it. Removes the item from the list but doesn’t return it.
3) list.clear()
Python list method clear() removes all items from the list, thereby making it empty. It takes no arguments.
This method is useful for re-assigning the list object with new values.
Example 7: We have a list that holds a collection of names, but we want to replace the names with new names. In this case, we first clear the previous names by making the list object empty, then add our new names with one of the different Python list methods for an addition that we saw above.
names = ['enow','kevin','eyong'] # our collection of names
names.clear() # clear the list object
names
[]
names.extend(['ayamba','derrick','fred']) # add new names with the extend() method
names
['ayamba', 'derrick', 'fred']
Note: We can achieve the same thing with del list[:].
Many may arguably say that instead of using clear(), why not we just re-assign the list with an empty list like this
a = [4,5,3] # list object containing numbers
a= [] # re-assign to an empty list
a
[]
Well here is the problem with doing this. If you have two variables(a and b) that reference a list object([4,5,3]), calling clear() on either of the variables(say a) will remove items from that referenced list object.
However, if you re-assign either of the variables(say a) with an empty list, then the one that was re-assigned(a) will no longer reference the same list object as b.
a = [4,5,3] # define a list object with numbers
b = a # assign 'a' to a new variable 'b'
b
[4, 5, 3]
a = [] # re-assign 'a' to an empty list. This changes its referenced object
a
[]
b # 'b' doesn't change because it doesn't reference the same list object as 'a'
[4, 5, 3]
Methods For Analyzing
Most of the time, we want to know and understand items in our list object. Python list has a few methods that we can use to understand its items.
Learn
1) list.index[x[,start[,end]])
The Python list method index(x) returns a zero-based index in the list of the first item whose value is equal to x. It takes one mandatory and two optional arguments. The first argument(x) represents the item we wish to find its index.
The last two arguments(start, end) determine a start and end(exclusive) index from which to search. Unlike other programming languages like JavaScript where its equivalent method indexOf() returns -1 when there is no such item, the Python list method index() raises a ValueError instead.
Learn more programming skills with python books for beginners
Example 8: Reference Example 4 where we used index() to get the index position of a name in a list of names. In this example 8, we will see how to use the optional arguments to limit the search to a particular subsequence of the list.
fruits = ['Apples','Avocados','Eggfruit','Apples','Cantaloupe','Cucumbers'] # list of fruits
fruits.index('Apples',1,3) # find index of 'Apples' from index 1 to index 3(exclusive)
Traceback (most recent call last):
File "", line 1, in
ValueError: 'Apples' is not in list
fruits.index('Apples',1,4) # find index of 'Apples' from index 1 to index 4(exclusive)
3
fruits.index('Cucumbers',3) # find index of 'Cucumbers' from index 3 to end of list.
52) list.count(x)
The Python list method count(x) is used to determine how many times a given item x appears in a list.
Unlike sets(), Python lists can hold duplicate items, if it interests us to know about these duplicates and determine how many times an item is duplicated, then count() is the way to go.
Example 9: Say we have a list object that holds the names of senators based on their votes. The more a senate is voted, the more its name appears in the list object. Now, we want to find out how many votes a particular senate has.
senate_votes = ['Tammy','John','Tammy','Tammy','Thomas','Maria','Maria'] # list of senate names based on their votes
senate_votes
['Tammy', 'John', 'Tammy', 'Tammy', 'Thomas', 'Maria', 'Maria']
tammy_votes = senate_votes.count('Tammy') # get tammy's vote count
tammy_votes
3
thomas_votes = senate_votes.count('Thomas') # get thomas's vote count
thomas_votes
1
mike_votes = senate_votes.count('Mike') # get mike's vote count
mike_votes
0
Note: The Python list method count() returns zero(0) if the item doesn’t appear in the list.
3) list.sort(key=None, reverse=False)
The Python list method sort() sorts list data in place. It takes in two keyword arguments.
key(defaults to None): It specifies a function of one argument used to extract items from the list and prepares them for comparison. For example, if we have a list of positive numbers, but we want our sort function to sort their negative values, we can use this key to achieve this.
reverse(defaults to False): It is a boolean value and if set to True, it will sort in descending order. Its value is False by default hence it sorts in ascending order.
Example 10: We have a list of numbers and we want to know the largest number in that list.
numbers = [4,34,6,1,0, 9,33,82,2] # list of numbers
numbers
[4, 34, 6, 1, 0, 9, 33, 82, 2]
sort_numb = numbers.copy() # make a copy to work with
sort_numb.sort(reverse=True) # sort in descending order
sort_numb
[82, 34, 33, 9, 6, 4, 2, 1, 0]
sort_numb[0] # get the first item which is the largest number
82
Note: We used the Python list method copy() to make a shallow copy of our list (More on this later).
Example 11: To demonstrate the use of the key, let’s sort the negative value of the items in the list.
numbers # list of num
[4, 34, 6, 1, 0, 9, 33, 82, 2]
sort_neg_numb = numbers.copy() # make a copy to work with
sort_neg_numb
[4, 34, 6, 1, 0, 9, 33, 82, 2]
sort_neg_numb.sort(key=lambda x: -x, reverse=True) # sort in descending order the negative value of each item.
sort_neg_numb
[0, 1, 2, 4, 6, 9, 33, 34, 82]From the output above, we see that though we sorted in descending order, the output is in ascending order. This is because our lambda function basically made it such that the comparison was made on the negative values of each item in the list.
Note: Since the key specifies a function of one argument, we used a lambda expression to define an anonymous function. All it does is, it returns the negative value of each item in the list.
Other Methods
In this section, we will look at some Python list methods that may not fall in any of the categories described above.
Learn more programming skills with best python book for beginners
1) list.copy()
The Python method copy() returns a shallow copy of the list. Shallow means it makes a copy of only the first level of a list(more on this later).
This method is useful for keeping the original list values before modifying the list. As we saw in Example 7 above, simply assigning a variable that references a list object to another variable doesn’t create a new object. Rather, the two variables will reference the same object.
Hence, changing one will cause the other to change.
Example 12: Reference examples 10 and 11 to see how this method was used.
Note: It is interesting to know that, the same can be achieved with slicing(a[:])
Example 13: To better understand the meaning of shallow in the description of copy(), consider the example below.
list1 = [1,2,3] # object list 1
list2 = [0,list1] # object list 2 containing reference to list 1
list2
[0, [1, 2, 3]]
list2_copy = list2.copy() # make a shallow copy of list 2
list2_copy
[0, [1, 2, 3]]
list2[0] = -1 # modify list 2's first level item
list2
[-1, [1, 2, 3]]
list2_copy # list 2's copy doesn't change
[0, [1, 2, 3]]
list1[0] = 'a' # modify list 1
list1
['a', 2, 3]
list2 # list 2 changes since it has reference to list 1
[-1, ['a', 2, 3]]
list2_copy # list 2's copy changes because the nested list ‘list 1’ wasn't copied.
[0, ['a', 2, 3]]The above example demonstrates that shallow copying means any nested iterable in a list won’t be copied.
2) list.reverse()
The Python list method reverse() reverses the items of a list in place. It doesn’t take any argument.
This method is useful when we want to change the ordering to either ascending or descending after sorting.
Example 14: We have sorted our list in ascending order, then later, we want to get the descending order of the sorted list. We could also use the Python list method sort() and set reverse to True, or we could use the reverse() list method as shown below.
numbs = [3,4,1,8,0,44,5] # our list of disordered numbers
numbs
[3, 4, 1, 8, 0, 44, 5]
numbs.sort() # sort, in ascending by default
numbs
[0, 1, 3, 4, 5, 8, 44]
numbs.reverse() # reverse to arrange in descending order
numbs
[44, 8, 5, 4, 3, 1, 0]FAQs
Q #1) How do I remove something from a list in Python?
Answer: We can remove items from a list in several ways using the Python list methods and the Python built in function del.
Remove using list.remove(x): This will remove item x from the list.
a = [4, 3, 6, 1] # our list of numbers
a
[4, 3, 6, 1]
a.remove(3) # remove item '3' from the list
a
[4, 6, 1]Remove using list.pop([i]): This is used to delete and return an item at any position in the list. If an index position is not given, it deletes the item at the end of the list.
a = [4, 3, 6, 1] # our list of numbers
a.pop() # remove the last item in the list
1
a
[4, 3, 6]
a.pop(1) # remove the item at index position 1
3
a
[4, 6]Remove using list.clear(): This will remove all items in the list.
a = [4, 3, 6, 1] # list of numbers
a
[4, 3, 6, 1]
a.clear() # delete all items
a
[]
Remove using del: The Python built-in function del can be used to delete items from the list.
a = [4, 3, 6, 1] # list of numbers
a
[4, 3, 6, 1]
del a[0] # delete item at index 0
a
[3, 6, 1]
del a[1] # delete item at index 1
a
[3, 1]
del a[:] # delete all items. Same as clear()
a
[]Q #2) How do you sort a list in Python?
Answer: To sort lists in Python, we can use the Python list method sort(). This method sorts the list in place and takes in two keyword arguments key and reverse.
The reverse keyword can be set to sort in either ascending or descending order. Alternatively, we can use Python’s built-in function sorted(). However, this doesn’t sort in place.
Q #3) Can you append multiple items to a list in Python?
Answer: We can append multiple items to a list by using the Python list method append() in a loop, where we loop through an iterable and append each item to the list.
def append_loop():
list1 = [0,1,2,3] # list 1
list2 = [4,5,6,7] # list 2
# iterate through ‘list 2’ using ‘for loop’, and append each item to list1
for item in list2:
list1.append(item)
print("Concatenated list is {}".format(list1))
Enter fullscreen mode
Exit fullscreen mode
if name == "main":
append_loop()
Output
multiple items to a list in Python
We can also append multiple items using the Python list method extend(). It works like “append() in a for loop” as we saw above.
list1 = [0,1,2,3] # list 1
list2 = [4,5,6,7] # list 2
list1.extend(list2) # append each item in list2 into list1
list1
[0, 1, 2, 3, 4, 5, 6, 7]Alternatively, we could concatenate using the + operator. However, this doesn’t work in place. Meaning it will return the concatenated list while the original lists stay unchanged.
list1 = [0,1,2,3] # list 1
list2 = [4,5,6,7] # list 2
list3 = list1 + list2 # concatenate using the + operator
list3
[0, 1, 2, 3, 4, 5, 6, 7]
Conclusion
In this tutorial, we went through some common Python list methods like append, extend, insert, pop, remove, clear, index, count, copy, and reverse.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK