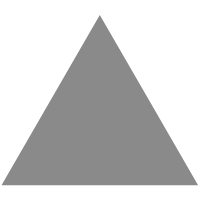
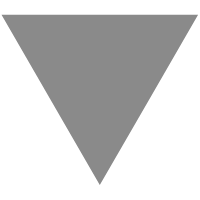
What is a React Component?
source link: https://dev.to/coderamrin/what-is-a-react-component-api
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
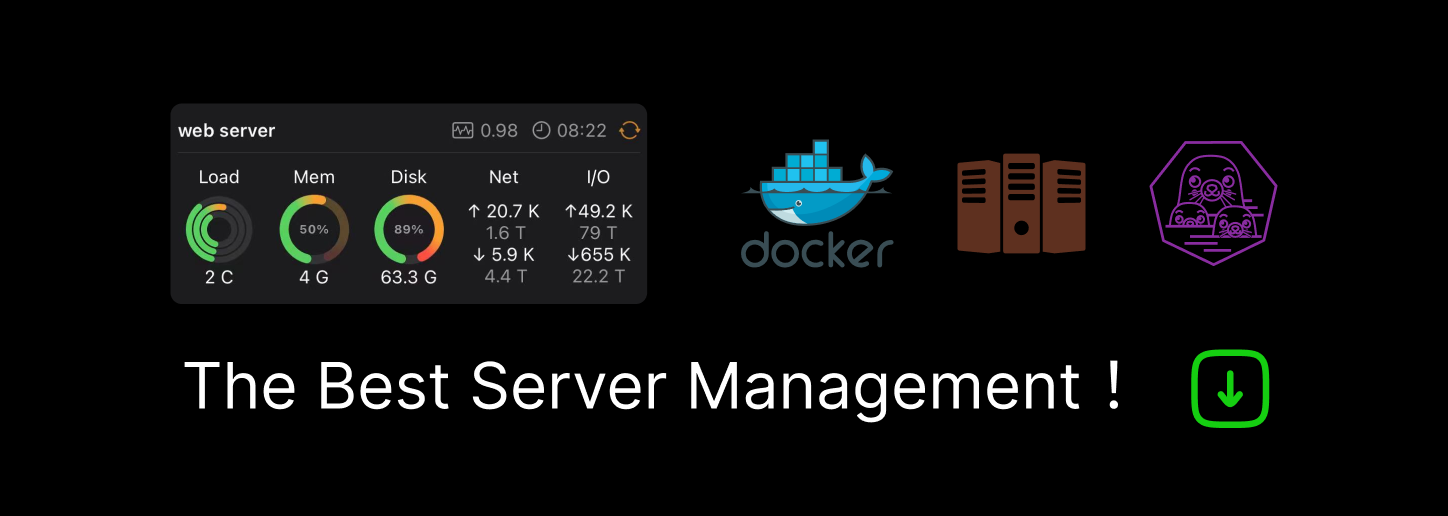
Hey there!
In this article, we are going to see what is a Component in React.js.
If you prefer video, then check it out
In short, React components are a function or a class that returns Html.
Components come in two types Class component and Functional component.
Just like a function component takes an argument called props.
Here's an example of a Functional Component:
import React from 'react';
function FunctionalComponent(props) {
return (
<div>
<h2>{props.greeting} world form functional component</h2>
</div>
);
}
export default FunctionalComponent;
Enter fullscreen mode
Exit fullscreen mode
A Functional component is just a plain old JavaScript function. It could also be an arrow function.
In a functional component, we access props from the props argument.
Here's an example of a Class Component:
import React, { Component } from 'react';
class ClassComponent extends Component {
render() {
return (
<div>
<h2 className='class'>
{this.props.greeting} world form Class component
</h2>
</div>
);
}
}
export default CassComponent;
Enter fullscreen mode
Exit fullscreen mode
Here we created a simple Class component. To create a Class Component we need to first import the "Component" component from React. Then extends it to create a Class Component.
In a class component, we access props from "this.props".
To render these components, import them inside the app.js file and call them like this:
function App() {
return (
<div className='App'>
<ClassComponent greeting={"Hello" }/>
<FunctionalComponent greeting={"Hello" }/>
</div>
);
}
Enter fullscreen mode
Exit fullscreen mode
Conclusion:
That's it for today.
If you liked it don't forget to give it a like. And if you want to add anything to it please leave a comment below.
Thanks for reading.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK