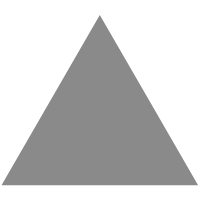
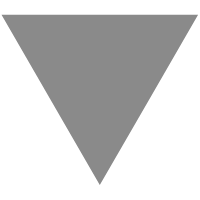
JSON.stringify和JSON.parse
source link: https://segmentfault.com/a/1190000041124982
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
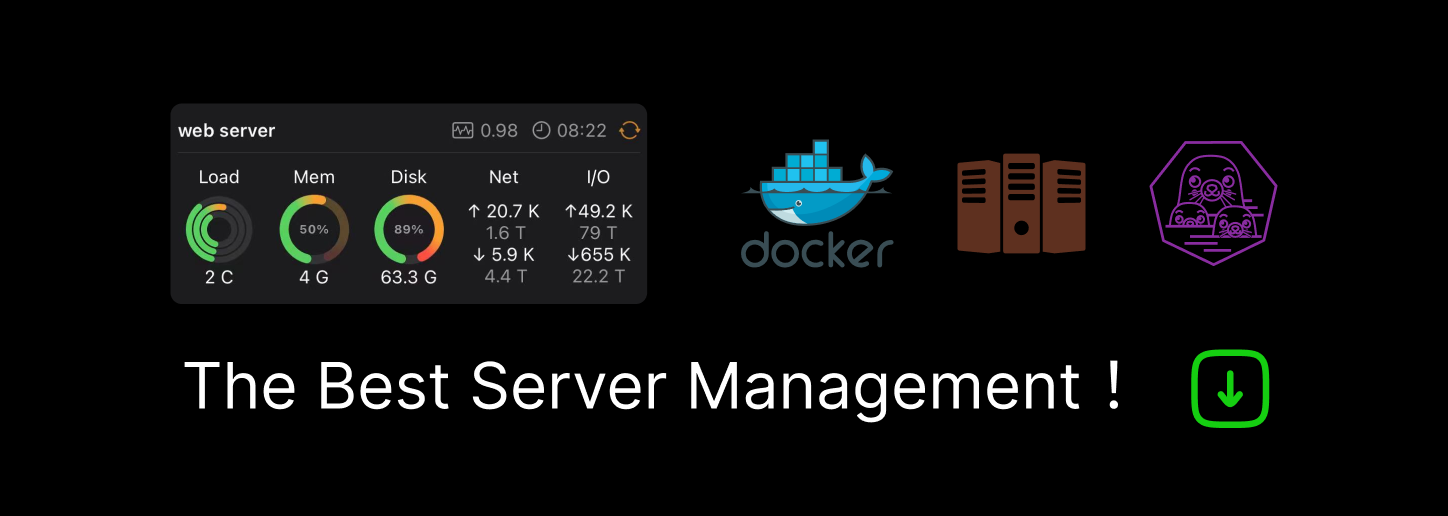
JSON 对象包含两个方法:一是用于解析成 JSON 对象的 parse();二是用于将对象转换为 JSON 字符串方法的 stringify()。这两个方法比较常用
JSON.parse
该方法的语法为:JSON.parse(text[, reviver])
参数说明:text:必需, 一个有效的 JSON 字符串。
reviver: 可选,一个转换结果的函数, 将为对象的每个成员调用此函数。
第二个参数使用例子,第二个参数可以将待处理的字符串进行一定的操作处理
let result = JSON.parse('{"a":3}', (k, v) => { if (k === "") return v return v * 2 }) console.log(result) // {a:6}
JSON.stringify
MDN描述
avaScript 对象或值转换为 JSON 字符串,默认该方法有三个参数
JSON.stringify(value[, replacer[, space]])
value:必需, 要转换的 JavaScript 值(通常为对象或数组)。
replacer:可选。用于转换结果的函数或数组。如果 replacer 为函数,则 JSON.stringify 将调用该函数,并传入每个成员的键和值。使用返回值而不是原始值。如果此函数返回 undefined,则排除成员。根对象的键是一个空字符串:""。如果 replacer 是一个数组,则仅转换该数组中具有键值的成员。成员的转换顺序与键在数组中的顺序一样。
space:可选,文本添加缩进、空格和换行符,如果 space 是一个数字,则返回值文本在每个级别缩进指定数目的空格,如果 space 大于 10,则文本缩进 10 个空格。space 也可以使用非数字,如:\t。
JSON.stringify({ x: [1, undefined, function(){}, Symbol('')] }) // {"x":[1,null,null,null]} function replacer(key,value){ if(typeof value === "string"){ return undefined } return value } var foo = {a:"aa",b:"bb",c:1,d:"d",e:5} // 通过替换方法把对象中的属性为字符串的过滤掉 var jsonstr1= JSON.stringify(foo,replacer) console.log(jsonstr1) // {"c":1,"e":5} // 第三个参数传入的是多个空格的时候,则会增加结果字符串里面的间距数量 console.log(JSON.stringify({a:1},null," ")) // { // "a": 1 // } console.log(JSON.stringify({a:1},null,"")) // {"a":1}
JSON.stringify方法的实现要考虑到各种数据类型输出的实际情况。
输入输出undefined"undefined"boolean"true/false"number字符串类型数字symbolundefinednull"null"stringstringNaN Infinity"null"functionundefinedArray数组中出现 undefined,function,symbol"null"RegExp"{}"DateDate的toJson()字符串,对包含循环引用的对象(对象之间相互引用,形成无限循环)执行此方法,会抛出错误。普通的Object如果有toJson()方法,序列化toJson()返回值;如果属性中出现undefinde,函数,symbol,忽略;所有以Symbol为属性键的属性都会被忽略,即便 replacer 参数中强制指定包含了它们。此外, 其他类型的对象,包括 Map/Set/WeakMap/WeakSet,仅会序列化可枚举的属性
function myJsonStringfy(e) { let type = typeof e; // typeof [] 'object' // typeof {} 'object' if (type !== "object") { let result = e; // 基础数据类型处理 if (Number.isNaN(e) || e === Infinity || e === -Infinity) { //NaN 和 Infinity 序列化返回 "null" result = "null" } else if (type === "function" || type === "undefined" || type === "symbol") { // 由于 function 序列化返回 undefined,因此和 undefined、symbol 一起处理 return undefined } else if (type === "string") { return `"${e}"` } return String(result) } else if (type === "object") { // 处理typeof null 结果是object历史遗留BUG console.log(e, e.toJSON) if (e === null) { return "null" } else if (e.toJSON && typeof e.toJSON === "function") { return myJsonStringfy(e.toJSON()) } else if (e instanceof Array) { //处理数组 let result = [] e.forEach((item, index) => { // Array数组中出现 undefined,function,symbol 返回字符串null if (typeof item === "undefined" || typeof item === "function" || typeof item === "symbol") { result[index] = "null"; } else { result[index] = myJsonStringfy(item) } }) result = `[${result}]` return result.replace(/'/g, '"'); } else { // 普通对象情况 let result = [] Object.keys(e).forEach(item => { console.log("...", item, typeof item) if (typeof item !== "symbol") { // key 如果是 symbol 对象,忽略 if (e[item] !== undefined && typeof e[item] !== 'function' && typeof e[item] !== 'symbol') { //键值如果是 undefined、function、symbol 为属性值,忽略 result.push('"' + item + '"' + ":" + myJsonStringfy(e[item])); } } }) return ("{" + result + "}").replace(/'/g, '"'); } } }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK