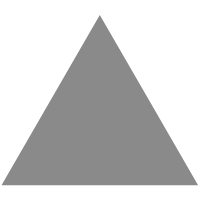
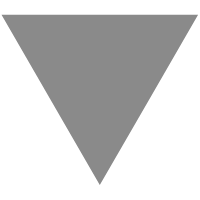
Writing system exits in C (and compiling them). – ColinPaice
source link: https://colinpaice.blog/2021/12/15/writing-system-exits-in-c-and-compiling-them/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
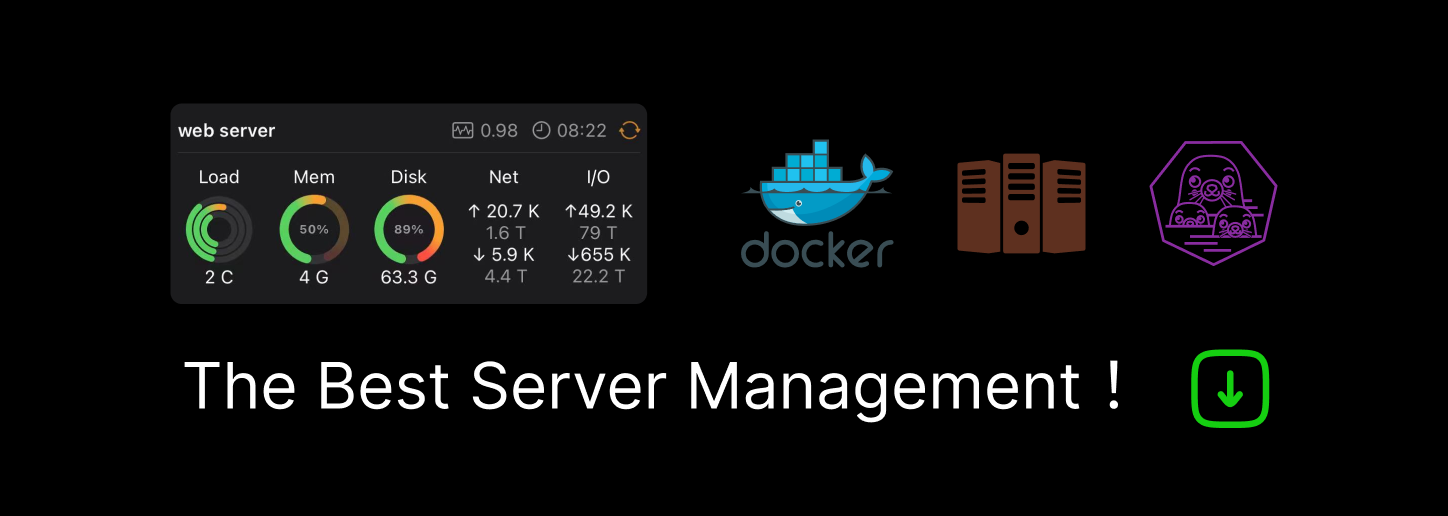
Writing system exits in C (and compiling them).
colin paice C, REXX, Z/OS December 15, 2021
4 Minutes
I wanted to call a C program from Rexx to do some special processing. The C programming guide gave me some hints, but I found it was a struggle to do it. It reminded me of when I was young and my father gave me a “beginners electronics kit” where had transistors, resisters, etc. You could build a “computer” that counted to 3, and make a radio. Unfortunately the instructions that came in it were in German, and for a different model kit to what I had. As a result it was very difficult to get working, but once you knew it was easy.
In the C programming guide there were instruction like “The CSECT must be the module entry point.” without saying which CSECT to use. They gave some sample programs, but not the JCL to compile them. After many failures, (looking at dumps and traces) I found you had to compile the C programs with “NORENT” which went against many years of experience.
I was using the System Programming C facility, which can be used, for example as z/OS exits. Note: This is different to Metal C, which allows you to include assembler code in your C program.
Some background
- These programs do not have a main() but are invoked with a z/OS type parameter list.
- They can use C facilities, such as printf, but not LE functions.
- You cannot use the UNIX file system functions.
- They need to be called with the C environment set up. You cannot just branch to the entry point.
- You can have several functions in the same source file. You branch to the one of interest.
Simple case
My C program was
#pragma environment(CPPROGH)
#pragma csect (CODE, “OEMPUT”)
int CPPROGH(int * p, evalblock * pEval, char * env) {
….
return 0;
}
The pragma environment said set up the C environment before calling executing this function. It takes the standard z/OS parameter list.
I needed some glue code to take the parameters from Rexx and store them in a parameter list for the function.
This glue codes saves parameters from R0,and 16(r1) and 20 (r1), then executes the function.
ENVA RMODE ANY
ENVA AMODE 31
ENVA CSECT
...
L R3,16(R1) a(Parmlist)
ST R3,Parmlist+0
L R3,20(R1) a(evalblk)
L R3,0(R3)
ST R3,Parmlist+4
ST R0,PARMLIST+08 A(env block)
OI PARMLIST+08,X'80'
la r1,parmlist
L R15,=V(CPPROGH)
BASR R14,R15
I wanted this to be called from REXX, which passes parameters in R0 and R1, so I had to write some glue code to store the parameters in storage before passing them to the program.
I compiled the glue code with
//GLUE EXEC PGM=ASMA90,PARM=’DECK,NOOBJECT,LIST,XREF(SHORT),NORENT’,
// REGION=0M
//SYSLIB DD DISP=SHR,DSN=CEE.SCEEMAC
// DD DSN=SYS1.MACLIB,DISP=SHR
//SYSUT1 DD UNIT=SYSDA,SPACE=(CYL,(1,1))
//SYSPUNCH DD DISP=SHR,DSN=COLIN.C.REXX.OBJ(GLUE2)
//SYSPRINT DD SYSOUT=*
//SYSIN DD DISP=SHR,DSN=COLIN.C.REXX(GLUE2)
//*
and compiled the C code with
//S1 JCLLIB ORDER=CBC.SCCNPRC
// SET LOADLIB=COLIN.C.REXX.LOAD
// SET LIBPRFX=CEE
//COMPILE EXEC PROC=EDCCB,
// LIBPRFX=&LIBPRFX,
// CPARM=’OPTFILE(DD:SYSOPTF),NORENT‘,
// BPARM=’SIZE=(900K,124K),RENT,LIST,XREF,RMODE=ANY,AMODE=31′
//COMPILE.SYSOPTF DD DISP=SHR,DSN=COLIN.C.REXX(CPARMS)
//COMPILE.SYSIN DD DISP=SHR,DSN=COLIN.C.REXX(CPPROGHE)
//BIND.SYSLMOD DD DISP=SHR,DSN=&LOADLIB.
//BIND.SYSLIB DD DISP=SHR,DSN=CEE.SCEESPC
// DD DISP=SHR,DSN=CEE.SCEELKED
//BIND.OBJLIB DD DISP=SHR,DSN=COLIN.C.REXX.OBJ
//BIND.SYSIN DD *
INCLUDE OBJLIB(GLUE2)
ENTRY ENVA
NAME COLIN(R)
/*
The EDCCB procedure to compile and bind, stores the object deck in a temporary file then passes this file and BIND.SYSIN into the binder.
C persistent environment.
The previous example created a C environment, ran my program, and deleted he C environment. If you want to do many calls to C functions you can set up a Persistent C environment. In this environment you do
- From assembler, set up the environment
- From assembler, use the environment, and call functions with your program as many times as you need
- From assembler close down the environment,
This is well documented in the C programming guide, (but not how to compile it).
The essence of my program was
Set up the environment
L R15,=V(EDCXHOTL)
BASR R14,R15
Call my function
LA R4,HANDLE
LA R5,USEFN This has the
STM R4,R5,PARMLIST
* now the user paramaters
...
OI PARMLIST+16,X'80'
LA R1,PARMLIST
L R15,=V(EDCXHOTU)
BASR R14,R15
...
USEFN DC V(CPPROGH) << This function name
Clean up
LA R1,PARMLIST
OI 0(R1),X'80'
L R15,=V(EDCXHOTT)
BASR R14,R15
My C program was
#pragma linkage(CPPROGH,OS)
int CPPROGH(int * p, evalblock * pEval, char * env) {
printf(“in CPPROG\n”);
return 0}
In this case the pragma is LINKAGE(CPPROGH,OS). The previous, self contained code, had ENVIRONMENT(CPPROGH). You need to use the right one.
Which procedure do I use to compile?
The C books describe the various procedures, for example EDCCB for compile and BIND, and EDCCL for compile and LINKEDIT. They do the same thing. The LINKEDIT uses program HEWL to link edit. The BIND uses IEWL to invoke the binder. These are both aliases to the binder IEWBLINK.
What’s the difference between BALR and BASR?
When coding, my fingers automatically used BALR (Branch and Link Register). This worked fine, but I should have used BASR (Branch and Save Register). As the Principles of Operation (POP) says
It is recommended, however, that BRANCH AND SAVE (BAS and BASR) be used instead and that BRANCH AND LINK be avoided since it places nonzero information in bit positions 32-39 of the general register in the 24-bit addressing mode, which may lead to problems and may decrease performance.
In 31 bit mode with BALR 14,15, the return address is stored in register 14. ‘1’ followed by the 31 it address.
In 24 bit mode, the return address has other information at the top, including the condition code. Most of the time this information will be ignored.
So using BALR is not wrong, it is that BASR is better.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK