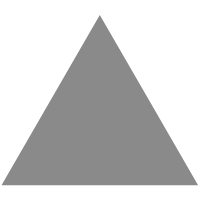
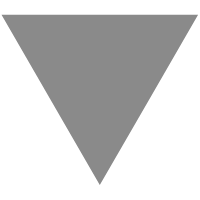
Laravel 7 - Column sorting with pagination example
source link: https://www.laravelcode.com/post/laravel-7-column-sorting-with-pagination-example
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
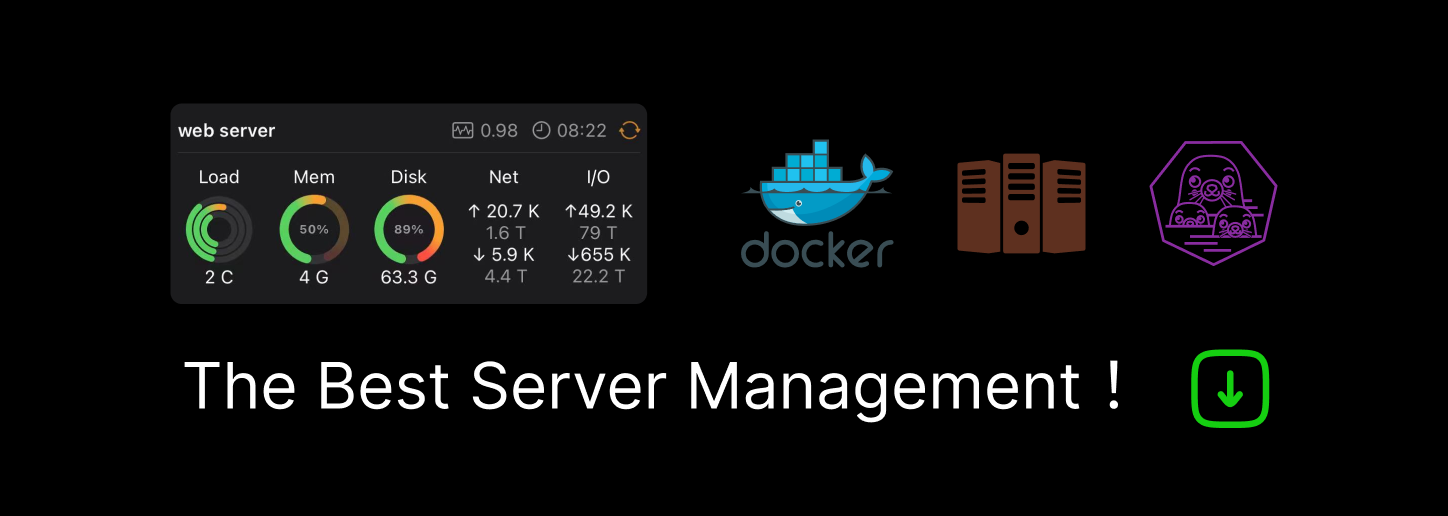
Laravel 7 - Column sorting with pagination example
In this article, I will share with you how to sorting on a column with pagination in laravel 7 using kyslik/column-sortable laravel package. column sorting is a very basic functionality when you show some records or data in table format. you also achieve this functionality by default in laravel if you use the data table in your laravel application. but if you don't won't to use the datatable in your application and you want to make column sorting functionality in your laravel application then here i will show you how to done column sorting with the kyslik/column-sortable laravel package. just follow all step.
Step - 1 : Create Laravel Application
First, we need to create the laravel application using the following composer command in your terminal. if you want to implement a column sorting functionality in your already existing laravel application then skip this one.
composer create-project --prefer-dist laravel/laravel myLaravelApp
Step - 2 : Install kyslik/column-sortable Package
Then the second step you need to install the kyslik/column-sortable in your laravel 7 application using the following composer command run in your terminal application's root directory.
composer require kyslik/column-sortable
Step - 3 Configure package
After install kyslik/column-sortable
package then you should configure some settings in laravel application. just following these small things. open your config/app.php file and set the following values into the provider's array.
'providers' => [
....
Kyslik\ColumnSortable\ColumnSortableServiceProvider::class,
]
.....
You can publish the default configuration file by the following command.
php artisan vendor:publish --provider="Kyslik\ColumnSortable\ColumnSortableServiceProvider" --tag="config"
after the public package's service provider then the columnsortable.php file created in the config
folder. there you can change the by default setting.
Step - 4 : Create Product Table and Model
In this step, we will create the first product table migration using the following artisan command.
php artisan make:migration create_product_table
after the run above command then the open created product's migration file and make changes the following in it.
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateProductsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->string('price');
$table->text('details');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('products');
}
}
after done changes then the run migration using the following artisan command in your terminal.
php artisan migrate
now let's make the app/Product.php
model file.
namespace App;
use Illuminate\Database\Eloquent\Model;
use Kyslik\ColumnSortable\Sortable;
class Product extends Model
{
use Sortable;
protected $fillable = [ 'name', 'price','details' ];
public $sortable = ['id', 'name', 'price','details', 'created_at', 'updated_at'];
}
Step - 5 : Create Route
Now, create the one following route in the routes/web.php
path.
Route::get('products-listing', 'ProductController@index');
Step - 6 : Create ProductController Controller
After create the route then we need to create also ProductController
controller using the following command run in your terminal or cmd.
php artisan make:controller ProductController
After running this command your ProductController.php
file automatically created on app/Http/Controllers
a folder. just open it and write the following code into that file.
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Product;
class ProductController extends Controller
{
/**
* Display the products dashboard.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$products = Product::sortable()->paginate(5);
return view('products',compact('products'));
}
}
Step - 6 : Create products View File
After done the controller file then we create one laravel blade HTML file which displays all product listing. Now we are creating one simple blade file in resources/views/producs.blade.php
file and here we are make a very simple HTML layout for listing the product information in the table.
<html>
<head>
<title>Laravel 7 - Column sorting with pagination example - laravelcode.com</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous">
</head>
<body>
<div class="container">
<h3 class="text-center">Laravel 7 - Column sorting with pagination example - laravelcode.com</h3>
<table class="table table-bordered">
<tr>
<th width="80px">@sortablelink('id')</th>
<th>@sortablelink('name')</th>
<th>@sortablelink('price')</th>
<th>@sortablelink('details')</th>
<th>@sortablelink('created_at')</th>
</tr>
@if($products->count())
@foreach($products as $key => $product)
<tr>
<td>{{ $product->id }}</td>
<td>{{ $product->name }}</td>
<td>{{ $product->price }}</td>
<td>{{ $product->details }}</td>
<td>{{ $product->created_at->format('d-m-Y') }}</td>
</tr>
@endforeach
@endif
</table>
{!! $products->appends(\Request::except('page'))->render() !!}
</div>
</body>
</html>
now we ready to run our application and let's look at the final output. just run the laravel application by running the following artisan command in your terminal.
php artisan serve
then open your web browser then simply run the following localhost URL in it.
http://localhost:8000/products-listing
i hope you like this article.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK