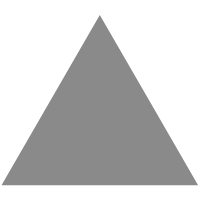
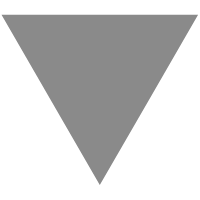
.NET知识梳理——3.面向对象
source link: https://www.cnblogs.com/Olive116/p/12344346.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
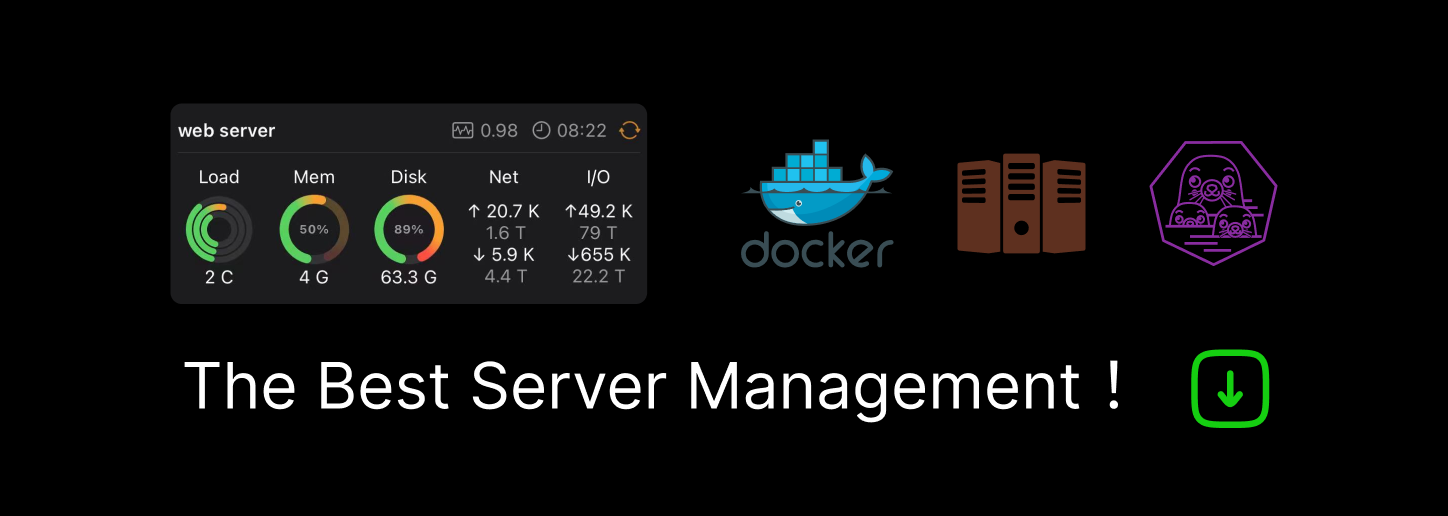
1. 面向对象
1.1 封装、继承、多态理解
1.1.1 封装
封装就是将抽象得到的数据和行为(或功能)相结合,形成一个有机的整体,也就是将数据与操作数据的源代码进行有机的结合,形成“类”,其中数据和函数都是类的成员。封装的目的是增强安全性和简化编程,使用者不必了解具体的实现细节,而只是要通过外部接口,以特定的访问权限来使用类的成员。降低耦合、提高重用性。
1.1.2 继承
子类拥有父类的一切属性和行为。可以实现代码的重用,.NET中类只能单继承。
1.1.3 多态
同一操作作用于不同的对象,可以有不同的解释,产生不同的执行结果,这就是多态性。多态按照不同的维度有以下几种:
1.1.3.1 编译时多态
编译时的多态性是通过重载来实现的。对于非虚的成员来说,系统在编译时,根据传递的参数、返回的类型等信息决定实现何种操作。重载只是针对一个类内部的几个参数不同,名称相同的方法。
1.1.3.2 运行时多态
运行时的多态性就是指直到系统运行时,才根据实际情况决定实现何种操作。C#中运行时的多态性是通过覆写虚成员实现。
1.1.3.3 接口多态
一个类可以实现多个接口。
1.1.3.4 继承多态
一个基类可以扩展多个子类。
1.2 覆写override、重载overload
1.2.1 覆写override
子类对父类虚方法、抽象方法的重新实现。子类对父类的虚方法可重写也可不重写,对父类的抽象方法,必须重写,都需要用override关键字修饰,如下:
/// <summary>
/// 父类,抽象类
/// </summary>
public abstract class ParentClass
public ParentClass(string name)
Console.WriteLine($"This is ParentClass,My name is {name}");
public void CommonMethod()
Console.WriteLine("ParentClass CommonMethod");
/// <summary>
/// 虚方法,包含方法实现,可以被子类重写
/// </summary>
public virtual void VirtualMethod()
Console.WriteLine("ParentClass VirtualMethod");
/// <summary>
/// 抽象方法,子类必须实现该方法
/// </summary>
public abstract void AbstractMethod();
/// <summary>
/// 子类
/// </summary>
public class ChildClass:ParentClass
/// <summary>
/// 子类实例化的时候,要先进行父类的实例化
/// </summary>
public ChildClass()
:base("Olive")//调用父类构造函数
Console.WriteLine("This is ChildClass");
public new void CommonMethod()
Console.WriteLine("ChildClass CommonMethod");
/// <summary>
/// 重写父类的虚方法
/// </summary>
public override void VirtualMethod()
Console.WriteLine("ChildClass VirtualMethod");
base.VirtualMethod();//调用父类方法
/// <summary>
/// 实现父类的抽象方法
/// </summary>
public sealed override void AbstractMethod()
Console.WriteLine("ChildClass AbstractMethod");
ParentClass parent = new ChildClass();
Console.WriteLine("parent.CommonMethod()");
parent.CommonMethod();
Console.WriteLine("parent.VirtualMethod()");
parent.VirtualMethod();
Console.WriteLine("parent.AbstractMethod()");
parent.AbstractMethod();
结果如下:
由以上结果可以发现,子类用new修饰的方法,
运行ParentClass p=new ChildClass();
p.CommonMethod();
会输出ParentClass CommonMethod
这是因为class ChildClass继承于class ParentClass,现在ChildClass中的CommonMethod函数隐藏ParentClass中的CommonMethod,所以从ChildClass (包括继承于ChildClass的子类)的角度来看类中的CommonMethod就是ChildClass. CommonMethod,ParentClass的CommonMethod不可见,但是如果从ParentClass的角度来看ChildClass,ParentClass只认识类ChildClass中继承于类ParentClass的CommonMethod函数,对于ChildClass类中的CommonMethod它不可见,所以ParentClass a=new ChildClass ();a. CommonMethod ();相当于是调用了类ChildClass中继承于ParentClass的CommonMethod函数
1.2.2 重载overload
重载只是针对一个类内部的几个参数不同,名称相同的方法。
/// <summary>
/// 重载(编译时多态)
/// </summary>
/// <param name="name"></param>
public void CommonMethod(string name)
Console.WriteLine($"ChildClass.CommonMethod的重载:Name is {name}");
public void CommonMethod(int age)
Console.WriteLine($"ChildClass.CommonMethod的重载:Age is {age}");
public void CommonMethod(string name,int age)
Console.WriteLine($"ChildClass.CommonMethod的重载:Name is {name}, Age is {age}");
public void CommonMethod(int age,string name)
Console.WriteLine($"ChildClass.CommonMethod的重载:Age is {age}, Name is {name}");
1.3 抽象类、接口理解和选择
1.3.1 抽象类
抽象类提供多个派生类共享基类的公共定义,它既可以提供抽象方法,也可以提供非抽象方法。
抽象类不能实例化,必须通过继承由派生类实现其抽象方法,因此对抽象类不能使用new关键字,也不能被密封。另外,实现抽象方法由override方法来实现
抽象类就是父类+约束,父类是为了更好的代码重用,约束是为了多态变化。
/// <summary>
/// 父类,抽象类
/// </summary>
public abstract class ParentClass
public ParentClass(string name)
Console.WriteLine($"This is ParentClass,My name is {name}");
public void CommonMethod()
Console.WriteLine("ParentClass CommonMethod");
/// <summary>
/// 虚方法,包含方法实现,可以被子类重写
/// </summary>
public virtual void VirtualMethod()
Console.WriteLine("ParentClass VirtualMethod");
/// <summary>
/// 抽象方法,子类必须实现该方法
/// </summary>
public abstract void AbstractMethod();
1.3.2 接口
接口是包含一组方法的抽象类型,其中每一种方法都有其名称、参数和返回值。为了约束,告诉外部有哪些功能。
接口包含方法(不包含方法实现)、事件、属性、索引器,不包含字段、委托。
一个类可以实现多个接口,当一个类继承某个接口时,它不仅要实现该接口定义的所有方法,还要实现该接口从其他接口中继承的所有方法。
public interface ITestInterface
string Show(string name);
string Info { get; set; }
int this[int i] { get; }
event Action DoWorkHandler;
1.3.3 如何选择
接口着重于CAN-DO关系类型,而抽象类则偏重于IS-A式的关系。
抽象类应主要用于关系密切的对象,而接口最适合为不相关的类提供通用功能。如果只是为了约束,有哪些功能,则选择接口,如果包含代码的重用则选择抽象类。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK