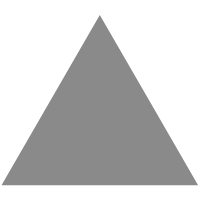
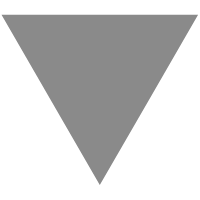
A tour to the Scala Tuples
source link: https://blog.knoldus.com/a-tour-to-the-scala-tuples/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
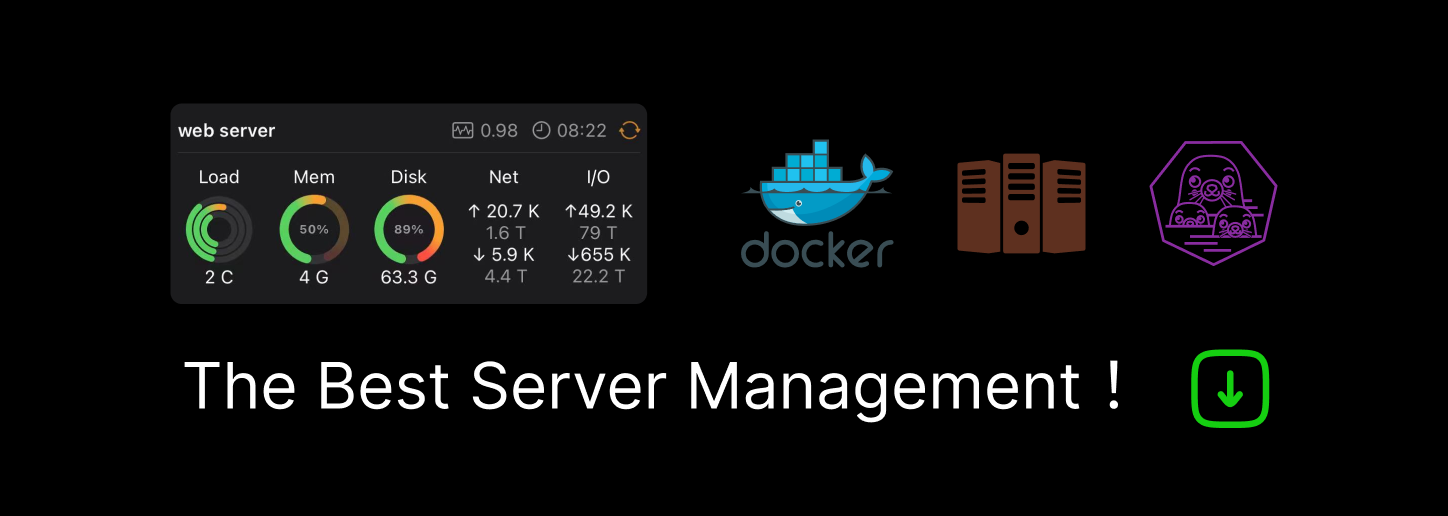
A tour to the Scala Tuples
Reading Time: 3 minutes
In Scala, a tuple is a class that gives us a simple way to store heterogeneous items or different data types in the same container. A tuples purpose is to combine a fixed and finite number of items together to allow the programmer to pass a tuple as a whole. A tuple is immutable in Scala. Click here to know more about mutability and immutability. An example of a tuple storing an integer, and a string value.
val t = (1, "hello world")
which is a syntactic sugar for the following-
val t = new Tuple2(1, "hello world")
The type of a tuple depends upon the number of elements it contains and the types of those elements. Thus, the type of (1, “hello world”) is Tuple2[Int, String]. Tuples can be of type Tuple1, Tuple2, Tuple3 and so on. Currently there is an upper limit of 22 in the Scala and if we need more, then we will have to use a collection, not a tuple.
Operations on Scala Tuple
Access element from tuple
One approach is to access them by element number, where the number is preceded by an underscore, method tuple._i is used to access the ith element of the tuple.
Pattern matching on Scala tuples
The another approach to access elements of a tuple is pattern-matching. It is a great way to assign tuple elements to variables. Pattern matching is a mechanism for checking a value against a pattern.
Another Example for pattern-matching a tuple:
val relation =
List(("Bob", "Father"), ("Jack", "Brother"), ("Rock", "Friend"))
relation.foreach{
case ("Jack", relation) =>
println(s"Jack is $relation of Alice")
case ("Bob", relation) =>
println(s"Bob is $relation of Alice")
case _ => println(relation(2)._1 + " has wrong relation with Alice")
}
The output for above code will be:
Bob is Father of Alice
Jack is Brother of Alice
Rock has wrong relation with Alice
Iterating over a Scala tuple
We can use productIterator() method to iterate over all the elements of a Tuple.
The foreach method takes function as a parameter and applies it to every element in the tuple, names.
Swapping the Tuple elements
We can use tuple.swap Method for Swapping the elements of a tuple.
Note: Technically, Scala 2.x tuples aren’t collections classes, they’re just a convenient little container. Because they aren’t a collection, they don’t have methods like map
, filter
, etc.
The best place where we can use tuple is, when we want to return multiple values from a method or pass multiple values to a function. This may be preferable instead of using a case class, particularly if we need to return unrelated values.
def getEncryptedCredential(userId: String, password: String)= {
//do some password encryption or hashing
(userId,password)
}
Now we can call that method and assign variable names to the return values:
val (encryptedUserId,encryptedPassword) = getEncryptedCredential("Admin", "XXXXXXXX")
We will get values for encryptedUserId and encryptedPassword variables as an output.
Conclusion
We introduced the tuple type in Scala and we learned how to create tuples and how to access values inside of tuple objects. As well we had gone through different operations that can be performed on a tuple. We also reviewed several useful methods and walked through some typical use cases.
References
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK