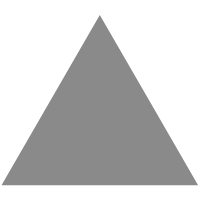
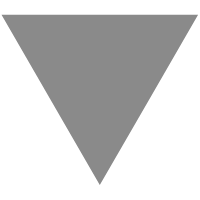
React router V6: Some of the new changes
source link: https://dev.to/gabrlcj/react-router-v6-some-of-the-new-changes-181m
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
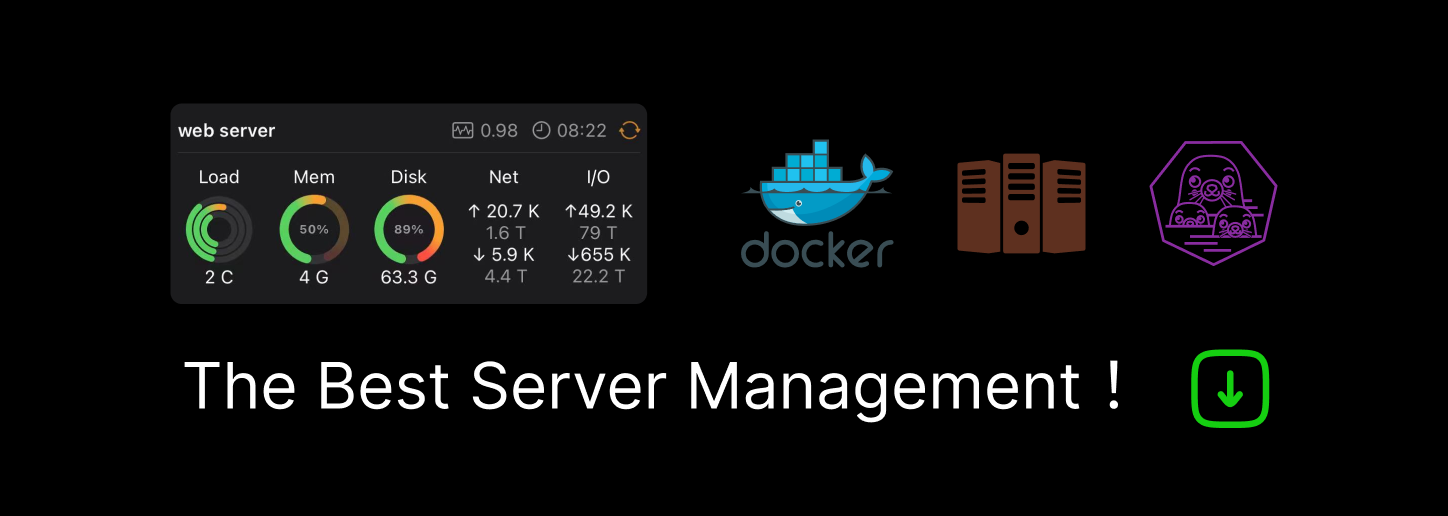
So not too long ago the library React Router has updated to version 6 and with that it came with some interesting changes that I notice and see people talking about, so I'm gonna describe some of them.
If you want to upgrade the version from 5 to 6 you can by seeing this link Upgrading React Router V5 to V6
First what is React Router?
React Router is a package for routing in React.js, as the documentation said "React Router is a fully-featured client and server-side routing library for React, a JavaScript library for building user interfaces. React Router runs anywhere React runs; on the web, on the server with node.js, and on React Native."
So now that we know the basics of it, let's talk about some new changes it had.
Replacing Switch component with Routes
If you have ever used React Router you know that we need to wrap our routes into this Switch component that makes sure that only one of these routes is loaded at the same time, instead of all matching routes. Something like this
export function App() {
return (
<div>
<Switch>
<Route path="/about">
<AboutPage />
</Route>
<Route exact path="/profile">
<ProfilePage />
</Route>
<Route path="/profile/:id">
<ProfileUserPage />
</Route>
</Switch>
</div>
)
}
Now with V6 we changed the name from Switch to Routes and now the Routes component has a new prop called element, where you pass the component it needs to render inside this component and be like this
export function App() {
return (
<div>
<Routes>
<Route path="/about" element={<AboutPage />} />
<Route exact path="/profile" element={<ProfilePage />} />
<Route path="/profile/:id" element={<ProfileUserPage />} />
</Routes>
</div>
)
}
Internal changes & path evaluation (no more needed exact prop)
So with this new version some internal changes were made and the evaluation that React Router does for these paths and then picking a route to load changed. For V5 we needed to put the exact prop on the component to go for that specific route we want, like this in the Profile path below
export function App() {
return (
<div>
<Routes>
<Route path="/about" element={<AboutPage />} />
<Route exact path="/profile" element={<ProfilePage />} />
<Route path="/profile/:id" element={<ProfileUserPage />} />
</Routes>
</div>
)
}
If we not put that exact prop it will render the path that starts with that path we pass and that's not what we wanted, now with V6 we don't need this prop anymore because React Router will always look for the exact path we pass, being like this
export function App() {
return (
<div>
<Routes>
<Route path="/about" element={<AboutPage />} />
<Route path="/profile" element={<ProfilePage />} />
<Route path="/profile/:id" element={<ProfileUserPage />} />
</Routes>
</div>
)
}
Now let's talk about the Link component that we have, that still is on V6
NavLink activeClassName prop does not exists anymore
With that prop you could pass a class for that specific Link to be modified with some CSS to show that it's became active, like this
export function Header() {
return (
<header>
<ul>
<li>
<NavLink activeClassName="active" to="/about" />
</li>
<li>
<NavLink activeClassName="active" to="/profile" />
</li>
</ul>
</header>
)
}
With V6 you have to manually do that and you can by passing a function to the className prop we have on React, like this
export function Header() {
return (
<header>
<ul>
<li>
<NavLink className={(navData) => navData.isActive ? "active" : "" } to="/about" />
</li>
<li>
<NavLink className={(navData) => navData.isActive ? "active" : "" } to="/profile" />
</li>
</ul>
</header>
)
}
Note that the React Router provides you the navData argument and it's an object and inside has the isActive property that will be true if the route is active in that moment
The useParams hook
This hook from V5 has not changed from V6, you can still use it the same way, passing parameters to match a specific URL you're trying to match.
Well these are some of the changes React Router had, if you wanna see more of these changes you can see this video from Academind where he talks about it Here or by reading the documentation Here. I see you next time, thanks!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK