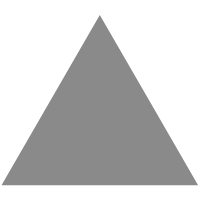
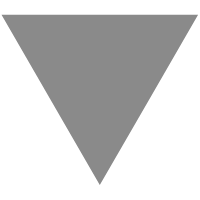
重写数组的方法(改变原数组)
source link: https://segmentfault.com/a/1190000040969572
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
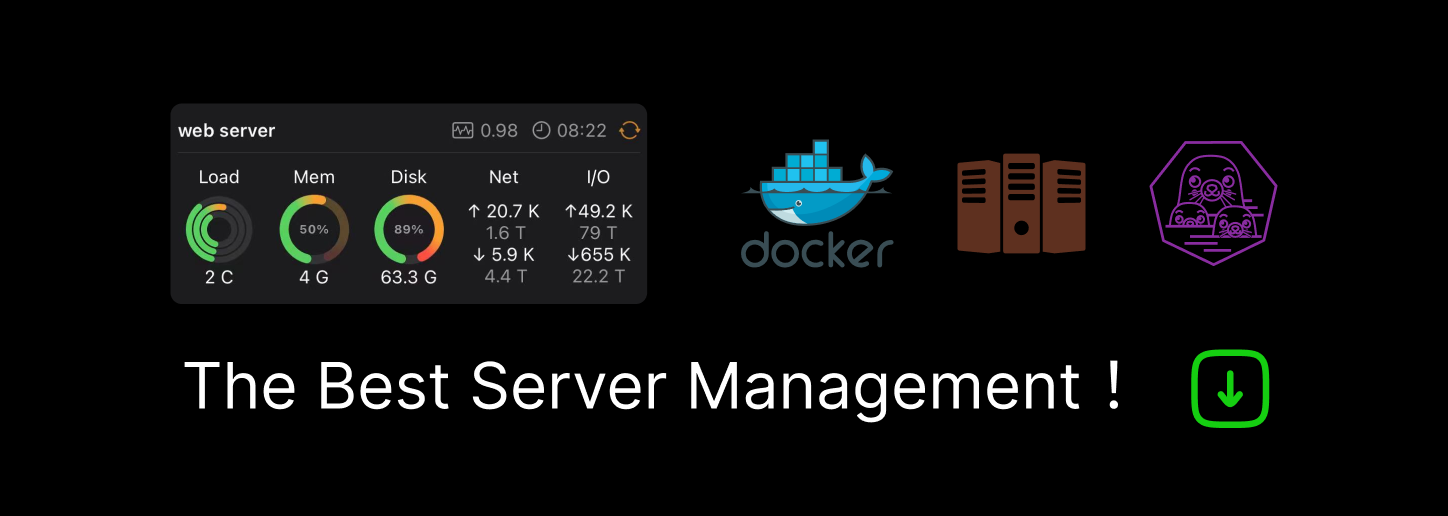
下图是我自我学习模拟数组时总结的一些重新数组的方法:
本文我们暂不讨论不改变原数组的方法,只谈改变原数组用到的 6 种方法。
改变原数组的方法
push()
按参数顺序向数组尾部添加元素,返回新数组的长度
var color = ['red', 'green'] var color2 = color2.push(['blue','purple']) alert(color) // ['red', 'green'] alert(color2) // ['red', 'green','blue','purple']
Array.prototype._push = function() { for(let i = 0; i < arguments.length; i++) { this[this.length] = arguments[i] } return this.length } var arr1 = [1, 2, 3] console.log(arr1.push(4, 5)) // 返回新数组的长度 5 console.log(arr1._push(6, 7)) // 返回新数组的长度 7 console.log(arr1) // [1, 2, 3, 4, 5, 6, 7]
pop()
删除数组中的最后一个元素,并返回该元素
var color = ['red', 'green','blue','purple'] var color2 = color.pop() alert(color) // ['red','green','blue'] alert(color2) // ['purple']
Array.prototype._pop() = function() { if(this.length) { let res = this[this.length - 1] delete this[this.length] this.length-- return res } } let arr2 = [1, 2, 3, 4, 5] console.log(arr2.pop()) // 返回删除的元素 5 console.log(arr2._pop()) // 返回删除的元素 4 console.log(arr2) // [1, 2, 3]
sort()
默认情况下,sort() 会按照升序重新排列数组元素,即最小的值在前面,最大的值在后面。因此,sort() 会在每一项上调用 string() 转换函数,然后比较字符串来决定顺序。即使数组的元素都是数值,也会先把数组转换成字符串再比较、排序。例如:
let values = [0, 1, 5, 10, 15] values.sort() console.log(values) //0,1,10,15,5
一开始数组中的数值的顺序是正确的,但是调用 sort() 会按照这些数值的字符串形式重新排序。所以它可以接收一个比较函数,用于判断哪个值应该排在前面。
function compare(a, b) { if(a < b) return -1 else if(a > b) return 1 else return 0 }
这个比较函数可以适用于大多数数据类型,可以把他当做参数传给 sort(),例如:
let values = [0, 1, 5, 10, 15] values.sort(compare) console.log(values) // 0,1,5,10,15
当然,也可以使排序产生降序效果,只需要把返回值交换一下即可:
function compare(a, b) { if(a < b) return 1 else if(a > b) return -1 else return 0 } let values = [0, 1, 5, 10, 15] values.sort(compare) console.log(values) // 15,10,5,1,0
var arr = [4, 1, 6, 9, 3, 2, 8, 7] var arr2 = [4, 1, 6, 9, 3, 2, 8, 7] console.log(arr.sort()); Array.prototype.mySort = function (arr) { for (var i = 0; i < this.length; i++) { for (var j = i + 1; j < this.length; j++) { if (this[i] > this[j]) { var temp = this[i] this[i] = this[j] this[j] = temp; } } } return this } console.log('mySort:',arr2.mySort());
reverse()
将数组倒叙,改变原数组
Array.prototype.myReverse = function () { var left = 0, right = this.length - 1; while (left < right) { var temp = this[left]; this[left] = this[right]; this[right] = temp; left++; right--; } } var arr2 = [1, 3, 5, 7, 9] console.log('before:', arr2) myReverse(arr2) console.log('after:', arr2)
shift()
删除数组第一个元素,并返回该元素
var arr = [1, 3, 5, 7] console.log(arr.unshift(9)) console.log(arr) Array.prototype.myUnshift = function () { var L = this.length; var newArr = arguments.length for (var i = L + newArr - 1; i >= 0; i--) { if (i > newArr - 1) { this[i] = this[i - newArr]; } else { this[i] = arguments[i]; } } return this.length; } var arr2 = [1, 3, 5, 7,] console.log('myUnshift:', arr2.myUnshift(9)); console.log('myUnshift:', arr2)
unshift()
向数组开头增加一个或多个元素,并返回新的长度
var arr = [1, 3, 5, 7] console.log(arr.unshift(9)) console.log(arr) Array.prototype.myUnshift = function () { var L = this.length; var newArr = arguments.length for (var i = L + newArr - 1; i >= 0; i--) { if (i > newArr - 1) { this[i] = this[i - newArr]; } else { this[i] = arguments[i]; } } return this.length; } var arr2 = [1, 3, 5, 7,] console.log('myUnshift:', arr2.myUnshift(9)); console.log('myUnshift:', arr2)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK