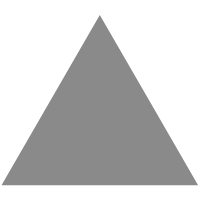
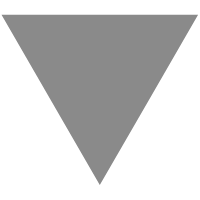
Usage of ESM modules in CommonJS world.
source link: https://gist.github.com/ShogunPanda/fe98fd23d77cdfb918010dbc42f4504d
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
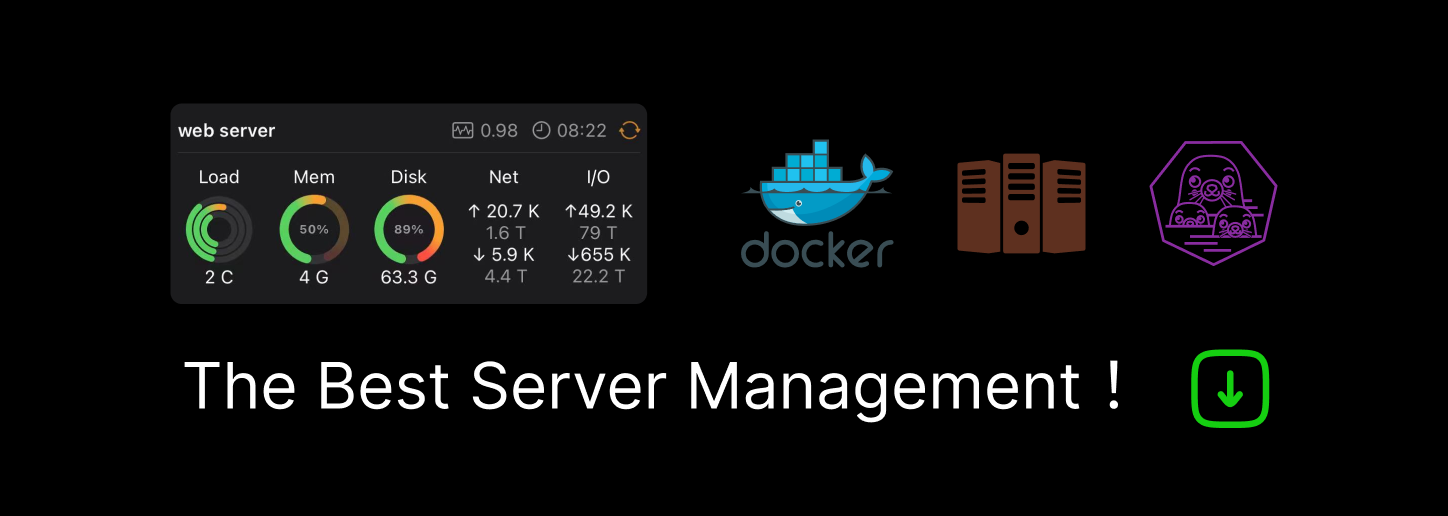
Import ESM module in a CommonJS module
Do not use require
s or top-level import
s (if you're transpiling server side code).
Replace all occurrences with await import
and wrap your code in a async function
.
Example
If your original code was:
const { someFunction } = require('some-module') someFunction('AWESOME!')
then your new code must be something like this:
async function main() { const { someFunction } = await import('some-module') someFunction('AWESOME!') } main().catch(console.error)
Import ESM modules in a TypeScript CommonJS module
Considering what we saw in the previous paragraph, you might incur into microsoft/TypeScript#43329. In that case the workaround is to define (using a new Function) which internally performs the import:
const importDynamic = new Function("modulePath", "return import(modulePath)") async function main() { const { someFunction } = await importDynamic('some-module') someFunction('AWESOME!') } main().catch(console.error)
Other resources
Recommend
-
14
README.md One Click, Offline, CommonJS Modules
-
15
-
19
All you need to know to move from CommonJS to ECMAScript Modules (ESM) in Node.jsPublished: 2021.05.05 | 5 minutes readOne of the most revolutionary features introduced as part of ECMAScript 2015 specification are modules (ESM...
-
9
Fix a read head-buffer-overflow in esm The check forgot to account for the terminal zero. Request to merge...
-
5
JavaScript的CJS、AMD、UMD、ESM都是啥在最开始JavaScript没有import / export模块这些机制。所有的代码都在一个文件里,这简直就是灾难。之后就出现了一些机制改变只...
-
9
Ubuntu 14.04 與 16.04 的 ESM 從八年延長到十年 本來的舊的 Ubuntu ESM 是額外的三年 (加上本來的 LTS 五年,共八年),14.04 會支援到 2022 年四月 (參考 I...
-
5
Welcome to the release notes for Yarn 3.1! We're quite excited by this release, as it brings various improvements that we've all been looking forward to. Let's dig into that! As always, keep in mind those are only the...
-
20
Pure ESM package Pure ESM package The package linked to from here is now pure ESM....
-
12
Moving gulpfile from CommonJS (CJS) to ECMAScript Modules (ESM) Context del v7.0.0 moved to pure ESM (no dual support), which forced me to move...
-
5
ESM to CJS We love ESM and think it is a great step in the right direction. Unfortunately like many developers, we still have to support CommonJS and cannot simply switch everything to ESM. The fact that some maintainers decided to make p...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK