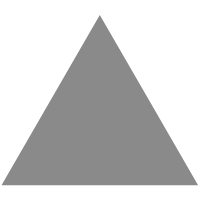
2
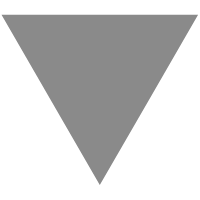
QuickJS 初探
source link: https://blog.drawoceans.com/codes/498/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
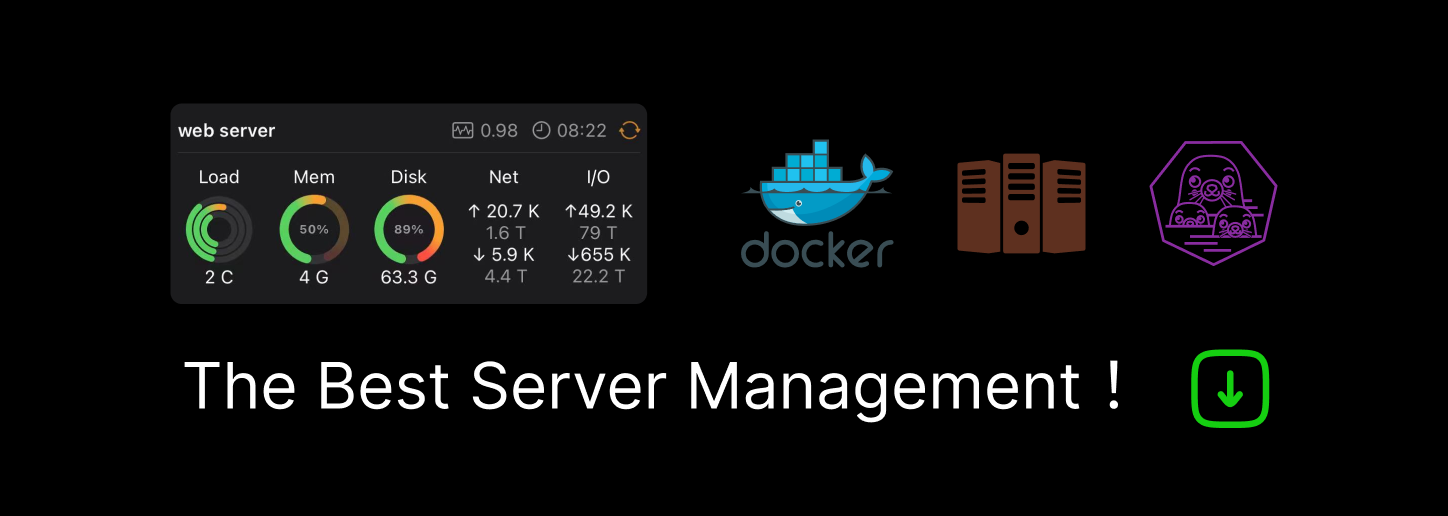
QuickJS 初探 – Drawoceans的博客
/* C++ doesn't support nested designators */
#ifndef __cplusplus
#define JS_MKVAL(tag, val) (JSValue){ (JSValueUnion){ .int32 = val }, tag }
#define JS_MKPTR(tag, p) (JSValue){ (JSValueUnion){ .ptr = p }, tag }
#define JS_NAN (JSValue){ .u.float64 = JS_FLOAT64_NAN, JS_TAG_FLOAT64 }
#else
static
inline
JSValue JS_MKVAL(
int64_t
tag,
int32_t
val) {
JSValue ret;
ret.tag = tag;
ret.u.int32 = val;
return
ret;
}
static
inline
JSValue JS_MKPTR(
int64_t
tag,
void
* p) {
JSValue ret;
ret.tag = tag;
ret.u.ptr = p;
return
ret;
}
static
inline
JSValue JS_NAN() {
JSValue ret;
ret.tag = JS_TAG_FLOAT64;
ret.u.float64 = JS_FLOAT64_NAN;
return
ret;
}
#endif /* __cplusplus */
1006行也是(Fabrice Bellard 大神还贴心地告诉你 C++ 不喜欢指派初始化器):
/* Note: c++ does not like nested designators */
#ifndef __cplusplus
#define JS_CFUNC_DEF(name, length, func1) { name, JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE, JS_DEF_CFUNC, 0, .u = { .func = { length, JS_CFUNC_generic, { .generic = func1 } } } }
#define JS_CFUNC_MAGIC_DEF(name, length, func1, magic) { name, JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE, JS_DEF_CFUNC, magic, .u = { .func = { length, JS_CFUNC_generic_magic, { .generic_magic = func1 } } } }
#define JS_CFUNC_SPECIAL_DEF(name, length, cproto, func1) { name, JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE, JS_DEF_CFUNC, 0, .u = { .func = { length, JS_CFUNC_ ## cproto, { .cproto = func1 } } } }
#define JS_ITERATOR_NEXT_DEF(name, length, func1, magic) { name, JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE, JS_DEF_CFUNC, magic, .u = { .func = { length, JS_CFUNC_iterator_next, { .iterator_next = func1 } } } }
#define JS_CGETSET_DEF(name, fgetter, fsetter) { name, JS_PROP_CONFIGURABLE, JS_DEF_CGETSET, 0, .u = { .getset = { .get = { .getter = fgetter }, .set = { .setter = fsetter } } } }
#define JS_CGETSET_MAGIC_DEF(name, fgetter, fsetter, magic) { name, JS_PROP_CONFIGURABLE, JS_DEF_CGETSET_MAGIC, magic, .u = { .getset = { .get = { .getter_magic = fgetter }, .set = { .setter_magic = fsetter } } } }
#define JS_PROP_STRING_DEF(name, cstr, prop_flags) { name, prop_flags, JS_DEF_PROP_STRING, 0, .u = { .str = cstr } }
#define JS_PROP_INT32_DEF(name, val, prop_flags) { name, prop_flags, JS_DEF_PROP_INT32, 0, .u = { .i32 = val } }
#define JS_PROP_INT64_DEF(name, val, prop_flags) { name, prop_flags, JS_DEF_PROP_INT64, 0, .u = { .i64 = val } }
#define JS_PROP_DOUBLE_DEF(name, val, prop_flags) { name, prop_flags, JS_DEF_PROP_DOUBLE, 0, .u = { .f64 = val } }
#define JS_PROP_UNDEFINED_DEF(name, prop_flags) { name, prop_flags, JS_DEF_PROP_UNDEFINED, 0, .u = { .i32 = 0 } }
#define JS_OBJECT_DEF(name, tab, len, prop_flags) { name, prop_flags, JS_DEF_OBJECT, 0, .u = { .prop_list = { tab, len } } }
#define JS_ALIAS_DEF(name, from) { name, JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE, JS_DEF_ALIAS, 0, .u = { .alias = { from, -1 } } }
#define JS_ALIAS_BASE_DEF(name, from, base) { name, JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE, JS_DEF_ALIAS, 0, .u = { .alias = { from, base } } }
#else
static
inline
JSCFunctionListEntry JS_CFUNC_DEF(
const
char
* name,
uint8_t
length, JSCFunction func1) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE;
ret.def_type = JS_DEF_CFUNC;
ret.magic = 0;
ret.u.func.length = length;
ret.u.func.cproto = JS_CFUNC_generic;
ret.u.func.cfunc.generic = func1;
return
ret;
}
using
JSCFuncMagic = std::add_pointer_t<JSValue(JSContext*, JSValue,
int
, JSValue*,
int
)>;
static
inline
JSCFunctionListEntry JS_CFUNC_MAGIC_DEF(
const
char
* name,
uint8_t
length, JSCFuncMagic func1,
int16_t
magic) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE;
ret.def_type = JS_DEF_CFUNC;
ret.magic = magic;
ret.u.func.length = length;
ret.u.func.cproto = JS_CFUNC_generic_magic;
ret.u.func.cfunc.generic_magic = func1;
return
ret;
}
#define CXX_JSCFENUM_GEN(cproto) JS_CFUNC_ ## cproto
static
inline
JSCFunctionListEntry JS_CFUNC_SPECIAL_DEF(
const
char
* name,
uint8_t
length, JSCFunctionEnum cproto, JSCFunction func1) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE;
ret.def_type = JS_DEF_CFUNC;
ret.magic = 0;
ret.u.func.length = length;
ret.u.func.cproto = cproto;
ret.u.func.cfunc.generic = func1;
return
ret;
}
using
JSCFuncIter = std::add_pointer_t<JSValue(JSContext*, JSValueConst,
int
, JSValueConst*,
int
*,
int
)>;
static
inline
JSCFunctionListEntry JS_ITERATOR_NEXT_DEF(
const
char
* name,
uint8_t
length, JSCFuncIter func1,
int16_t
magic) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE;
ret.def_type = JS_DEF_CFUNC;
ret.magic = magic;
ret.u.func.length = length;
ret.u.func.cproto = JS_CFUNC_iterator_next;
ret.u.func.cfunc.iterator_next = func1;
return
ret;
}
using
JSCFuncGetter = std::add_pointer_t <JSValue(JSContext*, JSValueConst)>;
using
JSCFuncSetter = std::add_pointer_t <JSValue(JSContext*, JSValueConst, JSValueConst)>;
static
inline
JSCFunctionListEntry JS_CGETSET_DEF(
const
char
* name, JSCFuncGetter fgetter, JSCFuncSetter fsetter) {
JSCFunctionListEntry ret;
ret.prop_flags = JS_PROP_CONFIGURABLE;
ret.def_type = JS_DEF_CGETSET;
ret.magic = 0;
ret.u.getset.get.getter = fgetter;
ret.u.getset.set.setter = fsetter;
return
ret;
}
using
JSCFuncGetterMagic = std::add_pointer_t <JSValue(JSContext*, JSValueConst)>;
using
JSCFuncSetterMagic = std::add_pointer_t <JSValue(JSContext*, JSValueConst, JSValueConst)>;
static
inline
JSCFunctionListEntry JS_CGETSET_MAGIC_DEF(
const
char
* name, JSCFuncGetterMagic fgetter, JSCFuncSetterMagic fsetter,
int16_t
magic) {
JSCFunctionListEntry ret;
ret.prop_flags = JS_PROP_CONFIGURABLE;
ret.def_type = JS_DEF_CGETSET;
ret.magic = magic;
ret.u.getset.get.getter = fgetter;
ret.u.getset.set.setter = fsetter;
return
ret;
}
static
inline
JSCFunctionListEntry JS_PROP_STRING_DEF(
const
char
* name,
const
char
* cstr,
uint8_t
prop_flags) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = prop_flags;
ret.def_type = JS_DEF_PROP_STRING;
ret.magic = 0;
ret.u.str = cstr;
return
ret;
}
static
inline
JSCFunctionListEntry JS_PROP_INT32_DEF(
const
char
* name,
int32_t
val,
uint8_t
prop_flags) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = prop_flags;
ret.def_type = JS_DEF_PROP_INT32;
ret.magic = 0;
ret.u.i32 = val;
return
ret;
}
static
inline
JSCFunctionListEntry JS_PROP_INT64_DEF(
const
char
* name,
int64_t
val,
uint8_t
prop_flags) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = prop_flags;
ret.def_type = JS_DEF_PROP_INT64;
ret.magic = 0;
ret.u.i64 = val;
return
ret;
}
static
inline
JSCFunctionListEntry JS_PROP_DOUBLE_DEF(
const
char
* name,
double
val,
uint8_t
prop_flags) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = prop_flags;
ret.def_type = JS_DEF_PROP_DOUBLE;
ret.magic = 0;
ret.u.f64 = val;
return
ret;
}
static
inline
JSCFunctionListEntry JS_PROP_UNDEFINED_DEF(
const
char
* name,
uint8_t
prop_flags) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = prop_flags;
ret.def_type = JS_DEF_PROP_UNDEFINED;
ret.magic = 0;
ret.u.i32 = 0;
return
ret;
}
static
inline
JSCFunctionListEntry JS_OBJECT_DEF(
const
char
* name,
struct
JSCFunctionListEntry* tab,
int
len,
uint8_t
prop_flags) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = prop_flags;
ret.def_type = JS_DEF_OBJECT;
ret.u.prop_list.tab = tab;
ret.u.prop_list.len = len;
ret.magic = 0;
return
ret;
}
static
inline
JSCFunctionListEntry JS_ALIAS_DEF(
const
char
* name,
const
char
* from) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE;
ret.def_type = JS_DEF_ALIAS;
ret.magic = 0;
ret.u.alias.name = from;
ret.u.alias.base = -1;
return
ret;
}
static
inline
JSCFunctionListEntry JS_ALIAS_BASE_DEF(
const
char
* name,
const
char
* from,
int
base) {
JSCFunctionListEntry ret;
ret.name = name;
ret.prop_flags = JS_PROP_WRITABLE | JS_PROP_CONFIGURABLE;
ret.def_type = JS_DEF_ALIAS;
ret.magic = 0;
ret.u.alias.name = from;
ret.u.alias.base = base;
return
ret;
}
#endif /* __cplusplus */
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK