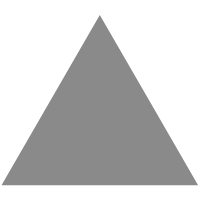
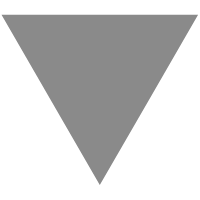
PHP: How do I call all methods of the same name in a class hierarchy?
source link: https://www.codesd.com/item/php-how-do-i-call-all-methods-of-the-same-name-in-a-class-hierarchy.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
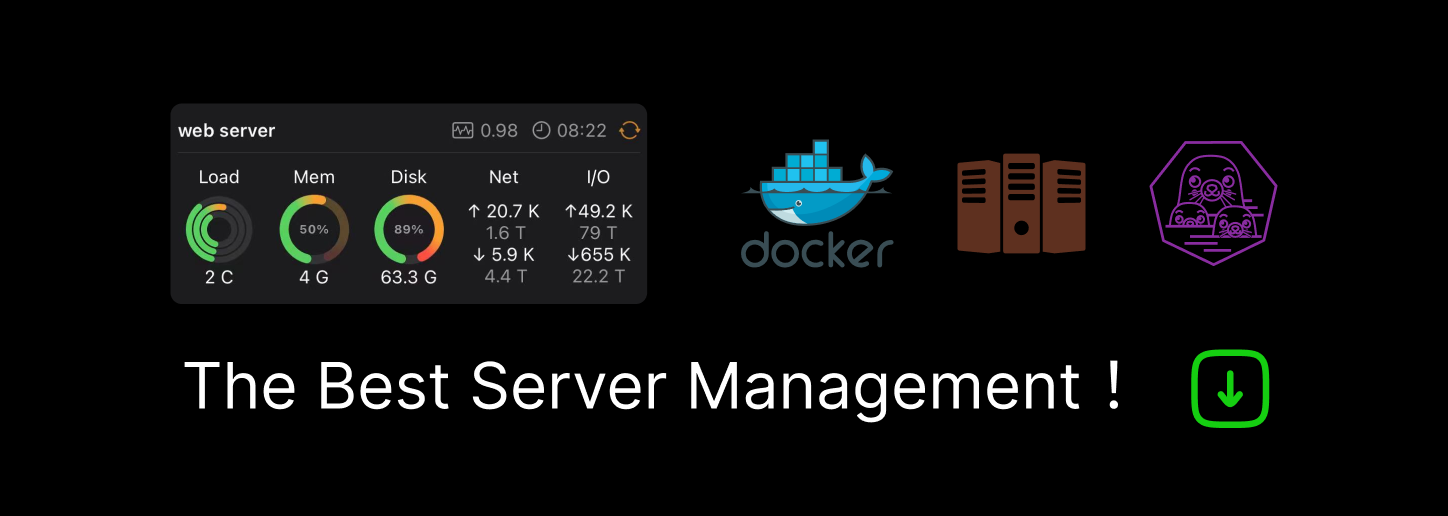
PHP: How do I call all methods of the same name in a class hierarchy?
I have a class hierarchy like the following:
class Alpha {
public function initialize() {
$class = get_class($this);
$family = array( $class );
while( ($class = get_parent_class($class)) !== false ) {
$family[] = $class;
}
$family = array_reverse($family);
foreach($family as $className) {
// call $className's start (instance) method
}
}
public function start() {
echo "calling start from Alpha\n";
}
}
class Beta extends Alpha {
public function start() {
echo "calling start from Beta\n";
}
}
class Charlie extends Beta {
public function start() {
echo "calling start from Charlie\n";
}
}
$c = new Charlie();
$c->initialize();
Alpha's initialize method should call the derived class's start method as well as all of the derived class's ancestor classes' start methods all the way back to Alpha's start method. The code should produce the following output:
calling start from Alpha
calling start from Beta
calling start from Charlie
However, I can't seem to figure out how to call an instance method of a specific ancestor class specified by the $className variable.
I've used call_user_func(array($className, 'start'))
but this causes the start method to be treated like a static function. Any ideas?
In class function call like Classname::start should call Classname's function start not static call
class Alpha {
public $myvar = 0;
public function initialize() {
$class = get_class($this);
$family = array( $class );
while( ($class = get_parent_class($class)) !== false ) {
$family[] = $class;
$this->myvar ++;
}
$family = array_reverse($family);
foreach($family as $className) {
// call $className's start method
eval("{$className}::start();");
}
}
public function start() {
echo "{$this->myvar} calling start from Alpha\n";
}
}
class Beta extends Alpha {
public function start() {
echo "{$this->myvar} calling start from Beta\n";
}
}
class Charlie extends Beta {
public function start() {
echo "{$this->myvar} calling start from Charlie\n";
}
}
$c = new Charlie();
$c->initialize();
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK