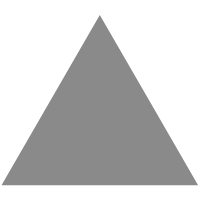
14
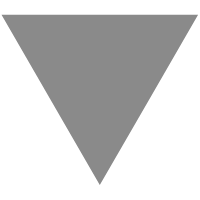
How do it fix this java code
source link: https://www.codeproject.com/Questions/5317028/How-do-it-fix-this-java-code
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
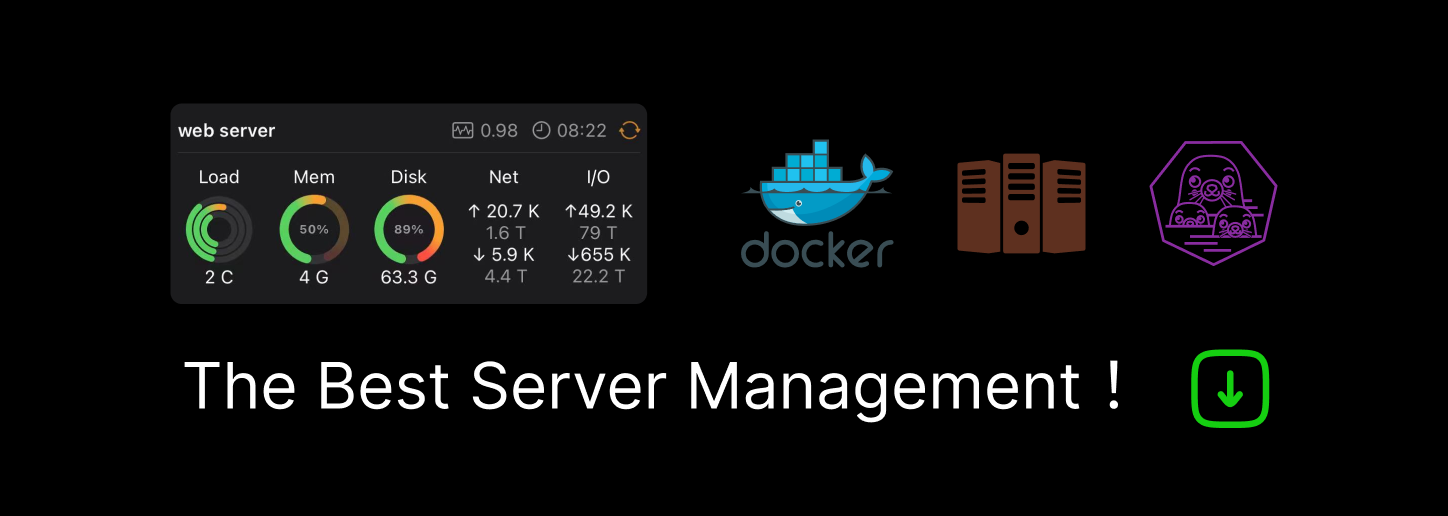
See more:
Expand ▼ Copy Code
A new seat discount system is required to help managers of a railway company determine how much discount they have given out. Every time the system runs, the discount rate may be specified by the managers or set to a suitable default. You are thusly required to produce a console application (using Java) that is ready to open the file M:\data\seats.txt which contains the seat’s data. Upon application launch, the system should ask the managers if they wish to specify a custom discount rate. This should be in the form of a yes / no question. When the managers say yes, the system should request the discount rate as input (from the keyboard) which will then overwrite the default discount rate (i.e. Appendix B). When the managers say no, the system should report (to the screen) the default discount rate (i.e. Appendix C). Next, the system should loop through the seat’s data, calculating and printing (to the screen) the seat type, seat price, number of bookings, discount and income following discount. The latter two require some basic calculations. Finally, the running totals for the discount and income following discount should be calculated and printed before the application gracefully exits. Appendix A- 1ST(Table) 48.50 2 1ST 44.50 3 STD(Table) 28.50 3 STD 24.50 5 Appendix B - - Seat Discount System - - Specify Custom Discount Rate [Y|N] : Y Specify Discount Rate (%) : 17.5 Seat Type : 1ST(Table), Seat Price : £48.50, Bookings : 2, Discount : £16.97, Income :£80.03 Seat Type : 1ST, Seat Price : £44.50, Bookings : 3, Discount : £23.36, Income :£110.14 Seat Type : STD(Table), Seat Price : £28.50, Bookings : 3, Discount : £14.96, Income :£70.54 Seat Type : STD, Seat Price : £24.50, Bookings : 5, Discount : £21.44, Income :£101.06 Total Income : £361.76 Total Discount : £76.74 Appendix C - - Seat Discount System - - Specify Custom Discount Rate [Y|N] : N Assuming Discount Rate = 20.0% Seat Type : 1ST(Table), Seat Price : £48.50, Bookings : 2, Discount : £19.40, Income :£77.60 Seat Type : 1ST, Seat Price : £44.50, Bookings : 3, Discount : £26.70, Income : £106.80 Seat Type : STD(Table), Seat Price : £28.50, Bookings : 3, Discount : £17.10, Income : £68.40 Seat Type : STD, Seat Price : £24.50, Bookings : 5, Discount : £24.50, Income : £98.00 Total Income : £350.80 Total Discount : £87.70
What I have tried:
package seatDiscountSystem;
import java.util.Scanner;
import java.io.File;
import java.io.FileNotFoundException;
public class SeatDiscountSystem {
public static void main(String[] args) {
// display header
System.out.println("-- Seat Discount System--");
String customDiscountChoice = "N";
double DEFAULT_DISCOUNT_RATE = 20.0;
Scanner scanner = new Scanner(System.in);
// get custom discount rate wish from user
System.out.print("\nSpecify Custom Discount Rate[Y|N]: ");
customDiscountChoice = scanner.nextLine();
// if custom discount rate wish 'Y'
if (customDiscountChoice.equalsIgnoreCase("y")) {
// get discount rate from user
System.out.print("Specify Discount Rate(%): ");
DEFAULT_DISCOUNT_RATE = scanner.nextDouble();
} else {
// if discount rate wish is 'N' display default discount rate
System.out.print("Assuming Discount Rate = " + DEFAULT_DISCOUNT_RATE + "%\n");
}
System.out.print("\n");
// call function to read and display the contents
readDispalyFileContents(DEFAULT_DISCOUNT_RATE);
// close the scanner instance
scanner.close();
}
public static void readDispalyFileContents(double discountRate) {
// variables to hold total income and discount
double totalIncome = 0;
double totalDiscount = 0;
// variable to display euro symbol
String euro = "\u20ac";
try {
// instantiate and open the file
Scanner scanner = new Scanner(new File("seats.txt"));
// read the file contents
while (scanner.hasNextLine()) {
// read contents line by line and store in appropriate variables
String seatType = scanner.nextLine();
double seatPrice = Double.parseDouble(scanner.nextLine());
int numberOfBookings = Integer.parseInt(scanner.nextLine());
// calculate discount price
double discountPrice = (seatPrice * numberOfBookings * discountRate) / 100;
// calculate income
double income = (seatPrice * numberOfBookings) - discountPrice;
// display the contents
System.out.printf("Seat Type:%s,Seat Price:%s%.2f,Bookings:%d,Discount:%s%.2f,Income:%s%.2f\n",
seatType, euro, seatPrice, numberOfBookings, euro, discountPrice, euro, income);
// add Income to the total income
totalIncome += income;
// add discount price to the total discount
totalDiscount += discountPrice;
}
// display the total income and discount
System.out.print("\n");
System.out.printf("Total Income: %s%.2f", euro, totalIncome);
System.out.printf("\nTotal Discount: %s%.2f", euro, totalDiscount);
scanner.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK