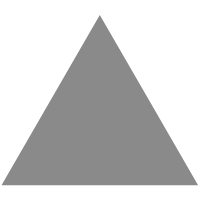
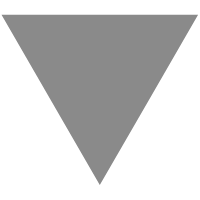
Different Ways to add CSS in React JS
source link: https://dev.to/salehmubashar/3-ways-to-add-css-in-react-js-336f
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
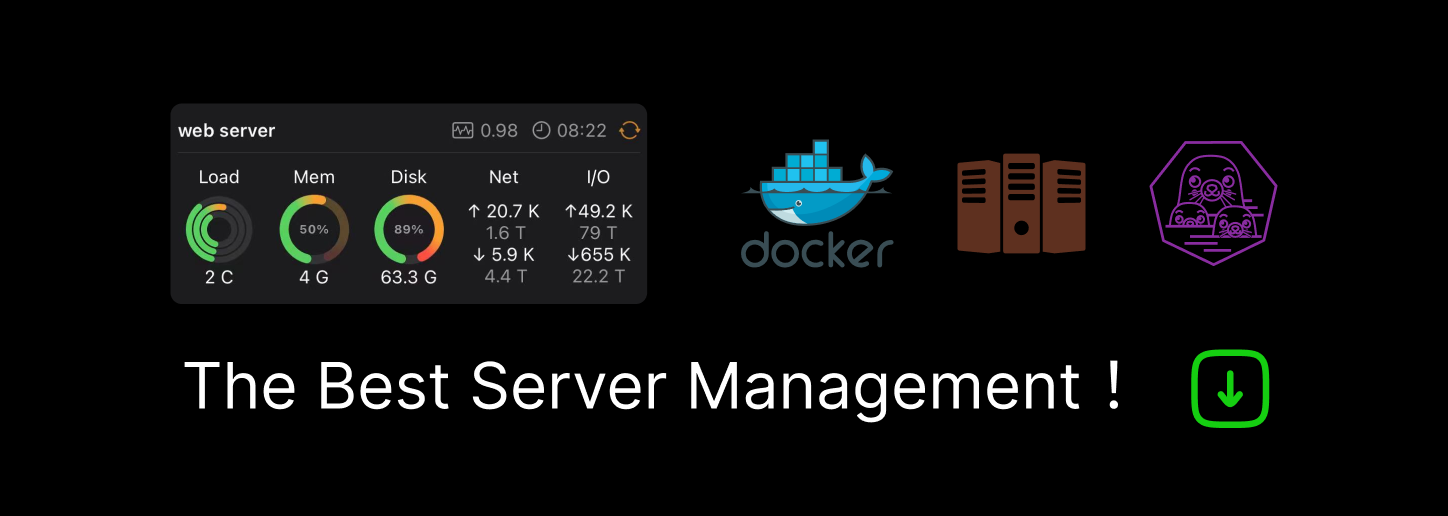
Hi guys!.
In this post, we will be looking into the best ways CSS code can be added in React JS or to you React App.
Obviously, CSS is crucial in making your app user friendly and visually attractive. These are the different ways to add CSS to your react app:
1 - External Stylesheet
You can create a new CSS file in your project directory and add your CSS inside it. You can then import it in your component, class or React JS page.
The following code is used to import an external CSS stylesheet.
import "./styles.css";
2 - Inline CSS
Probably the most common and quickest out of all 3 is inline CSS. However it has many disadvantages and it is generally discouraged to use unless it is a very small application.
Basically, we create an object that contains different references which are then called using the style{}
attribute.
For example, the CSS is added like this:
const styles = {
section: {
fontSize: "18px",
color: "#292b2c",
backgroundColor: "#fff",
padding: "0 20px"
},
wrapper: {
textAlign: "center",
margin: "0 auto",
marginTop: "50px"
}
}
It is then added to an element like this:
<section style={styles.section}>
<div style={styles.wrapper}>
</div>
</section>
3 - Styled Components
Probably the most powerful and useful in my opinion is Styled Components. Styled Components lets you write actual CSS in your JavaScript. The main advantage is that you can add conditional code and use variables and functions within the CSS!.
You can install Styled Components using the following command:
npm install --save styled-components
Next, you need to import it in you component. Then you can create a new variable that will contain the CSS. The same variable name with open and close brackets will render or create an HTML element with the previously added styles on it.
import styled from 'styled-components'
// Create a button variable and add CSS
const Button = styled.button`
background: transparent;
border-radius: 3px;
border: 2px solid red;
color:red;
`
//display the HTML
render(
<div>
<Button>Button</Button>
</div>
);
Other than these, there are 3 more useful ways you can add CSS (credit to lukeshiru):
4 - CSS Modules
You can also add scoped styles quite easily, you just need to create a file with the extension .module.css, like this:
// ComponentName.module.css
.Red {
color: #f00;
}
.Blue {
color: #00f;
}
Then you import it like this:
import styles from "./ComponentName.module.css";
and use it like this:
<span className={styles.Red}>This text will be red</span>
<span className={styles.Blue}>This text will be blue</span>
5 - Atomic CSS
One of the most popular atomic CSS frameworks out there is Tailwind, by just making it part of your project following their instructions you can just use classNames without even touching CSS.
<button className="font-bold bg-blue-600 px-6 py-3 text-white rounded-md">Blue button</button>
6 - Emotion
Styled-components is not the only library that allows you to create components with styles embeded on them, you have great alternatives out there such as Emotion. The best thing about Emotion is that it's framework agnostic, so you can take your knowledge to other libraries and frameworks besides React, while being pretty similar to styled-components (so you can in several scenarios just change the import).
And there you have it. I am sure there are many more out there but I think these options tick most of the boxes needed when adding CSS to your app.
Once again, thanks to lukeshiru for adding a few good alternatives.
Thanks for reading the post!.
Please check out my new tutorial.
And please support me on hubpages as well.
Until next time.
Cheers!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK