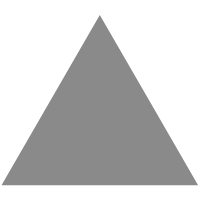
130
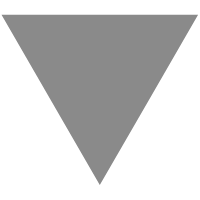
Blazor WebAssembly File Upload Using MudBlazor UI Components
source link: https://www.learmoreseekmore.com/2021/10/blazor-webassembly-fileupload-using-mudblazor-ui-components.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
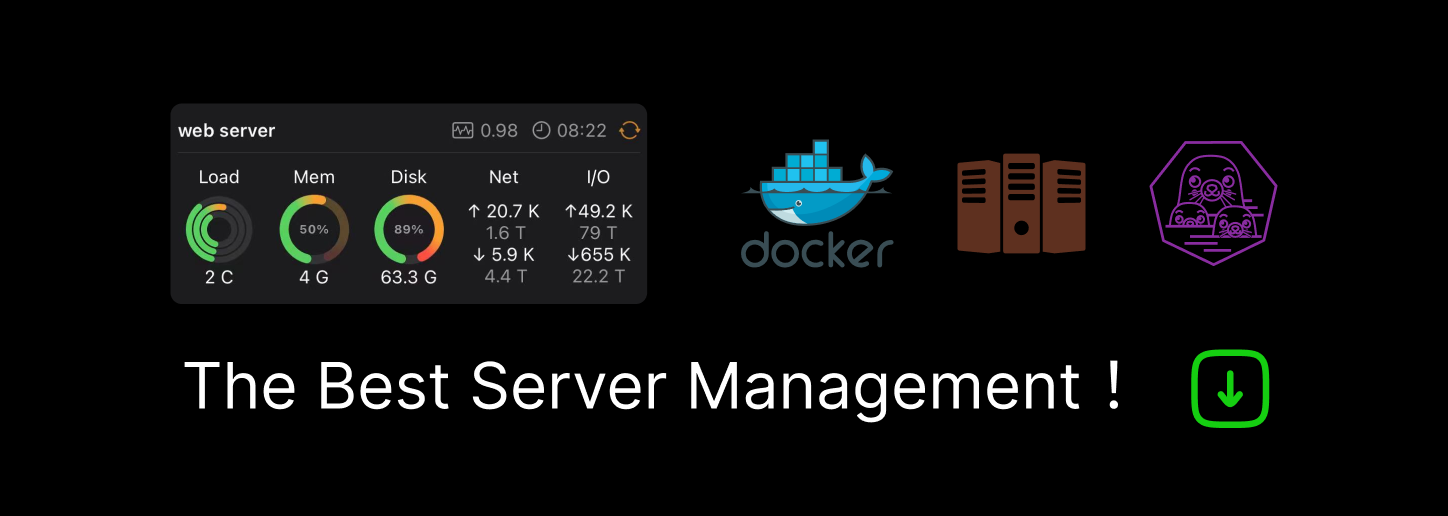
In this article, we are going to implement a Blazor WebAssembly application file upload using MudBlazor UI components.
Pages/Index.razor:(HTML Part)
Create A Sample Blazor WebAssembly Application:
Let's create a sample Blazor WebAssembly application to accomplish our demo on file uploading.
Initial MudBlazor Setup:
Install the 'MudBlazor' library package.
Add Mudblazor namespace into the '_Imports.razor'._Imports.razor:
@using MudBlazorAdd the below CSS files inside of the head tag in 'index.html'.
wwwroot/index.html:
<link href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700&display=swap" rel="stylesheet" /> <link href="_content/MudBlazor/MudBlazor.min.css" rel="stylesheet" />Now comment the 'bootstrap.min.css' and '{your_applicationname}.styles.css' links in the head tag.
Add MudBlazor javascript file in 'index.html' just above the closing body tag.
wwwroot/index.html:
<script src="_content/MudBlazor/MudBlazor.min.js"></script>Register MudBlazor service in 'Program.cs'.
Program.cs:
- builder.Services.AddMudServices();
Shared/MainLayout.razor:
- @inherits LayoutComponentBase
- <MudThemeProvider />
- <div class="page">
- <MudAppBar Color="Color.Info">
- <MudText Typo="Typo.h4">File Upload Demo</MudText>
- </MudAppBar>
- <div class="main mt-12" >
- <div class="content px-4">
- @Body
- </div>
- </div>
- </div>
Now our application looks as below.
MudBlazor To Compose File Upload:
Using HTML elements like 'label', 'input' we can create a file upload button.
The 'for' attribute value of the label must match with 'id' value of the 'input', we enable the 'input' to be triggered by clicking on the label. So the trick is to style the label to like like a button and hide the input.
Blazor WebAssembly from .Net 3.1 provides a default blazor component for file upload like 'InputFile'. So we can use 'InputFile' component instead of HTML 'input' filed.
We can make 'MudButton' component as a 'HTML' label element to do that 'MudButton' has an attribute like 'HtmlTag' and its value should be set like 'label'. By doing this HTML label getts rendered with all the beautiful styles of the MudBlazor button.
Implement File Upload Blazor Component Logic:
Let's first create the model class like 'SaveFile.cs' to capture the uploaded files.
Models/SaveFile.cs:
- using System.Collections.Generic;
- namespace Bwasm.MudFileUpload.UI.Models
- public class SaveFile
- public SaveFile()
- Files = new List<FileData>();
- public List<FileData> Files { get; set; }
- public class FileData
- public byte[] ImageBytes { get; set; }
- public string FileName { get; set; }
- public string FileType { get; set; }
- public long FileSize { get; set; }
Pages/Index.razor:(HTML Part)
- @page "/"
- <MudPaper Class="d-flex justify-center py-2 px-1 mt-6">
- <InputFile id="fileInput" OnChange="UploadFiles" hidden multiple />
- <MudButton HtmlTag="label"
- Variant="Variant.Filled"
- Color="Color.Success"
- StartIcon="@Icons.Filled.CloudUpload"
- for="fileInput">
- Upload Files
- </MudButton>
- </MudPaper>
- @if ((saveFile?.Files?.Count ?? 0) > 0)
- <MudPaper Class="d-flex justify-center py-2 px-1">
- @foreach (var item in saveFile?.Files)
- <MudPaper Class="pa-2 mx-2">
- <MudIconButton Color="Color.Error" Icon="@Icons.Material.Filled.Delete" OnClick="@(_ => RemoveImg(item))" aria-label="delete"></MudIconButton>
- <img width="150" height="150" src="@($"data:{item.FileType};base64,{Convert.ToBase64String(item.ImageBytes)}")">
- </MudPaper>
- </MudPaper>
- (Line: 3-10) Using 'InputField' and 'MudButton' we are creating the file upload UI. Here on 'InputFile' component we added attributes like 'hidden'(to hide the HTML), 'multiple' (enables multiple images to upload). On 'MudButton' we must define the 'HtmlTag' and its value should be 'label'. On 'MudButton' component 'for' attribute value must be match with the 'id' value of the 'InputFile' component. The 'InputFile' component registered 'OnChange' event with 'UpLoadFiles' method.
- (Line: 13-25) Preview section of the uploaded images.
- (Line: 19) Delete button to remove the selected image. Button registered with a 'RemoveImg' method.
- (Line: 21) Previewing the selected image.
- @code{
- SaveFile saveFile = new SaveFile();
- private async Task UploadFiles(InputFileChangeEventArgs e)
- foreach (var file in e.GetMultipleFiles())
- var fileData = new FileData();
- var buffers = new byte[file.Size];
- await file.OpenReadStream().ReadAsync(buffers);
- fileData.FileName = file.Name;
- fileData.FileSize = file.Size;
- fileData.FileType = file.ContentType;
- fileData.ImageBytes = buffers;
- saveFile.Files.Add(fileData);
- private void RemoveImg(FileData file)
- saveFile.Files.Remove(file);
- (Line: 2) Initialized the 'SaveFile' object to store the uploaded images.
- (Line: 3-17) The 'UploadFiles' method registered with the 'OnChange' event of the 'InputFile' component.
- (Line: 10) Fetching the image as bytes of data.
- (Line: 19-22) Remove the selected image from the preview.
Now run the application and check the output.
Create API Endpoint To Save Files To Physical Location:
Create another sample .NetCore Web API application. Then we will create an endpoint to save the files to a physical location on the server. This endpoint will be consumed by our Blazor Assembly application.
Now create a payload object for our endpoint as below.
Models/SaveFile.cs:
- using System.Collections.Generic;
- namespace Bwasm.MudFileUpload.API.Models
- public class SaveFile
- public SaveFile()
- Files = new List<FileData>();
- public List<FileData> Files { get; set; }
- public class FileData
- public byte[] ImageBytes { get; set; }
- public string FileName { get; set; }
- public string FileType { get; set; }
- public long FileSize { get; set; }
Controllers/FileUpLoadController.cs:
- using Bwasm.MudFileUpload.API.Models;
- using Microsoft.AspNetCore.Mvc;
- using System;
- using System.Threading.Tasks;
- namespace Bwasm.MudFileUpload.API.Controllers
- [Route("api/[controller]")]
- [ApiController]
- public class FileUploadController : ControllerBase
- [HttpPost]
- [Route("save-file-to-physicallocation")]
- public async Task<IActionResult> SaveToPhysicalLocation([FromBody] SaveFile saveFile)
- foreach (var file in saveFile.Files)
- string fileExtenstion = file.FileType.ToLower().Contains("png") ? "png" : "jpg";
- string fileName = $@"D:\MyTest\{Guid.NewGuid()}.{fileExtenstion}";
- using (var fileStream = System.IO.File.Create(fileName))
- await fileStream.WriteAsync(file.ImageBytes);
- return Ok();
- (Line: 16) Looping the collection of file information.
- (Line: 19) The full path of the file name. It is always recommended to save the files in non-application folder on the server.
- (Line: 20) The 'System.IO.File.Create("image_path")' generates the file stream at the specified path.
- (Line: 22) Saving the files array of byte data to the specified physical path.
Startup.cs:
- public void ConfigureServices(IServiceCollection services)
- services.AddCors(options =>
- options.AddPolicy(name: "MyAllowSpecificOrigins",
- builder =>
- builder.AllowAnyOrigin();
- builder.AllowAnyHeader();
- builder.AllowAnyMethod();
- public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
- app.UseCors("MyAllowSpecificOrigins");
Invoke API Endpoint From Blazor WebAssembly Application:
Add our web API domain to HttpClient configuration in Program.cs file.
Program.cs:
- builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri("https://localhost:6001/") });
Pages/Index.razor:(Html Part)
- @page "/"
- @inject HttpClient Http
- <MudPaper Class="d-flex justify-center py-2 px-1 mt-6">
- <InputFile id="fileInput" OnChange="UploadFiles" hidden multiple />
- <MudButton HtmlTag="label"
- Variant="Variant.Filled"
- Color="Color.Success"
- StartIcon="@Icons.Filled.CloudUpload"
- for="fileInput">
- Upload Files
- </MudButton>
- <MudButton OnClick="SaveToServer"
- Variant="Variant.Filled"
- Color="Color.Warning"
- StartIcon="@Icons.Filled.CloudUpload"
- for="fileInput">
- Upload Files To Server
- </MudButton>
- </MudPaper>
- (Line: 2) Inject the 'HttpClient'.
- (Line: 13-19) Add new button that will invoke the API call for uploading the image to the server. Here click event registered with 'SaveToServer' method.
- private async Task SaveToServer()
- await Http.PostAsJsonAsync("/api/FileUpload/save-file-to-physicallocation", saveFile);
Support Me!
Buy Me A Coffee
PayPal Me
Video Session:
Wrapping Up:
Hopefully, I think this article delivered some useful information on the Blazor WebAssembly File Upload using MudBalzor UI Components. I love to have your feedback, suggestions, and better techniques in the comment section below.
Follow Me:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK