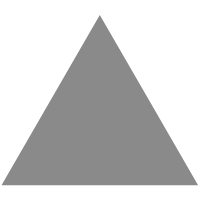
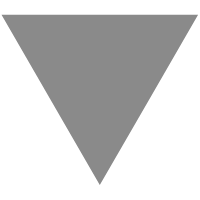
HashSet in std::collections::hash_set - Rust
source link: https://doc.rust-lang.org/stable/std/collections/hash_set/struct.HashSet.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Struct std::collections::hash_set::HashSet1.0.0[−][src]
pub struct HashSet<T, S = RandomState> { /* fields omitted */ }
A hash set implemented as a HashMap
where the value is ()
.
As with the HashMap
type, a HashSet
requires that the elements
implement the Eq
and Hash
traits. This can frequently be achieved by
using #[derive(PartialEq, Eq, Hash)]
. If you implement these yourself,
it is important that the following property holds:
k1 == k2 -> hash(k1) == hash(k2)
In other words, if two keys are equal, their hashes must be equal.
It is a logic error for an item to be modified in such a way that the
item’s hash, as determined by the Hash
trait, or its equality, as
determined by the Eq
trait, changes while it is in the set. This is
normally only possible through Cell
, RefCell
, global state, I/O, or
unsafe code. The behavior resulting from such a logic error is not
specified, but will not result in undefined behavior. This could include
panics, incorrect results, aborts, memory leaks, and non-termination.
Examples
use std::collections::HashSet;
// Type inference lets us omit an explicit type signature (which
// would be `HashSet<String>` in this example).
let mut books = HashSet::new();
// Add some books.
books.insert("A Dance With Dragons".to_string());
books.insert("To Kill a Mockingbird".to_string());
books.insert("The Odyssey".to_string());
books.insert("The Great Gatsby".to_string());
// Check for a specific one.
if !books.contains("The Winds of Winter") {
println!("We have {} books, but The Winds of Winter ain't one.",
books.len());
}
// Remove a book.
books.remove("The Odyssey");
// Iterate over everything.
for book in &books {
println!("{}", book);
}
RunThe easiest way to use HashSet
with a custom type is to derive
Eq
and Hash
. We must also derive PartialEq
, this will in the
future be implied by Eq
.
use std::collections::HashSet;
#[derive(Hash, Eq, PartialEq, Debug)]
struct Viking {
name: String,
power: usize,
}
let mut vikings = HashSet::new();
vikings.insert(Viking { name: "Einar".to_string(), power: 9 });
vikings.insert(Viking { name: "Einar".to_string(), power: 9 });
vikings.insert(Viking { name: "Olaf".to_string(), power: 4 });
vikings.insert(Viking { name: "Harald".to_string(), power: 8 });
// Use derived implementation to print the vikings.
for x in &vikings {
println!("{:?}", x);
}
RunA HashSet
with a known list of items can be initialized from an array:
use std::collections::HashSet;
let viking_names = HashSet::from(["Einar", "Olaf", "Harald"]);
RunRecommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK