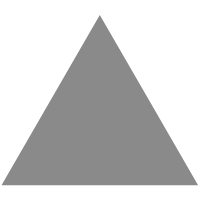
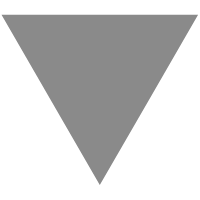
Spring Cloud Feign如何实现JWT令牌中继,传递认证信息?
source link: https://blog.didispace.com/spring-cloud-feign-transmit-jwt/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
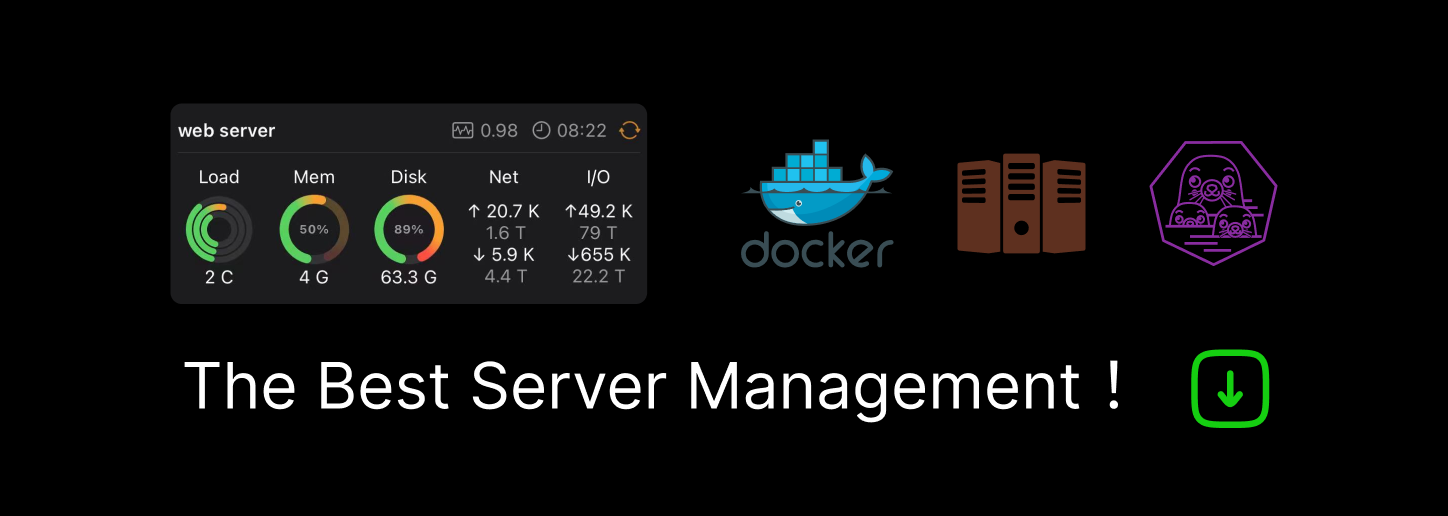
Spring Cloud Feign如何实现JWT令牌中继,传递认证信息?
在上一篇实现了Spring Cloud资源服务器的定制化,但是又发现了一个新的问题,Spring Cloud微服务调用的过程中需要令牌中继。只有令牌中继才能在调用链中保证用户认证信息的传递。今天就来分享一下如何在Feign中实现令牌中继。
令牌中继(Token Relay)是比较正式的说法,说白了就是让Token令牌在服务间传递下去以保证资源服务器能够正确地对调用方进行鉴权。
令牌难道不能在Feign自动中继吗?
如果我们携带Token去访问A服务,A服务肯定能够鉴权,但是A服务又通过Feign调用B服务,这时候A的令牌是无法直接传递给B服务的。
这里来简单说下原因,服务间的调用通过Feign接口来进行。在调用方通常我们编写类似下面的Feign接口:
@FeignClient(name = "foo-service",fallback = FooClient.Fallback.class)
public interface FooClient {
@GetMapping("/foo/bar")
Rest<Map<String, String>> bar();
@Component
class Fallback implements FooClient {
@Override
public Rest<Map<String, String>> bar() {
return RestBody.fallback();
}
}
}
当我们调用Feign接口后,会通过动态代理来生成该接口的代理类供我们调用。如果我们不打开熔断我们可以从Spring Security提供SecurityContext对象中提取到资源服务器的认证对象JwtAuthenticationToken,它包含了JWT令牌然后我们可以通过实现Feign的拦截器接口RequestInterceptor把Token放在请求头中,伪代码如下:
/**
* 需要注入Spring IoC
**/
static class BearerTokenRequestInterceptor implements RequestInterceptor {
@Override
public void apply(RequestTemplate template) {
final String authorization = HttpHeaders.AUTHORIZATION;
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
if (authentication instanceof JwtAuthenticationToken){
JwtAuthenticationToken jwtAuthenticationToken = (JwtAuthenticationToken) authentication;
String tokenValue = jwtAuthenticationToken.getToken().getTokenValue();
template.header(authorization,"Bearer "+tokenValue);
}
}
}
如果我们不开启熔断这样搞问题不大,为了防止调用链雪崩服务熔断基本没有不打开的。这时候从SecurityContextHolder就无法获取到Authentication了。因为这时Feign调用是在调用方的调用线程下又开启了一个子线程中进行的。由于我使用的熔断组件是Resilience4J,对应的线程源码在Resilience4JCircuitBreaker中:
Supplier<Future<T>> futureSupplier = () -> executorService.submit(toRun::get);
SecurityContextHolder保存信息是默认是通过ThreadLocal实现的,我们都知道这个是不能跨线程的,而Feign的拦截器这时恰恰在子线程中,因此开启了熔断功能(circuitBreaker)的Feign无法直接进行令牌中继。
熔断组件有过时的Hystrix、Resilience4J、还有阿里的哨兵Sentinel,它们的机制可能有小小的不同。
实现令牌中继
虽然直接不能实现令牌中继,但是我从中还是找到了一些信息。在Feign接口代理的处理器FeignCircuitBreakerInvocationHandler中发现了下面的代码:
private Supplier<Object> asSupplier(final Method method, final Object[] args) {
final RequestAttributes requestAttributes = RequestContextHolder.getRequestAttributes();
return () -> {
try {
RequestContextHolder.setRequestAttributes(requestAttributes);
return this.dispatch.get(method).invoke(args);
}
catch (RuntimeException throwable) {
throw throwable;
}
catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
finally {
RequestContextHolder.resetRequestAttributes();
}
};
}
这是Feign代理类的执行代码,我们可以看到在执行前:
final RequestAttributes requestAttributes = RequestContextHolder.getRequestAttributes();
这里是获得调用线程中请求的信息,包含了ServletHttpRequest、ServletHttpResponse等信息。紧接着又在lambda代码中把这些信息又Setter了进去:
RequestContextHolder.setRequestAttributes(requestAttributes);
如果这是一个线程中进行的简直就是吃饱了撑的,事实上Supplier返回值是在另一个线程中执行的。这样做的目的就是为了跨线程保存一些请求的元数据。
InheritableThreadLocal
RequestContextHolder 是如何做到跨线程了传递数据的呢?
public abstract class RequestContextHolder {
private static final ThreadLocal<RequestAttributes> requestAttributesHolder =
new NamedThreadLocal<>("Request attributes");
private static final ThreadLocal<RequestAttributes> inheritableRequestAttributesHolder =
new NamedInheritableThreadLocal<>("Request context");
// 省略
}
RequestContextHolder 维护了两个容器,一个是不能跨线程的ThreadLocal,一个是实现了InheritableThreadLocal的NamedInheritableThreadLocal。InheritableThreadLocal是可以把父线程的数据传递到子线程的,基于这个原理RequestContextHolder把调用方的请求信息带进了子线程,借助于这个原理就能实现令牌中继了。
实现令牌中继
把最开始的Feign拦截器代码改动了一下就实现了令牌的中继:
/**
* 令牌中继
*/
static class BearerTokenRequestInterceptor implements RequestInterceptor {
private static final Pattern BEARER_TOKEN_HEADER_PATTERN = Pattern.compile("^Bearer (?<token>[a-zA-Z0-9-._~+/]+=*)$",
Pattern.CASE_INSENSITIVE);
@Override
public void apply(RequestTemplate template) {
final String authorization = HttpHeaders.AUTHORIZATION;
ServletRequestAttributes requestAttributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
if (Objects.nonNull(requestAttributes)) {
String authorizationHeader = requestAttributes.getRequest().getHeader(HttpHeaders.AUTHORIZATION);
Matcher matcher = BEARER_TOKEN_HEADER_PATTERN.matcher(authorizationHeader);
if (matcher.matches()) {
// 清除token头 避免传染
template.header(authorization);
template.header(authorization, authorizationHeader);
}
}
}
}
这样当你调用FooClient.bar()时,在foo-service中资源服务器(OAuth2 Resource Server)也可以获得调用方的令牌,进而获得用户的信息来处理资源权限和业务。
❝
不要忘记将这个拦截器注入Spring IoC。
微服务令牌中继是非常重要的,保证了用户状态在调用链路的传递。而且这也是微服务的难点。今天借助于Feign的一些特性和ThreadLocal的特性实现了令牌中继供大家参考。原创不易,请大家多多点击再看、点赞、转发。
好了,今天的学习就到这里!如果您学习过程中如遇困难?可以加入我们超高质量的Spring技术交流群,参与交流与讨论,更好的学习与进步!更多Spring Cloud教程可以点击直达!,欢迎收藏与转发支持!
原文链接:https://mp.weixin.qq.com/s/F8-KLy-uKUwQZJgbvy00RQ
版权归作者所有,转载请注明作者、原文、译者等出处信息
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK