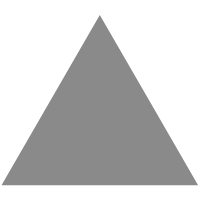
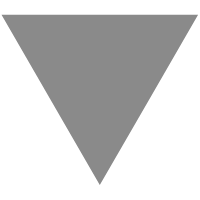
Creating User Interfaces in Python using PySimpleGUI
source link: https://www.codeproject.com/Articles/5315989/Creating-User-Interfaces-in-Python-using-PySimpleG
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
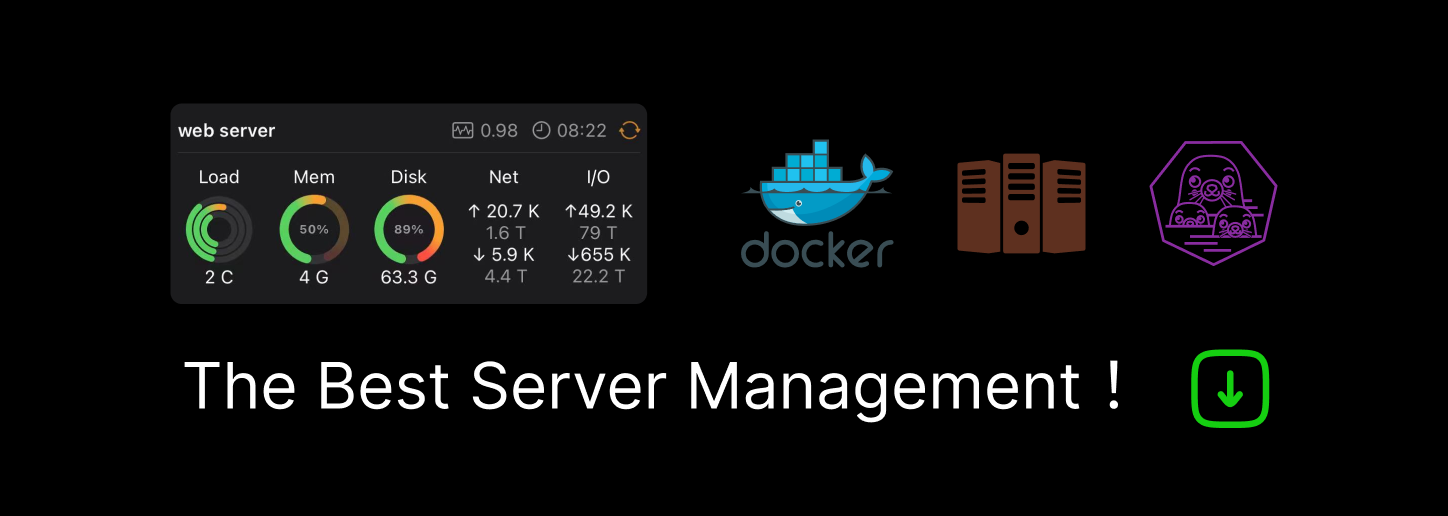
Introduction
PySimpleGUI is a Python package which can be used to develop user-interfaces with the simplest code. In spite of being simple it can be used to create complex and sophisticated user-interfaces. Any programmer, even if he is new to Python can easily learn PySimpleGUI and start creating Python applications with good looking user-interfaces within minutes.
Background
PySimpleGUI currently supports 4 GUI frameworks:
- PySimpleGUI
- PySimpleGUIQt
- PySimpleGUIWx
- PySimpleGUIWeb
Porting GUI from one framework to another can be done with very few changes. Some features may be supported by one framework but not by others, but overall, porting from one framework to another is easy with minimal changes in code. The framework to be used can be specified using the import
statement. For example, to use PySimpleGUI, we can write import PySimpleGUI as sg
and to use PySimpleGUIQt, we can write import PySimpleGUIQt as sg a
nd to use PySimpleGUIWeb, we can write import PySimpleGUIWeb as sg.
Using the code
Like other packages, PySimpleGUI can be installed using the pip command as follows:
pip install pysimplegui --user
To install PySimpleGUIWeb, the following command can be used:
pip install pysimpleguiweb --user
Layouts:
A layout is defined in PySimpleGUI using a List of Lists. Each list inside the main list defines one row. Each row consists of user-interface elements which are rendered on a single line.
Following are some of the commonly required user-interface elements than can be created in PySimpleGUI.
- sg.Text: To render constant text.
- sg.InputText: To accept input at runtime.
- sg.Checkbox: To display a checkbox.
- sg.Radio: To display a radio button.
- sg.Combo: To display a list of items as a combobox from which only one element can be selected.
- sg.Listbox: To display a list of items as a listbox. Setting select_mode="multiple" attributeallows selecting multiple elements.
For example the following code can be used to render a typical user-interface:
layout = [ [sg.Text("Select a theme: ",size=(15,1)),sg.Combo(sg.theme_list(),key="t",default_value="BlueMono",size=(10,1))], [sg.Text("Enter your name: ",size=(15,1)),sg.InputText(key="n",size=(10,1))], [sg.Checkbox("Capitalize",key="c",size=(15,1))], [sg.Text("Choose your gender: ",size=(15,1)),sg.Radio("Male","g",True,key="g1"),sg.Radio("Female","g",key="g2")], [sg.Text("Choose your qualification: ",size=(15,1)),sg.Combo(qualifications_list,key="q",default_value="Graduate",size=(15,3))], [sg.Text("Choose your hobbies: ",size=(15,1)),sg.Listbox(hobbies_list,key="h",select_mode="multiple",size=(15,3))], [sg.Button("Show",size=(15,1)),sg.Button("Reset",size=(15,1))] ] window = sg.Window("Controls",layout)
The first row in the above layout consists of a combo box containing all available themes, obtained using the sg.theme_list() function. Other rows define other user interface elements. The sg.Window is used to create a window with the title as "Controls" and having the specified layout. Its output is as follows:
Event Handling:
An event loop is required to process events and read the inputs entered at runtime. The event loop can be created as follows:
while True: event,values = window.read() if event == sg.WINDOW_CLOSED: break if event == "Show": name = values["n"].upper() if values["c"] else values["n"] gender = "Male" if values["g1"] else "Female" qualification = values["q"] hobbies = "" for h in values["h"]: hobbies = hobbies + h + "," hobbies = hobbies.rstrip(",") details = "Name: " + name + "\n" details = details + "Gender: " + gender + "\n" details = details + "Qualification: " + qualification + "\n" details = details + "Hobbies: " + hobbies + "\n" sg.theme(values["t"]) sg.popup(details,title="Details") if event == "Reset": window["n"].update("") window["c"].update(False) window["g1"].update(True) window["q"].update("Graduate") window["h"].update(hobbies_list) window.close()
In the above code, the information about the button clicked or wiindow closed events and the data entered are obtained using the window.read() function. If the window close button is clicked, the sg.WINDOW_CLOSED event is fired, which causes the control to break out of the event loop and the window is closed using the close() function. If the "Show" button is clicked, the "Show" event is fired, in which all data entered on the form is collected using the values dictionary and dispalyed in a popup window using the popup() function. If the "Reset" button is clicked, the "Reset" event is fired, in which all controls are reset to their default values using the update() function. In the "Show" event, the currently selected theme is applied using the sg.theme() function.
Themes: Themes can be used in PySimpleGUI to display colorful and more beautiful windows using the shortest possible code. The following line of code, creates a combo box filled with all available themes.
sg.Combo(sg.theme_list(),key="t",default_value="BlueMono",size=(10,1))
To apply the selected theme from the combo box, the following code can be used.
sg.theme(values["t"])
All available themes can be previewed using the sg.theme_previewer() function as follows.
import PySimpleGUI as sg sg.theme_previewer()
The above code produces the following output.
Points of Interest
I hope the above discussion is helpful to readers and leads more programmers to use PySimpleGUI.
Recommend
-
469
readme.md
-
62
【51CTO.com快译】很少有人通过像双击.exe文件那样双击.py文件来运行Python程序。普通用户(非程序员)双击.exe文件时,认为应该弹出可与之交互...
-
50
-
12
Creating a simple graphical user interface (GUI) that works across multiple platforms can be complicated. But it doesn’t have to be that way. You can use Python and the PySimpleGUI package to create nice-looking user interfaces that you and...
-
6
Simplify Python GUI Development With PySimpleGUI Creating a simple graphical user interface (GUI) that works across multiple platforms can be complicated. But it doesn’t have to be that way. You can use Python and the PySimpleGUI pa...
-
9
Python:一个简单易用的 Python GUI 图形化界面编程框架——PySimpleGUI By: taho On: 2021年7...
-
2
This Wednesday, 1 August, I did a brief presentation on GUI programming on Python with Tkinter, at Blip.PT, during a Summer Talks event promoted together by
-
6
Welcome to Enaml Enaml is a programming language and framework for creating professional-quality user interfaces with minimal effort. What you get A declarative programming language, with a Pythonic flavour.
-
3
-
6
PySimpleGUI是一个简单易用的Python GUI库,它提供了一种直观且快速创建图形用户界面的方式。 pip install PySimpleGUI
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK