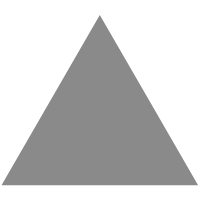
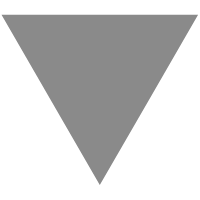
Java Math.pow() Method with Examples
source link: https://codeahoy.com/java/Math-Pow-method-JI_11/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
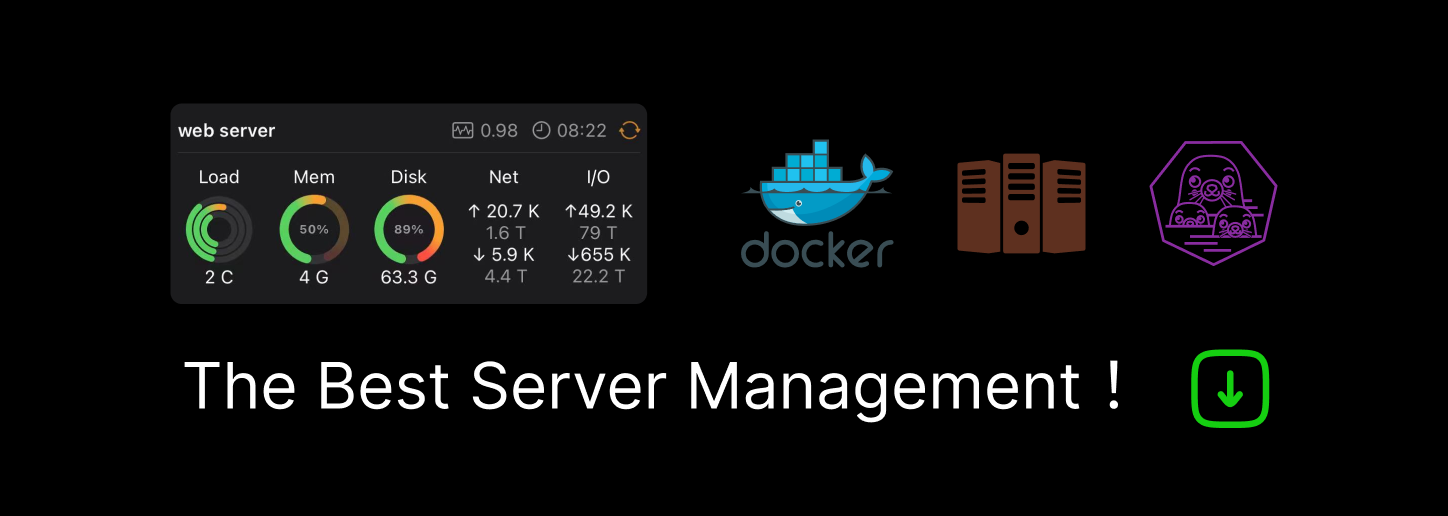
Java Math.pow() Method with Examples
Oct 26, 2016 · 3 mins read
The Math.pow() method is used for calculating a number raised to the power of another number. In other words, it’s used for calculating the power of a base. It is a static method that accepts two arguments. It returns the power of the first parameter, raised to the second parameter.
static double Math.pow(double a, double b)
Here, the first parameter, a is the base. The second parameter, b is the exponent. This method returns a^b or a raised to the power b.
Special cases
Here are some special cases that you need to aware of:
- If the second argument is positive or negative zero, then the result is 1.0.
- If the second argument is 1.0, then the result is the same as the first argument.
- If the second argument is NaN, then the result is NaN.
- If the first argument is NaN and the second argument is nonzero, then the result is NaN.
- If
- the absolute value of the first argument is greater than 1 and the second argument is positive infinity, or
- the absolute value of the first argument is less than 1 and the second argument is negative infinity,then the result is positive infinity.
Both arguments to Math.pow(…) method are of type double
. The return type is also double
. You can cast it to long
or int
(be careful because it might overflow.) Let’s raise 3 to the power of 9 using this function and cast the result to integer, that is,
int result = (int)Math.pow(3, 2) // result = 9
Code Example
import static java.lang.Double.NaN;
public class Main {
public static void main(String[] args) {
System.out.println((long) Math.pow(2, 4)); // 16
System.out.println((long) Math.pow(2, 1)); // 2
System.out.println((long) Math.pow(2, 0)); // 1
// If the second argument is NaN, then the result is NaN.
System.out.println(Math.pow(2, NaN)); // 0
System.out.println(Math.pow(2.5, 3)); // 15.625
}
}
Output
16
2
1
NaN
15.625
There you have it. Hope you found this tutorial useful!
See also
It’s worth noting that the Math
class in Java contains several useful methods for arithmetic, logarithms and trigonometric operations. The following YouTube video below talks about several methods of the Math
class with examples. It’s a short video and I recommend that you watch it.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK