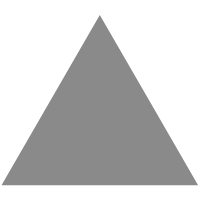
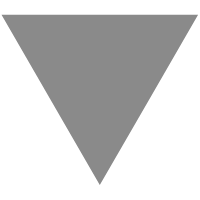
Getting Started With Django web framework
source link: https://blog.knoldus.com/django-web-framework/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
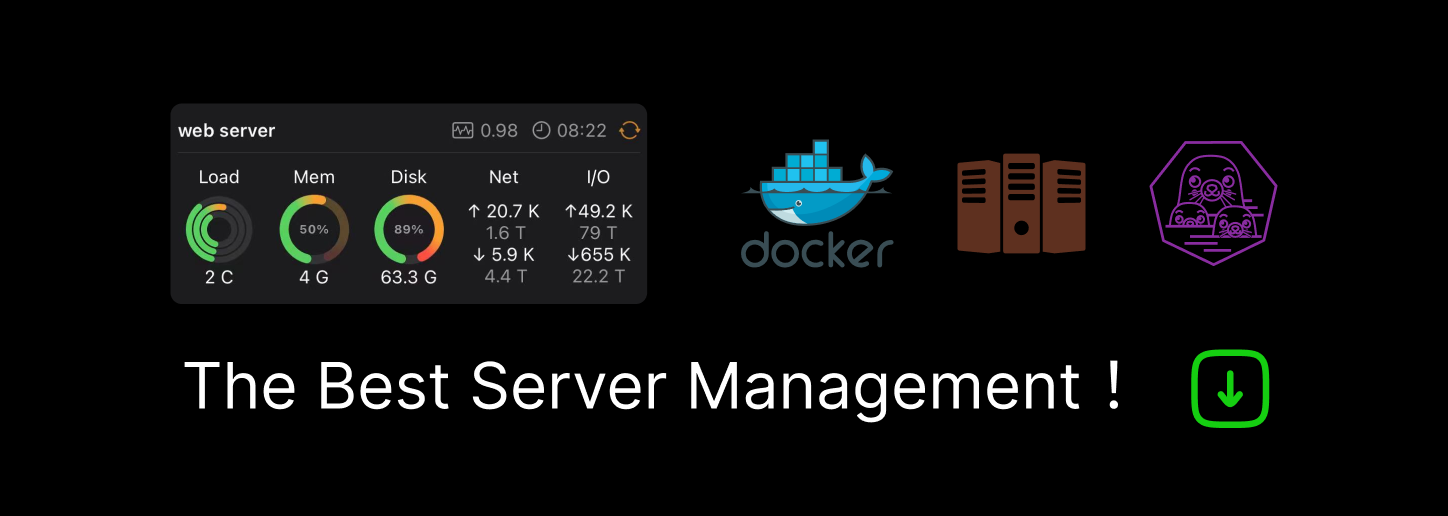
What is Django?
Django web framework is a full-fledged python based web framework that can be used to build complex and highly scalable web apps. It closely follows the MVC(Model, View, Controller) pattern of software development i.e., MVT(Model, View, Template) where the template is equivalent to the controller in the MVC pattern.
- Model defines the data structure. This is the base layer to an application and usually a database.
- View displays the content in the form of HTML and CSS.
- Template is a reusable part which handles the interaction between database and views.
Why Django?
- Pre Built-Packages
We don’t need to code the basic stuff, Django comes pre-packaged. It includes powerful ORM, easy database migrations, has built-in session handling, also handles URL Routing for you along with dynamic URLs
- Admin panel
Django has a customizable admin interface, so you can manage data in your database using basic CRUD operations. The admin panel can also be configured to have customized filters.
- Template System
Django has its own in-built templating system also known as Django template language (DTL). Django’s core template design principles and its design pattern that stands for Don’t-Repeat-Yourself (DRY).
- Security
We do not have to implement security features manually to keep web development going. The framework gives us protection against XSS and CSRF attacks, clickjacking, SQL injections, etc.
- Scalable and Reliable
Django gives us the functionality that enables us to create a small project and scale it as per our needs. Some of the most popular web applications like Instagram, Spotify, Reddit use Django web framework as a backend framework.
- Community
Django has excellent community support from all around the world which makes the framework even better.
Setting up our Development Environment
Pre-requisites
- Any Code Editor like VScode, Sublime, Pycharm etc.
- Python must be installed on the system.
- Basic understanding of python and its oops concepts.
When starting a new web development project, it’s a good idea to first set up your development environment. Create a new directory or folder for your application –
STEP 1:
Open the folder created in the terminal(for linux) and cmd(for windows)
STEP 2:
Create a virtual environment
python3 -m venv venv
STEP 3:
Activate your virtual by using –
source venv/bin/activate
STEP 4:
Installing Django
pip install django
Create a Django Project
A Django app is made up of a project which consists of its constituent apps. Make sure you are in the directory that we have created previously and our virtual environment is activated then run the following command to create a new Django project –
django-admin startproject demo
The above command creates a folder with name demo and when we cd inside the demo folder we have a directory structure like
main_directory/
│
├── demo/
│ ├── demo/
│ │ ├── __init__.py
│ │ ├── settings.py
│ │ ├── urls.py
│ │ └── wsgi.py
│ │
│ └── manage.py
│
└── venv/
Once we have set up our Django project and have the directory structure as above. Run the following command to check if everything is working fine. Make sure you are inside the demo folder in the first level.
python manage.py runserver
You will get a server address 127.0.0.1:8000 and when we open it on our browser it will open like this –
Creating an app inside our django project
After setting the project we will be doing all our inside the first level demo folder. To create a new app inside our Django project run the following command
python manage.py startapp home
The above command will create a folder named home with the following directory structure –
main_directory/
│
├── demo/
│ ├──demo/
│ ├── home/
│ │ ├── __init__.py
│ │ ├── admin.py
│ │ ├── apps.py
│ │ ├── models.py
│ │ ├── tests.py
│ │ └── views.py
│ │
│ └── manage.py
│
└── venv/
Files created inside the home folder :
- __init__.py tells Python to treat the directory as a Python package.
- admin.py contains all the necessary settings for the Django admin pages.
- apps.py contains settings for the application configuration.
- models.py contains a series of classes that will be resembles into database tables.
- tests.py contains classes for testing.
- views.py contains functions and classes that handle what to be displayed in the templates.
After this, we have to tell Django that we have created a new app with the name of home and we want to use that by making changes in the settings.py file
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'home',
]
Create a new file named urls.py inside the home folder to handle the path for our home app. And now the directory structure will look like –
main_directory/
│
├── demo/
│ ├──demo/
│ ├── home/
│ │ ├── __init__.py
│ │ ├── admin.py
│ │ ├── apps.py
│ │ ├── models.py
│ │ ├── tests.py
│ │ ├── urls.py
│ │ └── views.py
│ │
│ └── manage.py
│
└── venv/
Now we will update urls.py inside our demo folder to connect to the urls.py of our home app
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('home.urls')),
]
Next, we will write some code inside our views.py of our home app
from django.shortcuts import HttpResponse
def main(request):
return HttpResponse("<h1>Hello World</h1>")
Next, we will write some code inside our urls.py of our home app
from django.urls import path
from . import views
urlpatterns = [
path('', views.main, name='main'),
]
Now we will see the following screen on refreshing our page at 127.0.0.1:8000
Congratulations, You’ve created your first Django app and hooked it up to your project.
Please refer to the https://github.com/knolderdev/Knolsharing-assign/tree/master/demo
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK