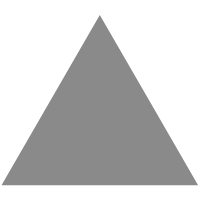
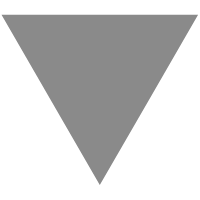
Awesome Redux cheatsheet
source link: https://devhints.io/awesome-redux
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
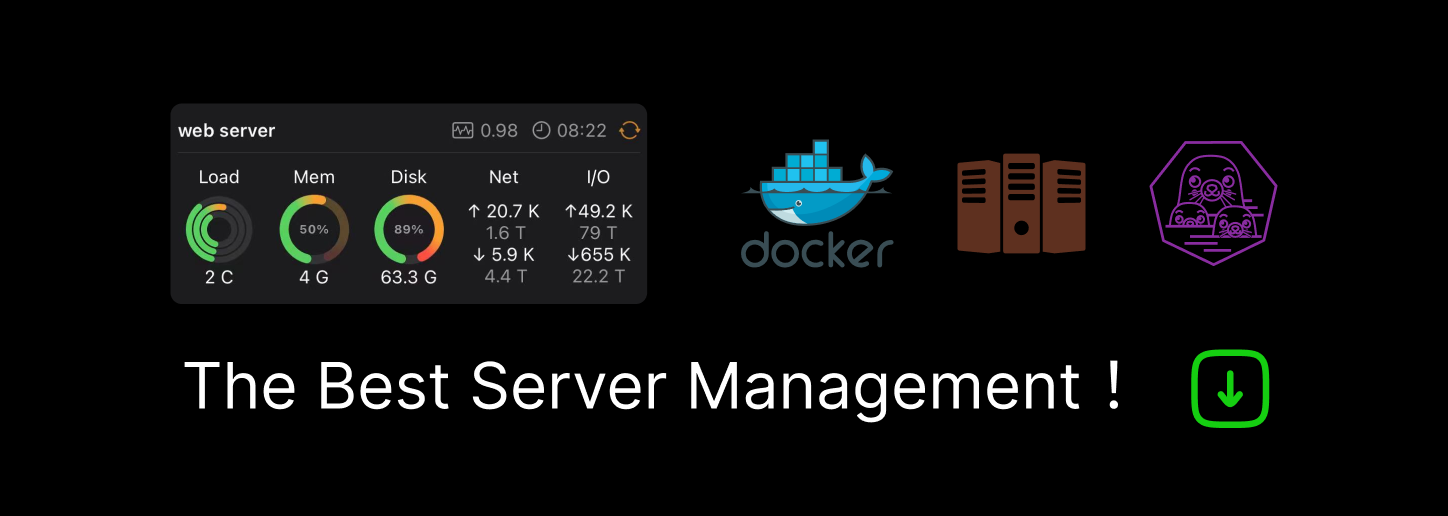
redux-actions
Create action creators in flux standard action format.
increment = createAction('INCREMENT', amount => amount)
increment = createAction('INCREMENT') // same
increment(42) === { type: 'INCREMENT', payload: 42 }
// Errors are handled for you:
err = new Error()
increment(err) === { type: 'INCREMENT', payload: err, error: true }
flux-standard-action
A standard for flux action objects. An action may have an error
, payload
and meta
and nothing else.
{ type: 'ADD_TODO', payload: { text: 'Work it' } }
{ type: 'ADD_TODO', payload: new Error(), error: true }
redux-multi
Dispatch multiple actions in one action creator.
store.dispatch([
{ type: 'INCREMENT', payload: 2 },
{ type: 'INCREMENT', payload: 3 }
])
reduce-reducers
Combines reducers (like combineReducers()), but without namespacing magic.
re = reduceReducers(
(state, action) => state + action.number,
(state, action) => state + action.number
)
re(10, { number: 2 }) //=> 14
redux-logger
#Async
redux-promise
Pass promises to actions. Dispatches a flux-standard-action.
increment = createAction('INCREMENT') // redux-actions
increment(Promise.resolve(42))
redux-promises
Sorta like that, too. Works by letting you pass thunks (functions) to dispatch()
. Also has ‘idle checking’.
fetchData = (url) => (dispatch) => {
dispatch({ type: 'FETCH_REQUEST' })
fetch(url)
.then((data) => dispatch({ type: 'FETCH_DONE', data })
.catch((error) => dispatch({ type: 'FETCH_ERROR', error })
})
store.dispatch(fetchData('/posts'))
// That's actually shorthand for:
fetchData('/posts')(store.dispatch)
redux-effects
Pass side effects declaratively to keep your actions pure.
{
type: 'EFFECT_COMPOSE',
payload: {
type: 'FETCH'
payload: {url: '/some/thing', method: 'GET'}
},
meta: {
steps: [ [success, failure] ]
}
}
redux-thunk
Pass “thunks” to as actions. Extremely similar to redux-promises, but has support for getState.
fetchData = (url) => (dispatch, getState) => {
dispatch({ type: 'FETCH_REQUEST' })
fetch(url)
.then((data) => dispatch({ type: 'FETCH_DONE', data })
.catch((error) => dispatch({ type: 'FETCH_ERROR', error })
})
store.dispatch(fetchData('/posts'))
// That's actually shorthand for:
fetchData('/posts')(store.dispatch, store.getState)
// Optional: since fetchData returns a promise, it can be chained
// for server-side rendering
store.dispatch(fetchPosts()).then(() => {
ReactDOMServer.renderToString(<MyApp store={store} />)
})
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK