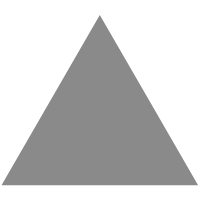
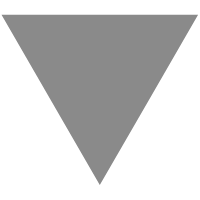
【DB系列】Mybatis之参数传递的几种姿势
source link: https://spring.hhui.top/spring-blog/2021/09/24/210924-SpringBoot%E7%B3%BB%E5%88%97Mybatis%E4%B9%8B%E5%8F%82%E6%95%B0%E4%BC%A0%E9%80%92%E7%9A%84%E5%87%A0%E7%A7%8D%E5%A7%BF%E5%8A%BF/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
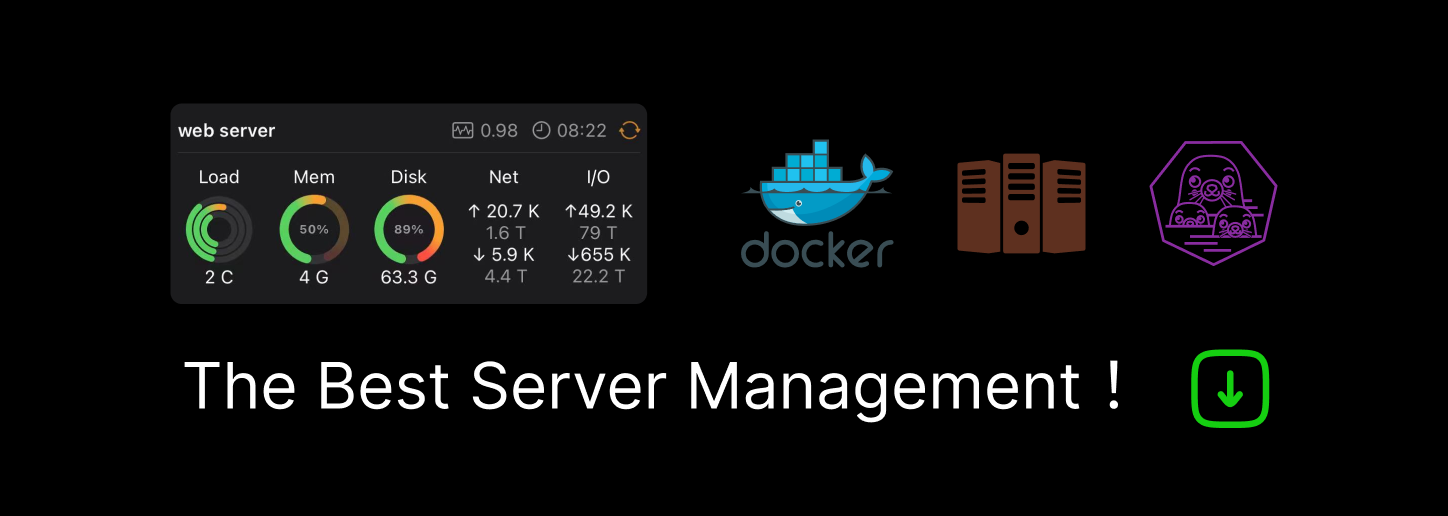
在mybatis的日常开发中,mapper接口中定义的参数如何与xml中的参数进行映射呢?除了我们常用的@Param
注解之外,其他的方式是怎样的呢?
I. 环境配置
我们使用SpringBoot + Mybatis + MySql来搭建实例demo
- springboot: 2.2.0.RELEASE
- mysql: 5.7.22
1. 项目配置
<dependencies>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
</dependencies>
核心的依赖mybatis-spring-boot-starter
,至于版本选择,到mvn仓库中,找最新的
另外一个不可获取的就是db配置信息,appliaction.yml
spring:
datasource:
url: jdbc:mysql://127.0.0.1:3306/story?useUnicode=true&characterEncoding=UTF-8&useSSL=false&serverTimezone=Asia/Shanghai
username: root
password:
2. 数据库表
用于测试的数据库
CREATE TABLE `money` (
`id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(20) NOT NULL DEFAULT '' COMMENT '用户名',
`money` int(26) NOT NULL DEFAULT '0' COMMENT '钱',
`is_deleted` tinyint(1) NOT NULL DEFAULT '0',
`create_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP COMMENT '创建时间',
`update_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP COMMENT '更新时间',
PRIMARY KEY (`id`),
KEY `name` (`name`)
) ENGINE=InnoDB AUTO_INCREMENT=551 DEFAULT CHARSET=utf8mb4;
II. 参数传递
接下来我们看一下Mapper接口中的参数与xml文件中的参数映射的几种姿势;关于mybatis项目的搭建,这里就略过,重点信息有下面几个
数据库实体对象
@Data
public class MoneyPo {
private Integer id;
private String name;
private Long money;
private Integer isDeleted;
private Timestamp createAt;
private Timestamp updateAt;
private Integer cnt;
}
mapper接口
@Mapper
public interface MoneyMapper {
}
xml文件,在资源文件夹下,目录层级与mapper接口的包路径完全一致(遵循默认的Mapper接口与xml文件绑定关系,详情查看SpringBoot系列Mybatis之Mapper接口与Sql绑定几种姿势)
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.git.hui.boot.mybatis.mapper.MoneyMapper">
<resultMap id="BaseResultMap" type="com.git.hui.boot.mybatis.entity.MoneyPo">
<id column="id" property="id" jdbcType="INTEGER"/>
<result column="name" property="name" jdbcType="VARCHAR"/>
<result column="money" property="money" jdbcType="INTEGER"/>
<result column="is_deleted" property="isDeleted" jdbcType="TINYINT"/>
<result column="create_at" property="createAt" jdbcType="TIMESTAMP"/>
<result column="update_at" property="updateAt" jdbcType="TIMESTAMP"/>
</resultMap>
<sql id="money_po">
id, name, money, is_deleted, create_at, update_at
</sql>
</mapper>
1. @Param注解
在接口的参数上添加@Param
注解,在内部指定传递给xml的参数名
一个简单的case如下
int addMoney(@Param("id") int id, @Param("money") int money);
重点关注上面的参数
- 通过
@Param
来指定传递给xml时的参数名
对应的xml文件中的sql如下,使用#{}
来实现参数绑定
<update id="addMoney" parameterType="java.util.Map">
update money set money=money+#{money} where id=#{id}
</update>
2. 单参数
接下来我们看一下不使用@Param
注解时,默认场景下,xml中应该如何指定参数;因为单参数与多参数的实际结果不一致,这里分开进行说明
单参数场景下,xml中的参数名,可以用任意值来表明
mapper接口定义如下
/**
* 单个参数时,默认可以直接通过参数名来表示,实际上#{}中用任意一个值都可以,没有任何限制,都表示的是这个唯一的参数
* @param id
* @return
*/
MoneyPo findById(int id);
/**
* 演示xml中的 #{} 为一个匹配补上的字符串,也可以正确的实现参数替换
* @param id
* @return
*/
MoneyPo findByIdV2(int id);
对应的xml文件内容如下
<select id="findById" parameterType="java.lang.Integer" resultMap="BaseResultMap">
select
<include refid="money_po"/>
from money where id=#{id}
</select>
<select id="findByIdV2" parameterType="java.lang.Integer" resultMap="BaseResultMap">
select
<include refid="money_po"/>
from money where id=#{dd}
</select>
重点看一下上面的findByIdV2
,上面的sql中传参使用的是 #{dd}
,和mapper接口中的参数名并不相同,但是最终的结果却没有什么区别
3. 多参数
当参数个数超过1个的时候,#{}
中的参数,有两种方式
- param1…N: 其中n代表的接口中的第几个参数
- arg0…N
/**
* 不指定参数名时,mybatis自动封装一个 param1 ... paramN的Map,其中n表示第n个参数
* 也可以使用 arg0...n 来指代具体的参数
*
* @param name
* @param money
* @return
*/
List<MoneyPo> findByNameAndMoney(String name, Integer money);
对应的xml如下
<select id="findByNameAndMoney" resultMap="BaseResultMap">
select
<include refid="money_po"/>
-- from money where name=#{param1} and money=#{param2}
from money where name=#{arg0} and money=#{arg1}
</select>
注意上面的xml中,两种传参都是可以的,当然不建议使用这种默认的方式来传参,因为非常不直观,对于后续的维护很不优雅
3. Map传参
如果参数类型并不是简单类型,当时Map类型时,在xml文件中的参数,可以直接使用map中对应的key来指代
/**
* 参数类型为map时,直接使用key即可
* @param map
* @return
*/
List<MoneyPo> findByMap(Map<String, Object> map);
对应的xml如下
<select id="findByMap" resultMap="BaseResultMap">
select
<include refid="money_po"/>
from money
<trim prefix="WHERE" prefixOverrides="AND | OR">
<if test="id != null">
id = #{id}
</if>
<if test="name != null">
AND name=#{name}
</if>
<if test="money != null">
AND money=#{money}
</if>
</trim>
</select>
4. POJO对象
另外一种常见的case是传参为简单的实体对象,这个时候xml中的参数也可以直接使用对象的fieldName来指代,和map的使用方式差不多
/**
* 参数类型为java对象,同样直接使用field name即可
* @param po
* @return
*/
List<MoneyPo> findByPo(MoneyPo po);
对应的xml文件如下
<select id="findByPo" parameterType="com.git.hui.boot.mybatis.entity.MoneyPo" resultMap="BaseResultMap">
select
<include refid="money_po"/>
from money
<trim prefix="WHERE" prefixOverrides="AND | OR">
<if test="id != null">
id = #{id}
</if>
<if test="name != null">
AND name=#{name}
</if>
<if test="money != null">
AND money=#{money}
</if>
</trim>
</select>
5. 简单参数 + Map参数
当参数有多个,其中部分为简单类型,部分为Map,这样的场景下参数如何处理呢?
- 简单类型遵循上面的规则
- map参数的传参,使用前缀 + “.” + key的方式
一个实例如下
List<MoneyPo> findByIdOrCondition(@Param("id") int id, @Param("map") Map<String, Object> map);
List<MoneyPo> findByIdOrConditionV2(int id, Map<String, Object> map);
对应的xml如下
<select id="findByIdOrCondition" resultMap="BaseResultMap">
select <include refid="money_po"/> from money where id = #{id} or `name`=#{map.name}
</select>
<select id="findByIdOrConditionV2" resultMap="BaseResultMap">
select <include refid="money_po"/> from money where id = #{param1} or `name`=#{param2.name}
</select>
本文主要介绍mybatis中传参的几种姿势:
- 默认场景下,单参数时,xml文件中可以用任意名称代替传参
- 默认场景下,多参数时,第一个参数可用 param1 或 arg0来表示,第二个参数为 param2 或 arg1。。。
- 单参数,且为map时,可以直接使用map的key作为传参
- 单参数,pojo对象时,使用对象的fieldName来表示传参
- @Param注解中定义的值,表示这个参数与xml中的占位映射关联
- 多参数场景下,简单对象 + map/pojo时,对于map/pojo中的参数占位,可以通过
paramN.xxx
的方式来完成
III. 不能错过的源码和相关知识点
系列博文
1. 微信公众号: 一灰灰Blog
尽信书则不如,以上内容,纯属一家之言,因个人能力有限,难免有疏漏和错误之处,如发现bug或者有更好的建议,欢迎批评指正,不吝感激
下面一灰灰的个人博客,记录所有学习和工作中的博文,欢迎大家前去逛逛
一灰灰blog
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK