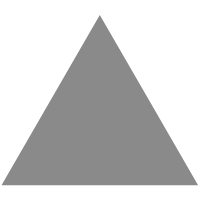
5
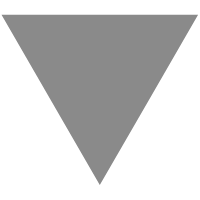
Rails models cheatsheet
source link: https://devhints.io/rails-models
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
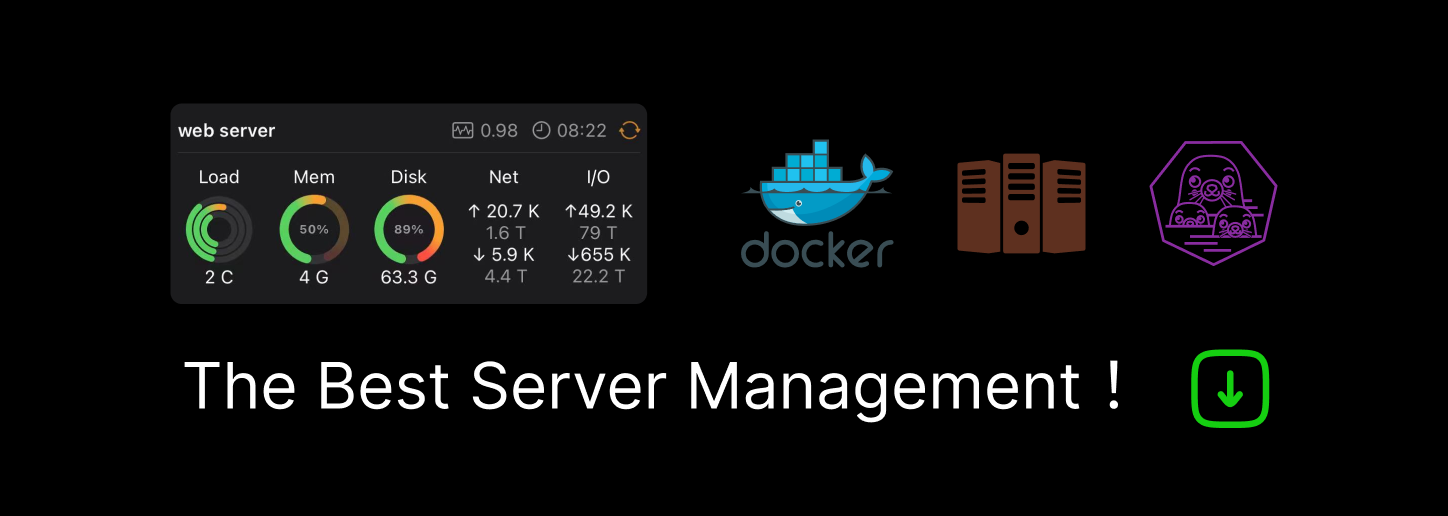
#Using models
Query methods
items = Model
.where(first_name: 'Harvey')
.where('id = 3')
.where('id = ?', 3)
.order(:title)
.order(title: :desc)
.order("title DESC")
.reorder(:title) # discards other .order's
.rewhere(...) # discards other .where's
.limit(2)
.offset(1)
.uniq
See: QueryMethods
Advanced query methods
items = Model
.select(:id)
.select([:id, :name])
.group(:name) # GROUP BY name
.group('name AS grouped_name, age')
.having('SUM(price) > 30') # needs to be chained with .group
.includes(:user)
.includes(user: [:articles])
.references(:posts)
# aka: .where("posts.name = 'foo'").references(:posts)
Finder methods
item = Model.find(id)
item = Model.find_by_email(email)
item = Model.where(email: email).first
Model
.exists?(5)
.exists?(name: "David")
.first
.last
.find_nth(4, [offset])
See: FinderMethods
Persistence
item.new_record?
item.persisted?
item.destroyed?
item.serialize_hash
item.save
item.save! # Same as above, but raises an Exception
item.update name: 'John' # Saves immediately
item.update! name: 'John'
item.update_column :name, 'John' # skips validations and callbacks
item.update_columns name: 'John'
item.update_columns! name: 'John'
item.touch # updates :updated_at
item.touch :published_at
item.destroy
item.delete # skips callbacks
Model.create # Same an #new then #save
Model.create! # Same as above, but raises an Exception
See: Persistence
Attribute Assignment
item.attributes # #<Hash>
item.attributes = { name: 'John' } # Merges attributes in. Doesn't save.
item.assign_attributes name: 'John' # Same as above
See: AttributeAssignment
Dirty
item.changed?
item.changed # ['name']
item.changed_attributes # { 'name' => 'Bob' } - original values
item.changes # { 'name' => ['Bob', 'Robert'] }
item.previous_changes # available after #save
item.restore_attributes
item.name = 'Robert'
item.name_was # 'Bob'
item.name_change # [ 'Bob', 'Robert' ]
item.name_changed? # true
item.name_changed?(from: 'Bob', to: 'Robert')
See: Dirty
Validations
item.valid?
item.invalid?
See: Validations
Calculations
Person.count
Person.count(:age) # counts non-nil's
Person.average(:age)
Person.maximum(:age)
Person.minimum(:age)
Person.sum('2 * age')
Person.calculate(:count, :all)
Advanced:
Person.distinct.count
Person.group(:city).count
See: Calculations
Dynamic attribute-based finders
Given a field called name
:
# Returns one record
Person.find_by_name(name)
Person.find_last_by_name(name)
Person.find_or_create_by_name(name)
Person.find_or_initialize_by_name(name)
# Returns a list of records
Person.find_all_by_name(name)
# Add a bang to make it raise an exception
Person.find_by_name!(name)
# You may use `scoped` instead of `find`
Person.scoped_by_user_name
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK