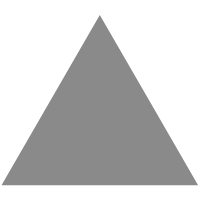
5
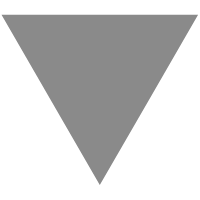
Linked List - Insert a node at the end of the list in C
source link: https://www.codesd.com/item/linked-list-insert-a-node-at-the-end-of-the-list-in-c.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
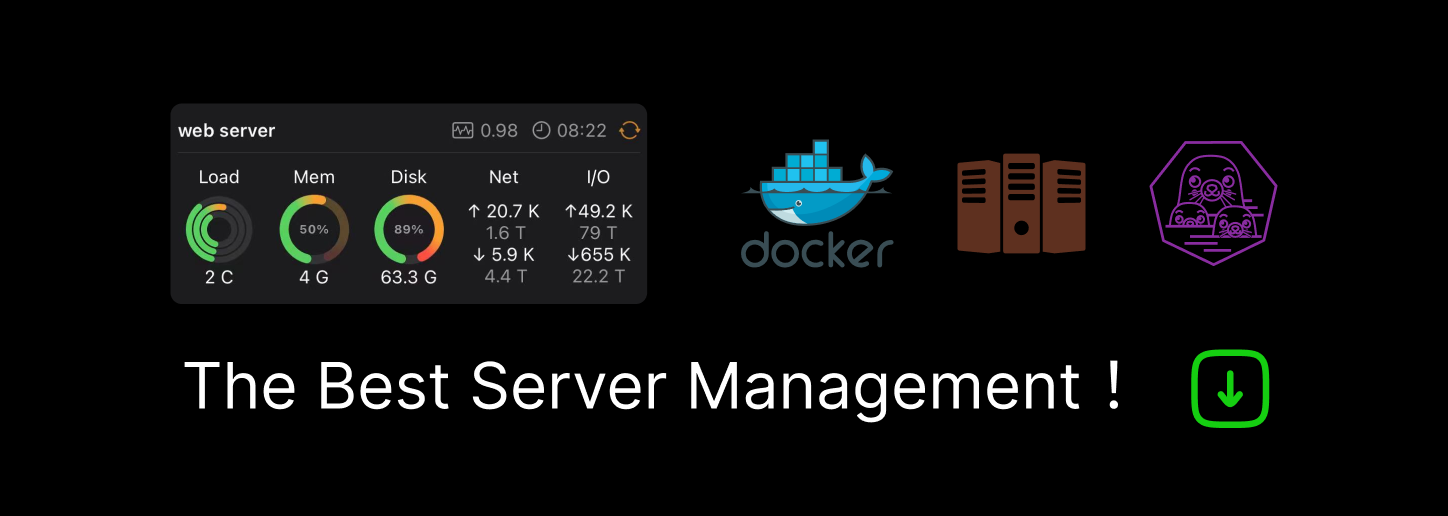
Linked List - Insert a node at the end of the list in C
advertisements
This is the code for insertion of a node at the end. I am not getting any error while running but it shows that the programme has stopped working. please tell me where i have messed it here?
enter code here
#include <stdio.h>
#include <stdlib.h>
struct node{
int data;
struct node* next;
};
struct node* head;
void Insert(int data)
{
struct node* temp, *temp2;
temp=(struct node*)malloc(sizeof(struct node));
temp->data = data;
temp2 = head;
while(temp2 != NULL)
{
temp2 = temp2->next;
}
temp2->next = temp;
temp->next = NULL;
}
void print()
{
struct node* temp = head;
while(temp!=NULL)
{
printf("%d", temp->data);
}
printf("\n");
}
int main()
{
head = NULL;
Insert(1);
Insert(2);
Insert(3);
Insert(4);
Insert(5);
print();
return 0;
}
while(temp2 != NULL)
{
temp2 = temp2->next;
}
temp2->next = temp;
After this loop temp2
is always NULL
.
Tags
data-structures
Related Articles
Inserting a node at the end of a linked list
#include <stdio.h> #include <conio.h> struct node { int data; struct node* next; }; int main() { struct node* head = NULL; struct node* second = NULL; struct node* third = NULL; head = (struct node*)malloc(sizeof(struct node)); second = (struc
insert a node at the end of the recursively linked list
typedef struct node { int a; struct node *next; }node; void generate(struct node **head) { int num = 10, i; //the num here is the length struct node *temp; for (i = 0; i < num; i++) { temp = (struct node*)malloc(sizeof(struct node)); temp->a = 10-i;
Insert a node at the tail of a list linked python HackerRank
Its a very simple program of adding a node at the end of a link list. I dont know what mistake I am doing. has it something to do with the exoected output of hackerRank or there's a mistake in my code. I m trying to implement the Python2 class Node(o
Why does not the following code to insert a node at the end of a linked list separately work?
Below is the code in C language: function call: insert(&head,value); void insert(struct node** headref,int value) { struct node* head = (*headref); while( head!=NULL ) { head= head->link; } struct node* new_node=(struct node*)malloc( sizeof(struct
How to insert a node in the linked list using java?
I'm trying this method but I can't figure out the problem. I think that there is some problem in insert() method because I have not used recursion but i can't exactly pinpoint it. Thanks in advance. import java.io.*; import java.util.*; class Node {
Insert a node between the text
I am familiar with inserting text nodes after or before a given reference node. But, I would like to know how to insert a tag between text in a given node. For example, Before insertion: <p>Lorem dolor</p> After insertion: <p>Lorem <s
Insert a node in the nth position
node* insertnth(struct node_q* list,int ndex,struct node_q* qtemp){//temp is the node to be inserted at the back of ndex struct node_q* temp; struct node_q* curr; int i; temp = list; curr = temp; for(i=0;i<=ndex;i++){ if(temp!=NULL) temp = temp->pne
Data structure in C ++. Insert at the beginning of the node in the linked list
I am learning data structures in C++. This is a simple program for insertion using links and nodes. The insertion takes place at the beginning of the node. I do not understand some parts of the code. In the function display() the pointer np points to
Insert a new node at the beginning of the linked list
In a simple Linked List implementation on C, I couldn't figure out a line of function named insert(). It takes a char and add to the linked list in alphabetical order. The line is about creating a new node when the list is empty. And since there will
Inserting a new node at the beginning of a linked list in Java
I have checked few posts that we have in SO. Insert new node at the beginning of Linked-List How do I insert a node at the beginning of a linked list? And implemented a simple LinkedList in java that works just fine. What I can't get my head around i
Insert at the end of a doubly linked list
I'm working with linked lists for my first time and have to create a function that can insert a node at the end of a doubly linked list. So far I have void LinkedList::insertAtTail(const value_type& entry) { Node *newNode = new Node(entry, NULL, tail
Why does not adding a new node to the front of the linked list work in my code?
why can't I insert a new node to my linked list as follows? My insertNodeToHead only works if my return type is a Node itself and if I return root. But I want to be able to change the linked list itself without returning anything. Currently, it shoul
Insert n nodes in a linked list and print their data (C ++)
I don't understand what's wrong with my code here: //Inserting n nodes, then print their values #include <iostream> #include <string> using namespace std; //Defining a node and it's head pointer struct node { int data; node *next; }; node *hea
How do I insert an arbitrary node into a linked list?
I'm about to implement a function that is able to insert a node in a linked list arbitrary . The Code below works properly for inserting a node at the first of the list but for putting a node after another node it doesn't work . I honestly can't figu
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK