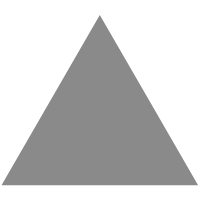
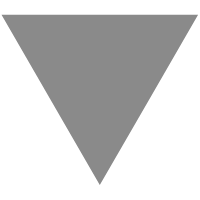
Fundamentals of Tensorflow - Part I - Knoldus Blogs
source link: https://blog.knoldus.com/fundamentals-of-tensorflow-part-i/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
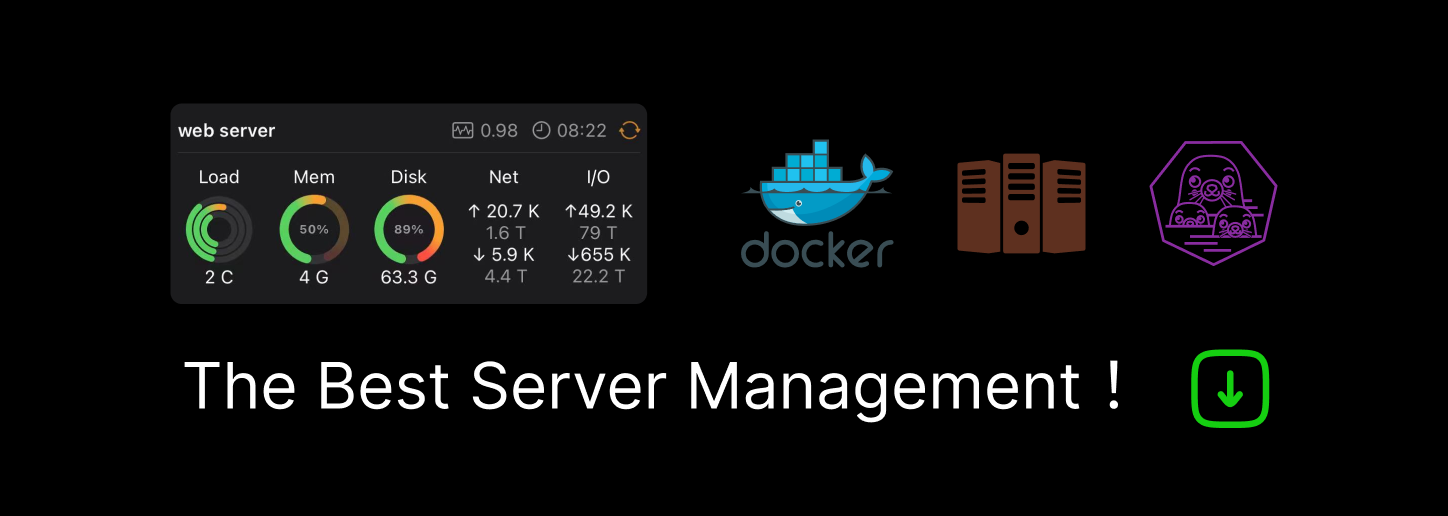
What is TensorFlow?
TensorFlow is an open-source end-to-end machine learning library. It is for preprocessing data, modeling data, and serving models (getting them into the hands of others).
It has a comprehensive, flexible ecosystem of tools, libraries, and community resources that lets researchers push the state-of-the-art in ML. And developers easily build and deploy ML-powered applications.
Installing TensorFlow
TensorFlow is tested and supported on the following 64-bit systems:
- Python 3.6–3.9
- Ubuntu 16.04 or later
- Windows 7 or later (with C++ redistributable)
- macOS 10.12.6 (Sierra) or later (no GPU support)
Download a package
Install TensorFlow with Python’s pip package manager.
Note : TensorFlow 2 packages require a pip
version >19.0 (or >20.3 for macOS).
# Requires the latest pippip install --upgrade pip
# Current stable release for CPU and GPUpip install tensorflow
# Or try the preview build (unstable)pip install tf-nightly
Run a TensorFlow container
The TensorFlow Docker images are already configured to run TensorFlow. And, Docker container runs in a virtual environment and is the easiest way to set up GPU support.
docker pull tensorflow/tensorflow:latest # Download latest stable image
docker run -it -p 8888:8888 tensorflow/tensorflow:latest-jupyter # Start Jupyter server
Google Colab: An easy way to learn and use TensorFlow
No install necessary—run the TensorFlow tutorials directly in the browser with Colaboratory. A Google research project created to help disseminate machine learning education and research. It’s a Jupyter notebook environment that requires no setup to use and runs entirely in the cloud. Read the blog post.
Introduction to Tensors
If you’ve ever used NumPy, tensors are kind of like NumPy arrays.
The main difference between tensors and NumPy arrays is that tensors can be used on GPUs and TPUs (tensor processing units).
The benefit of being able to run on GPUs and TPUs is faster computation. Therefore, if we wanted to find patterns in the numerical representations of our data, we can generally find them faster using GPUs and TPUs.
Okay, we’ve been talking enough about tensors, let’s see them.
The first thing we’ll do is import TensorFlow under the common alias tf.
# Import TensorFlow
import tensorflow as tf
print(tf.__version__) # find the version number (should be 2.x+)
Creating Tensors
As most programs start by declaring variables. Likeweise, tensorFlow applications start by making tensors.
A tensor is an array with zero or more dimensions. A zero-dimensional tensor is known as a scalar. A one-dimensional tensor is known as a vector. And a two-dimensional tensor is known as a matrix.
The tf package provides seven functions that form tensors with known values. The following table lists them and provides a description of each.
Creating Tensors with Known Values
FunctionDescriptionconstant(value, dtype=None, shape = None, name = ‘Const’, verify_shape=False)Returns a tensor containing the given valuezeros(shape, dtype=tf.float32, name = None)Returns a tensor filled with zerosones(shape, dtype=tf.float32, name=None)Returns a tensor filled with onesfill(dims, value, name=None)Returns a tensor filled with the given valuelinspace(start, stop, num, name=None)Returns a tensor containing a linear range of valuesrange(start, limit, delta=1, dtype=None, name=’range’)Returns a tensor containing a range of valuesrange(limit, delta=1, dtype=None, name=’range’)Returns a tensor containing a range of values
A tensor may have multiple dimensions and the number of dimensions in a tensor is its rank. The lengths of a tensor’s dimensions form an array called the tensor’s shape. Many of the functions in the table accept a shape parameter that identifies the desired shape of the new tensor. The following examples demonstrate how you can set this parameter:
[]
— The tensor contains a single value.[3]
— The tensor is a one-dimensional array containing three values.[3, 4]
— The tensor is a 3-x-4 matrix.[3, 4, 5]
— The tensor is a multidimensional array whose dimensions equal 3, 4, and 5.
Tensor Data Types
Data TypeDescriptionbool
Boolean valuesuint8/uint16
Unsigned integersquint8/quint16
Quantized unsigned integersint8/int16/int32/int64
Signed integersqint8/qint32
Quantized signed integersfloat16/float32/float64
Floating-point valuescomplex64/complex128
Complex floating-point valuesstring
Strings
Each function in the preceding table accepts an optional name argument that serves as an identifier for the tensor. Applications can access a tensor by name through the tensor’s graph.
The most popular function in the table is constant. Its only required argument is the first. It defines the value or values to be stored in the tensor. You can provide these values in a list, and the following code creates a one-dimensional tensor containing three floating-point values:
t1 = tf.constant([1.5, 2.5, 3.5])
Multidimensional arrays use similar notation. The following code creates a 2-x-2 matrix and sets each of its elements to the letter b:
t2 = tf.constant([['b', 'b'], ['b', 'b']])
But, here TensorFlow won’t raise an error if the function’s first argument doesn’t have the shape given by the shape argument. But if you set the last argument, verify_shape, to True. So, TensorFlow will verify that the two shapes are equal. The following code provides an example of mismatched shapes:
t3 = tf.constant([4, 2], tf.int16, [3], 'Const', True)
In this case, the given shape, [3]
, doesn’t match the shape of the first argument, which is [2]. As a result, TensorFlow displays the following error:
TypeError: Expected Tensor's shape: (3,), got (2,).
zeros, ones, and fill
However, the functions zeros, ones, and fill create tensors whose elements all have the same value for zeros and ones, the only required argument is shape. In addition it identifies the shape of the desired tensor. As an example, the following code creates a simple 1-x-3 vector whose elements equal 0.0:
zero_tensor = tf.zeros([3])
Similarly, the following function call creates a 4-x-4 matrix whose elements equal 1.0:
one_tensor = tf.ones([4, 4])
The fill function requires a value parameter which sets the value of the tensor’s elements. The following code creates a three-dimensional tensor whose values are set to 81.0:
fill_tensor = tf.fill([1, 2, 3], 81.0)
Unlike zeros and ones, fill doesn’t have a dtype argument. As a result, It can only create tensors containing 32-bit floating point values.
Creating sequences
The linspace and range functions create tensors. And, whose elements change regularly between a start and end value. The difference between them is that linspace creates a tensor with a specific number of values. For example, the following code creates a 1-x-5 tensor whose elements range from 5.0 to 9.0:
lin_tensor = tf.linspace(5., 9., 5) # Result: [5. 6. 7. 8. 9.]
Unlike linspace, range doesn’t accept the number of elements in the tensor. Instead, it computes successive elements by adding a value called a delta. In the following code, delta is set to 0.5:
range_tensor = tf.range(3., 7., delta=0.5) # Result: [3.0 3.5 4.0 4.5 5.0 5.5 6.0 6.5]
For instance Python’s range function, TensorFlow’s range function can be called without the start parameter. In this case, the starting value is assumed to be 0.0. The following code demonstrates this:
range_tensor = tf.range(1.5, delta=0.3) # Result: [0.0 0.3 0.6 0.9 1.2]
If the delta parameter is positive. As a result, the starting value must be less than the ending value. And, if delta is negative. As a result, the starting value must be greater than the ending value.
Conclusion
In conclusion, in this blog we have attained Fundamentals of TensorFlow. So, In next blog we’ll learn more about Tensorflow deeply.
HAPPY LEARNING
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK