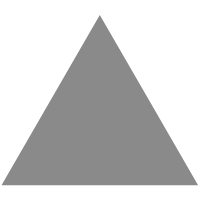
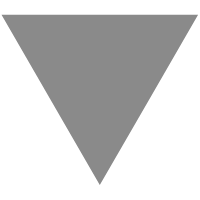
Understanding Maps and Sets in JavaScript
source link: https://www.makeuseof.com/javascript-map-set-data-structures/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
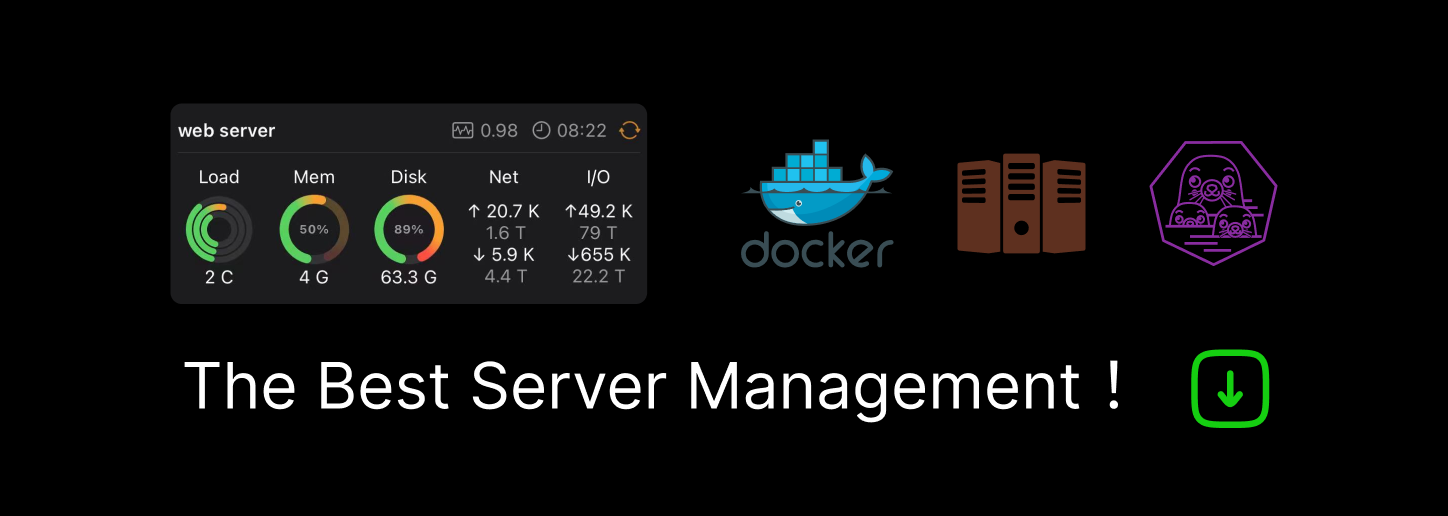
Understanding Maps and Sets in JavaScript
Published 9 hours ago
The set and map data structures are fundamental to computer programming. Learn how to use JavaScript's native implementations and simplify your code.
Data structures are the backbone of any programming language. Examples include the linked list, trees, stacks, queues, and graphs. In this article, you will learn how to use the map and set data structures in JavaScript.
In 2015 JavaScript introduced ES6 to provide developers with an enhanced coding experience. ES6 introduced maps and sets so that developers don't have to build them using primitive data types. Let's take a look at them in greater detail.
Understanding Maps in JavaScript
The map is a type of data structure that stores key-value pairs. It is one of the most efficient methods to search and retrieve data. You can store all types of data in JavaScript maps.
Let's have a look at some methods that you can perform on JavaScript maps.
Related: How To Build Data Structures With JavaScript ES6 Classes
1. set()
You can insert a key-value pair into a map using the set() method. This method takes two arguments: the key and the value.
Check out the code below that shows you how to insert into a map.
let map = new Map();
map.set("fruit1", "Grapes");
map.set("fruit2", "Mango");
map.set("fruit3", "Strawberry");
map.set("fruit4", "Mango");
console.log(map)
The output after printing the map:
Map {
'fruit1' => 'Grapes',
'fruit2' => 'Mango',
'fruit3' => 'Strawberry',
'fruit4' => 'Mango' }
2. delete()
To delete an element from a map, use the delete() method. This method accepts one argument: the key of the data you want to delete. If the key exists, this method removes that entry from the map and returns true. Otherwise, it returns false.
console.log("Is the fruit1 deleted", map.delete("fruit1"));
console.log(map)
The output after deleting the fruit1:
Is the fruit1 deleted true
Map {
'fruit2' => 'Mango',
'fruit3' => 'Strawberry',
'fruit4' => 'Mango' }
3. get()
The get() method retrieves the value of the specified key. Like the delete() method, it accepts one argument: the key.
console.log(map.get("fruit2"))
Produces the following output:
Mango
4. size()
The size() method returns the number of the key-value pairs present in the map.
let map = new Map();
map.set("fruit1", "Grapes");
map.set("fruit2", "Mango");
map.set("fruit3", "Strawberry");
map.set("fruit4", "Mango");
console.log("The size of the map is", map.size)
Gives the following result:
The size of the map is 4
5. clear()
To delete all the elements from the map, use the clear() method.
map.clear()
console.log(map)
The output of the map after deleting all the items:
Map {}
6. has()
The has() method helps you to check if the key exists in a map or not. It returns a boolean value depending on the existence of an item in the map. This method accepts one argument: the key to search for.
console.log("The grapes exists:", map.has("fruit1"))
Produces the following output with our example map:
The grapes exists: true
7. keys()
The keys() method returns an iterator with all the keys in it. You can loop through them to get access to an individual key.
const keys = map.keys();
for (let k of keys) {
console.log(k);
}
The output of the above code is:
fruit1
fruit2
fruit3
fruit4
8. values
The values() method returns an iterator with all the values in it.
const values = map.values();
for (let v of values) {
console.log(v);
}
The output of the above code is:
Grapes
Mango
Strawberry
Mango
Understanding Sets in JavaScript
The set data structure has unique values, which means no value can repeat. You can store all kinds of values in a set including primitives and objects.
Related: How to Remove Duplicate Elements From an Array in JavaScript, Python, and C++
Let us have a look at some methods in the set:
1. add()
The add() method helps you to add data into the set. If you try to add duplicate data, the set will simply ignore it.
let s = new Set();
s.add(1);
s.add(2);
s.add(3);
s.add(4);
s.add(4);
console.log(s);
Gives the output:
Set { 1, 2, 3, 4 }
2. delete()
The delete() method deletes the specified value from the set.
s.delete(3);
console.log(s);
The output after deleting 3:
Set { 1, 2, 4 }
3. has()
The has() methods helps you check if a value exists in the set or not.
console.log(s.has(1))
The output of the above code is true.
4. clear()
The clear() method helps you to delete all the items in the set.
s.clear();
console.log(s);
The output after deleting all the items in the set:
Set {}
Learn More About ES6
Now you've learned how to use maps and sets in JavaScript, it's time for you to implement them in your projects. You can use them while solving data structure and algorithm problems. You can use a map to implement a hashing algorithm.
Want to learn more about ES6? Check out the article below that will tell you all about it.
About The Author
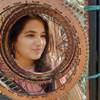
Unnati Bamania (4 Articles Published)
Unnati is an enthusiastic full stack developer. She loves to build projects using various programming languages. In her free time, she loves to play the guitar and is a cooking enthusiast.
Subscribe to our newsletter
Join our newsletter for tech tips, reviews, free ebooks, and exclusive deals!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK