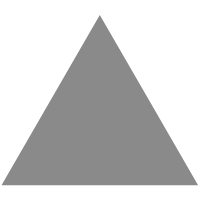
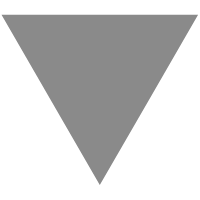
Using Recursion to get the sqrt sum of a list
source link: https://www.codesd.com/item/using-recursion-to-get-the-sqrt-sum-of-a-list.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Using Recursion to get the sqrt sum of a list
Ive been trying to create a recursive function that takes a list of integers and gets the sum of the square root. i've come from c# and im pretty new to haskell. I feel as though ive hit a comprehension block
i was having problems with types so i tried making an integer sqrt to help myself out, but ended up confusing myself more..
isqrt :: Integer -> Integer
isqrt = floor . sqrt . fromIntegral
sumsquares :: Int a => [a] -> a
sumsquares f [] = "List is Empty"
sumsquares f (x:xs) | sum . isqrt x | x <- xs
ive only done a little recursion and i can't find anywhere that really explains it in a way i understand
How your code is uncompilable
sumsquares :: Int a => [a] -> a
The type signature is weird. Int
is a type but you are using with the class syntax. What you really meant ("a list of integers") was:
[Integer] -> Integer
You are also taking as an argument an f
that simply isn't there. This makes me think that you thought Int a =>
was an argument. It's not. Anything in the form X a =>
is a type class constraint that does not contribute to the arity of the function.
Then we have:
sumsquares f [] = "List is Empty"
This is unreasonable and incorrect. Generally speaking one would expect that the sum of an empty list is 0
or mempty
(that's for a more complicated topic). "List is Empty"
is a String
(unless OverloadedString
is in place), so that line wouldn't even compile.
Finally:
sumsquares f (x:xs) | sum . isqrt x | x <- xs
I don't know what you were trying to do here, but |
is used for conditionals. For example:
sumSquares :: [Integer] -> Integer
sumSquares x
| null x = 0
| otherwise = (isqrt . head $ x) + tail x
Solution with explicit recursion
That would be like this:
sumSquares :: [Integer] -> Integer
sumSquares [] = 0
sumSquares (x:xs) = isqrt x + sumSquares xs
Solution with map
Using map
, it's simply:
sumSquares :: [Integer] -> Integer
sumSquares = sum . map isqrt
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK