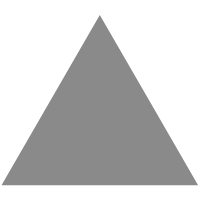
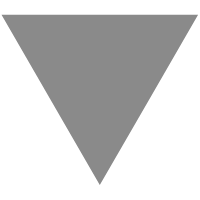
NullpointerException in Collection.sort () with multithreading
source link: https://www.codesd.com/item/nullpointerexception-in-collection-sort-with-multithreading.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
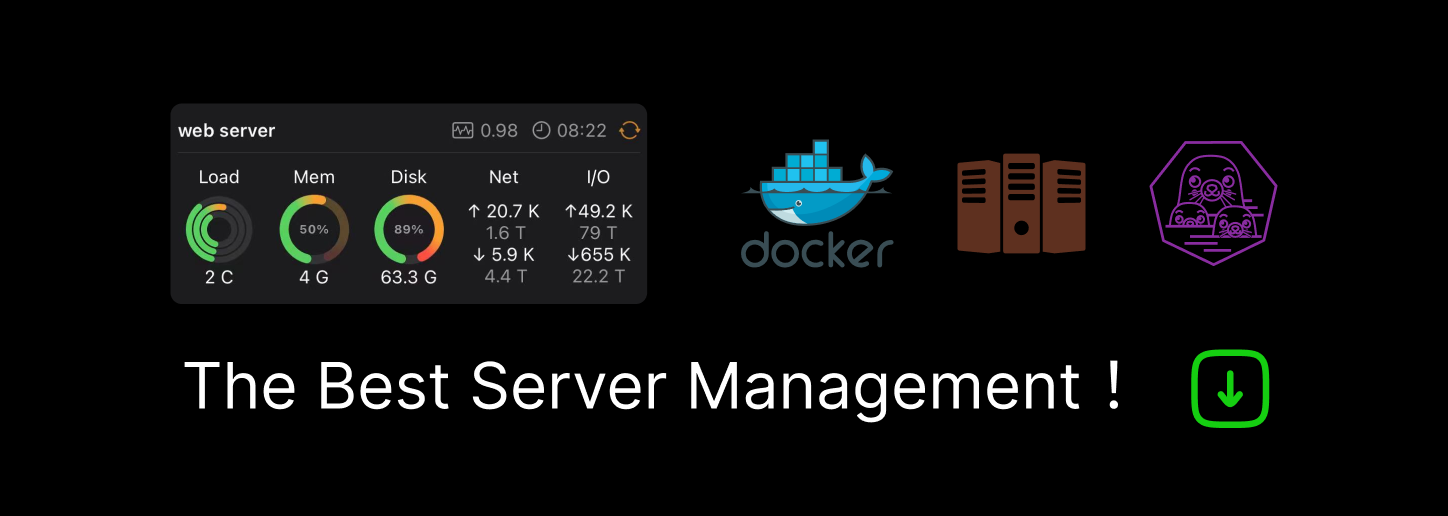
NullpointerException in Collection.sort () with multithreading
I have a class that should test my Fibonacci function using multithreading
public class PerformanceTesterImpl implements PerformanceTester{
public static List<Long> executionTimesList = new ArrayList();
public static List<Runnable> tasksList = new ArrayList();
public int fib;
public PerformanceTestResult performanceTestResult;
@Override
public PerformanceTestResult runPerformanceTest(Runnable task, int calculationCount, int threadPoolSize) {
for(int i=0; i<calculationCount; i++){
tasksList.add(createTask(fib));
}
ExecutorService executor = Executors.newFixedThreadPool(threadPoolSize);
for(Runnable r : tasksList){
executor.execute(r);
}
executor.shutdown();
try {
executor.awaitTermination(1, TimeUnit.MINUTES);
} catch (InterruptedException e) {
e.printStackTrace();
}
// Here all threads should complete all work. Is it OK?
mapValues();
return performanceTestResult;
}
private PerformanceTestResult mapValues(){
Collections.sort(executionTimesList);
performanceTestResult = new PerformanceTestResult(getSum(executionTimesList), (Long)executionTimesList.get(0), (Long)executionTimesList.get(executionTimesList.size()-1));
return performanceTestResult;
}
public Runnable createTask (final int n) {
fib = n;
Runnable runnable = new Runnable() {
@Override
public void run() {
long startTime = System.currentTimeMillis();
FibCalc fibCalc = new FibCalcImpl();
fibCalc.fib(n);
long executionTime = System.currentTimeMillis() - startTime;
executionTimesList.add(executionTime);
}
};
return runnable;
}
private static long getSum(List<Long> executionTimes){
long sum = 0;
for(long l : executionTimes){
sum += l;
}
return sum;
}
}
but from time to time NULL appears in my collection and when I'm trying to sort executionTimeList I receive NullpointerException. I think there is a problem with executing threads. What should I do to correct this exception?
executionTimesList is shared among all threads. They run in concurrence in your code with no synchronization. So it's logical that any inconsistency state appears if a thread works on the list and has not finished its job and the cpu gives the priority to another thread which works on the list too, the first thread will be in a inconsistent state when it will be restarted. You must synchronize the access for the static field executionTimesList
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK