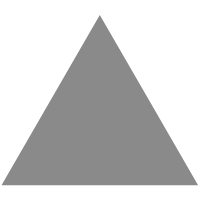
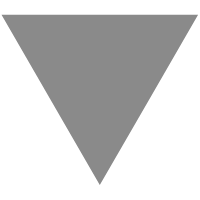
Parsing JSON with PowerShell
source link: https://techcommunity.microsoft.com/t5/core-infrastructure-and-security/parsing-json-with-powershell/ba-p/2768721?WT_mc_id=DOP-MVP-4025064
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
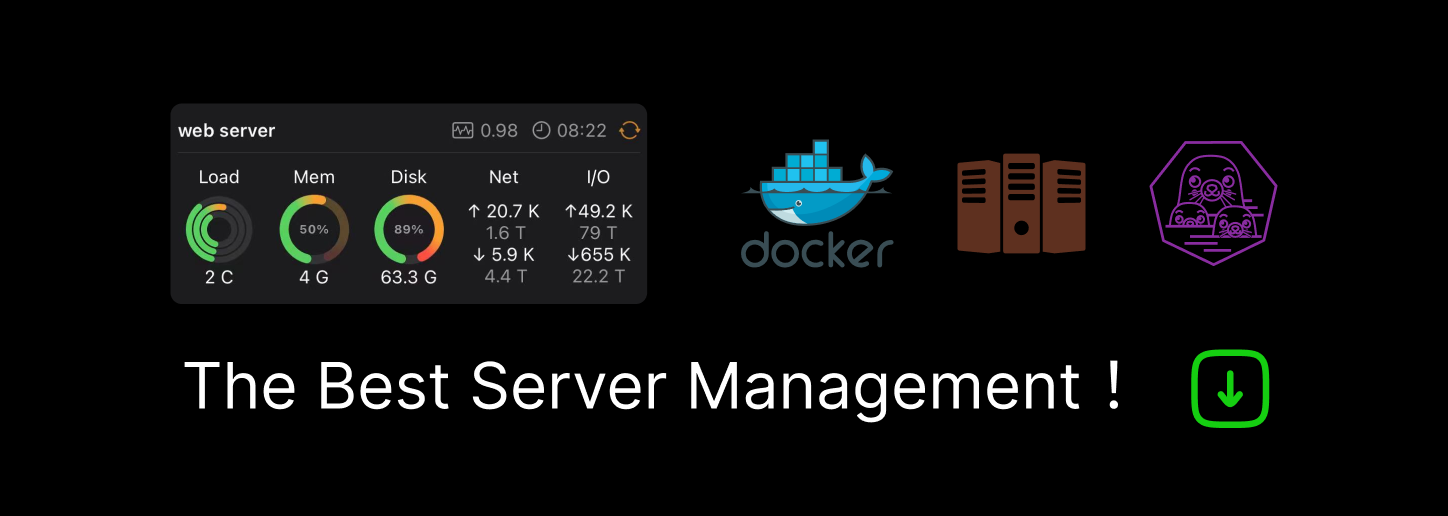
Parsing JSON with PowerShell
Q: I try to parse my JSON with the handy dandy “ConvertFrom-JSON” cmdlet but it only works in PowerShell 7, not in my good old PowerShell 5.1. How do I get it to work everywhere?
A: PS 7 parses JSON a little differently than PS 5.1 by ignoring comments and accepting less than ideal JSON so you need to clean up your JSON for PS 5.1. Use the function below to do it for you.
Java Script Object Notation (JSON) is a popular format these days for sending and receiving data with Azure. JSON is used for sending and receiving data using Azure REST API, deploying resources to Azure using ARM templates, configure governance in Azure using Azure Policy, and much more. PowerShell is a great tool for creating and modifying JSON. PowerShell 7.1 is my preferred solution since it has additional capabilities however sometimes we still need good old PS 5.1 so it is nice to be able to accomplish anything in either version.
Disclaimer: The sample scripts are not supported under any Microsoft standard support program or service. The sample scripts are provided AS IS without warranty of any kind. Microsoft further disclaims all implied warranties including, without limitation, any implied warranties of merchantability or of fitness for a particular purpose. The entire risk arising out of the use or performance of the sample scripts and documentation remains with you. In no event shall Microsoft, its authors, or anyone else involved in the creation, production, or delivery of the scripts be liable for any damages whatsoever (including, without limitation, damages for loss of business profits, business interruption, loss of business information, or other pecuniary loss) arising out of the use of or inability to use the sample scripts or documentation, even if Microsoft has been advised of the possibility of such damages.
First, here is a sample file I’ll use for the example or you can download it from here.
{
"Accounts": {
"Users": [
{
"jdoe": {
"givenName": "John", //Out on leave this month
"surname": "Doe",
"department": "Finance"
},
"jdoe2": {
"givenName": "Jane", //Department head
"surname": "Doe",
"department": "Marketing"
},
"asmith": {
"givenName": "Alice", /* Sits in the corner office */
"surname": "Smith",
"department": "IT"
}
}
]
}
}
Alice got promoted so we need to change her department to Senior Leadership. PowerShell makes it easy to modify JSON by converting JSON to a PSCustomObject. The object can then be modified easily like any other object. The object can then be exported back out using ConvertTo-Json.
$myJson = Get-Content .\test.json -Raw | ConvertFrom-Json
$myJson.Accounts.Users.asmith.department = "Senior Leadership"
$myJson | ConvertTo-Json -Depth 4 | Out-File .\test.json
This gives a test.json file that accomplished exactly what we wanted:
{
"Accounts": {
"Users": [
{
"jdoe": {
"givenName": "John",
"surname": "Doe",
"department": "Finance"
},
"jdoe2": {
"givenName": "Jane",
"surname": "Doe",
"department": "Marketing"
},
"asmith": {
"givenName": "Alice",
"surname": "Smith",
"department": "Senior Leadership"
}
}
]
}
}
Now if we’re on a computer without PowerShell 7.1 we try to run the same command in PowerShell 5.1 but it fails!
PS> $a = gc .\test.json -Raw | ConvertFrom-Json
ConvertFrom-Json : Invalid object passed in, ':' or '}' expected. (17161):{
<the entire contents of the json file listed here>
}
At line:1 char:28
+ $a = gc .\test.json -Raw | ConvertFrom-Json
+ ~~~~~~~~~~~~~~~~
+ CategoryInfo : NotSpecified: (:) [ConvertFrom-Json], ArgumentException
+ FullyQualifiedErrorId : System.ArgumentException,Microsoft.PowerShell.Commands.ConvertFromJsonCommand
Comments will prevent ConvertFrom-Json from working properly in PowerShell 5.1. I like to use this simple function to fix my JSON for me.
Function Remove-Comments{
param(
[CmdletBinding()]
[Parameter(Mandatory=$true,ValueFromPipelineByPropertyName=$true,Position=0)]
[string]
[ValidateScript({test-path $_})]
$File
)
$CComments = "(?s)/\\*.*?\\*/"
$content = Get-Content $File -Raw
[regex]::Replace($content,$CComments,"") | Out-File $File -Force
}
Simply run the following to fix the json file:
Remove-Comments .\test.json
Now test.json file looks like this:
{
"Accounts": {
"Users": [
{
"jdoe": {
"givenName": "John",
"surname": "Doe",
"department": "Finance"
},
"jdoe2": {
"givenName": "Jane",
"surname": "Doe",
"department": "Marketing"
},
"asmith": {
"givenName": "Alice",
"surname": "Smith",
"department": "IT"
}
}
]
}
}
Now that the comments are gone the JSON can be converted into a PSCustomObject using the following just like in PS7:
$myJson = Get-Content .\test.json -Raw | ConvertFrom-Json
PowerShell is a great tool to use for manipulating JSON which is used throughout Azure.
Have fun scripting!
Additional reading:
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
%3CLINGO-SUB%20id%3D%22lingo-sub-2768721%22%20slang%3D%22en-US%22%3EParsing%20JSON%20with%20PowerShell%3C%2FLINGO-SUB%3E%3CLINGO-BODY%20id%3D%22lingo-body-2768721%22%20slang%3D%22en-US%22%3E%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EQ%3A%20I%20try%20to%20parse%20my%20JSON%20with%20the%20handy%20dandy%20%E2%80%9CConvertFrom-JSON%E2%80%9D%20cmdlet%20but%20it%20only%20works%20in%20PowerShell%207%2C%20not%20in%20my%20good%20old%20PowerShell%205.1.%26nbsp%3B%20How%20do%20I%20get%20it%20to%20work%20everywhere%3F%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EA%3A%20PS%207%20parses%20JSON%20a%20little%20differently%20than%20PS%205.1%20by%20ignoring%20comments%20and%20accepting%20less%20than%20ideal%20JSON%20so%20you%20need%20to%20clean%20up%20your%20JSON%20for%20PS%205.1.%26nbsp%3B%20Use%20the%20function%20below%20to%20do%20it%20for%20you.%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EJava%20Script%20Object%20Notation%20(JSON)%20is%20a%20popular%20format%20these%20days%20for%20sending%20and%20receiving%20data%20with%20Azure.%26nbsp%3B%26nbsp%3B%20JSON%20is%20used%20for%20sending%20and%20receiving%20data%20using%20Azure%20REST%20API%2C%20deploying%20resources%20to%20Azure%20using%20ARM%20templates%2C%20configure%20governance%20in%20Azure%20using%20Azure%20Policy%2C%20and%20much%20more.%20PowerShell%20is%20a%20great%20tool%20for%20creating%20and%20modifying%20JSON.%20PowerShell%207.1%20is%20my%20preferred%20solution%20since%20it%20has%20additional%20capabilities%20however%20sometimes%20we%20still%20need%20good%20old%20PS%205.1%20so%20it%20is%20nice%20to%20be%20able%20to%20accomplish%20anything%20in%20either%20version.%26nbsp%3B%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CH6%20id%3D%22toc-hId-1050727603%22%20id%3D%22toc-hId-1050727765%22%3E%3CSPAN%20data-contrast%3D%22auto%22%3EDisclaimer%3A%26nbsp%3B%3C%2FSPAN%3E%3CSPAN%20data-contrast%3D%22none%22%3EThe%20sample%20scripts%20are%20not%20supported%20under%20any%20Microsoft%20standard%20support%20program%20or%20service.%20The%20sample%20scripts%20are%20provided%20AS%20IS%20without%20warranty%20of%20any%20kind.%20Microsoft%20further%20disclaims%20all%20implied%20warranties%20including%2C%20without%20limitation%2C%20any%20implied%20warranties%20of%20merchantability%20or%20of%20fitness%20for%20a%20particular%20purpose.%20The%20entire%20risk%20arising%20out%20of%20the%20use%20or%20performance%20of%20the%20sample%20scripts%20and%20documentation%20remains%20with%20you.%20In%20no%20event%20shall%20Microsoft%2C%20its%20authors%2C%20or%20anyone%20else%20involved%20in%20the%20creation%2C%20production%2C%20or%20delivery%20of%20the%20scripts%20be%20liable%20for%20any%20damages%20whatsoever%20(including%2C%20without%20limitation%2C%20damages%20for%20loss%20of%20business%20profits%2C%20business%20interruption%2C%20loss%20of%20business%20information%2C%20or%20other%20pecuniary%20loss)%20arising%20out%20of%20the%20use%20of%20or%20inability%20to%20use%20the%20sample%20scripts%20or%20documentation%2C%20even%20if%20Microsoft%20has%20been%20advised%20of%20the%20possibility%20of%20such%20damages.%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FH6%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EFirst%2C%20here%20is%20a%20sample%20file%20I%E2%80%99ll%20use%20for%20the%20example%26nbsp%3Bor%20you%20can%20download%20it%20from%26nbsp%3B%3C%2FSPAN%3E%3CA%20href%3D%22https%3A%2F%2Fgithub.com%2FPaulHCode%2FBlogBits%2Fblob%2Fmain%2Ftest.json%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3Ehere%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-contrast%3D%22auto%22%3E.%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CPRE%20class%3D%22lia-code-sample%20language-json%22%3E%3CCODE%3E%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22Accounts%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22Users%22%3A%E2%80%AF%5B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22jdoe%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22John%22%2C%E2%80%AF%2F%2FOut%E2%80%AFon%E2%80%AFleave%E2%80%AFthis%E2%80%AFmonth%E2%80%AF%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Doe%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22Finance%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22jdoe2%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22Jane%22%2C%E2%80%AF%2F%2FDepartment%E2%80%AFhead%E2%80%AF%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Doe%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22Marketing%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22asmith%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22Alice%22%2C%E2%80%AF%2F*%E2%80%AFSits%E2%80%AFin%E2%80%AFthe%E2%80%AFcorner%E2%80%AFoffice%E2%80%AF*%2F%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Smith%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22IT%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%5D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%7D%20%3C%2FCODE%3E%3C%2FPRE%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EAlice%20got%20promoted%20so%20we%20need%20to%20change%20her%20department%20to%20Senior%20Leadership.%26nbsp%3B%20PowerShell%20makes%20it%20easy%20to%20modify%20JSON%20by%20converting%20JSON%20to%20a%26nbsp%3BPSCustomObject.%26nbsp%3B%20The%20object%20can%20then%20be%20modified%20easily%20like%20any%20other%20object.%26nbsp%3B%20The%20object%20can%20then%20be%20exported%20back%20out%20using%26nbsp%3BConvertTo-Json.%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CPRE%20class%3D%22lia-code-sample%20language-powershell%22%3E%3CCODE%3E%24myJson%20%3D%20Get-Content%20.%5Ctest.json%20-Raw%20%7C%20ConvertFrom-Json%20%0A%24myJson.Accounts.Users.asmith.department%20%3D%20%22Senior%20Leadership%22%20%0A%24myJson%20%7C%20ConvertTo-Json%20-Depth%204%20%7C%20Out-File%20.%5Ctest.json%20%20%3C%2FCODE%3E%3C%2FPRE%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EThis%20gives%20a%26nbsp%3Btest.json%26nbsp%3Bfile%20that%20accomplished%20exactly%20what%20we%20wanted%3A%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CPRE%20class%3D%22lia-code-sample%20language-json%22%3E%3CCODE%3E%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22Accounts%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22Users%22%3A%E2%80%AF%5B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22jdoe%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22John%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Doe%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22Finance%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22jdoe2%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22Jane%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Doe%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22Marketing%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22asmith%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22Alice%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Smith%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22Senior%E2%80%AFLeadership%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%5D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%7D%3C%2FCODE%3E%3C%2FPRE%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A0%2C%26quot%3B335559740%26quot%3B%3A240%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3CSPAN%20data-contrast%3D%22auto%22%3ENow%20if%20we%E2%80%99re%20on%20a%20computer%20without%20PowerShell%207.1%20we%20try%20to%20run%20the%20same%20command%20in%20PowerShell%205.1%20but%20it%20fails!%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CPRE%20class%3D%22lia-code-sample%20language-powershell%22%3E%3CCODE%3EPS%26gt%3B%20%24a%20%3D%20gc%20.%5Ctest.json%20-Raw%20%7C%20ConvertFrom-Json%20%0AConvertFrom-Json%20%3A%20Invalid%20object%20passed%20in%2C%20'%3A'%20or%20'%7D'%20expected.%20(17161)%3A%7B%20%0A%3CTHE%20entire%3D%22%22%20contents%3D%22%22%20of%3D%22%22%20the%3D%22%22%20json%3D%22%22%20file%3D%22%22%20listed%3D%22%22%20here%3D%22%22%3E%20%0A%7D%20%0AAt%20line%3A1%20char%3A28%20%0A%2B%20%24a%20%3D%20gc%20.%5Ctest.json%20-Raw%20%7C%20ConvertFrom-Json%20%0A%2B%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20~~~~~~~~~~~~~~~~%20%0A%20%20%20%20%2B%20CategoryInfo%20%20%20%20%20%20%20%20%20%20%3A%20NotSpecified%3A%20(%3A)%20%5BConvertFrom-Json%5D%2C%20ArgumentException%20%0A%20%20%20%20%2B%20FullyQualifiedErrorId%20%3A%20System.ArgumentException%2CMicrosoft.PowerShell.Commands.ConvertFromJsonCommand%20%20%3C%2FTHE%3E%3C%2FCODE%3E%3C%2FPRE%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EComments%20will%20prevent%26nbsp%3BConvertFrom-Json%20from%20working%20properly%20in%20PowerShell%205.1.%26nbsp%3B%20I%20like%20to%20use%20this%20simple%20function%20to%20fix%20my%20JSON%20for%20me.%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CPRE%20class%3D%22lia-code-sample%20language-powershell%22%3E%3CCODE%3EFunction%20Remove-Comments%7B%20%0A%20%20%20%20param(%20%0A%20%20%20%20%20%20%20%20%5BCmdletBinding()%5D%20%0A%20%20%20%20%20%20%20%20%5BParameter(Mandatory%3D%24true%2CValueFromPipelineByPropertyName%3D%24true%2CPosition%3D0)%5D%20%0A%20%20%20%20%20%20%20%20%5Bstring%5D%20%0A%20%20%20%20%20%20%20%20%5BValidateScript(%7Btest-path%20%24_%7D)%5D%20%0A%20%20%20%20%20%20%20%20%24File%20%0A%20%20%20%20)%20%0A%20%20%20%20%24CComments%20%3D%20%22(%3Fs)%2F%5C%5C*.*%3F%5C%5C*%2F%22%20%0A%20%20%20%20%24content%20%3D%20Get-Content%20%24File%20-Raw%20%0A%20%20%20%20%5Bregex%5D%3A%3AReplace(%24content%2C%24CComments%2C%22%22)%20%7C%20Out-File%20%24File%20-Force%20%0A%7D%20%3C%2FCODE%3E%3C%2FPRE%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3ESimply%20run%20the%20following%20to%20fix%20the%20json%20file%3A%26nbsp%3B%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CPRE%20class%3D%22lia-code-sample%20language-powershell%22%3E%3CCODE%3ERemove-Comments%20.%5Ctest.json%20%3C%2FCODE%3E%3C%2FPRE%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3ENow%26nbsp%3Btest.json%26nbsp%3Bfile%20looks%20like%20this%3A%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CPRE%20class%3D%22lia-code-sample%20language-json%22%3E%3CCODE%3E%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22Accounts%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22Users%22%3A%E2%80%AF%5B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22jdoe%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22John%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Doe%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22Finance%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22jdoe2%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22Jane%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Doe%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22Marketing%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22asmith%22%3A%E2%80%AF%7B%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22givenName%22%3A%E2%80%AF%22Alice%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22surname%22%3A%E2%80%AF%22Smith%22%2C%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%22department%22%3A%E2%80%AF%22IT%22%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%5D%20%0A%E2%80%AF%E2%80%AF%E2%80%AF%E2%80%AF%7D%20%0A%7D%20%3C%2FCODE%3E%3C%2FPRE%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A0%2C%26quot%3B335559740%26quot%3B%3A240%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3CSPAN%20data-contrast%3D%22auto%22%3ENow%20that%20the%20comments%20are%20gone%20the%20JSON%20can%20be%20converted%20into%20a%26nbsp%3BPSCustomObject%26nbsp%3Busing%20the%20following%20just%20like%20in%20PS7%3A%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CPRE%20class%3D%22lia-code-sample%20language-powershell%22%3E%3CCODE%3E%24myJson%20%3D%20Get-Content%20.%5Ctest.json%20-Raw%20%7C%20ConvertFrom-Json%20%20%3C%2FCODE%3E%3C%2FPRE%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%26nbsp%3B%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EPowerShell%20is%20a%20great%20tool%20to%20use%20for%20manipulating%20JSON%20which%20is%20used%20throughout%20Azure.%26nbsp%3B%26nbsp%3B%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EHave%20fun%20scripting!%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CP%3E%3CSPAN%20data-contrast%3D%22auto%22%3EAdditional%20reading%3A%26nbsp%3B%3C%2FSPAN%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FP%3E%0A%3CUL%3E%0A%3CLI%20data-leveltext%3D%22-%22%20data-font%3D%22Calibri%22%20data-listid%3D%221%22%20aria-setsize%3D%22-1%22%20data-aria-posinset%3D%221%22%20data-aria-level%3D%221%22%3E%3CSPAN%20data-contrast%3D%22auto%22%3E7.1%3A%26nbsp%3B%3C%2FSPAN%3E%3CA%20href%3D%22https%3A%2F%2Fdocs.microsoft.com%2Fen-us%2Fpowershell%2Fmodule%2Fmicrosoft.powershell.utility%2Fconvertfrom-json%3Fview%3Dpowershell-7.1%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3EConvertFrom-Json%20(Microsoft.PowerShell.Utility)%20-%20PowerShell%20%7C%20Microsoft%20Docs%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B134233279%26quot%3B%3Atrue%2C%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FLI%3E%0A%3CLI%20data-leveltext%3D%22-%22%20data-font%3D%22Calibri%22%20data-listid%3D%221%22%20aria-setsize%3D%22-1%22%20data-aria-posinset%3D%222%22%20data-aria-level%3D%221%22%3E%3CSPAN%20data-contrast%3D%22auto%22%3E5.1%3A%26nbsp%3B%3C%2FSPAN%3E%3CA%20href%3D%22https%3A%2F%2Fdocs.microsoft.com%2Fen-us%2Fpowershell%2Fmodule%2Fmicrosoft.powershell.utility%2Fconvertfrom-json%3Fview%3Dpowershell-5.1%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3EConvertFrom-Json%20(Microsoft.PowerShell.Utility)%20-%20PowerShell%20%7C%20Microsoft%20Docs%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B134233279%26quot%3B%3Atrue%2C%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FLI%3E%0A%3CLI%20data-leveltext%3D%22-%22%20data-font%3D%22Calibri%22%20data-listid%3D%221%22%20aria-setsize%3D%22-1%22%20data-aria-posinset%3D%223%22%20data-aria-level%3D%221%22%3E%3CSPAN%20data-contrast%3D%22auto%22%3E7.1%3A%26nbsp%3B%3C%2FSPAN%3E%3CA%20href%3D%22https%3A%2F%2Fdocs.microsoft.com%2Fen-us%2Fpowershell%2Fmodule%2Fmicrosoft.powershell.utility%2Fconvertto-json%3Fview%3Dpowershell-7.1%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3EConvertTo-Json%20(Microsoft.PowerShell.Utility)%20-%20PowerShell%20%7C%20Microsoft%20Docs%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B134233279%26quot%3B%3Atrue%2C%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FLI%3E%0A%3CLI%20data-leveltext%3D%22-%22%20data-font%3D%22Calibri%22%20data-listid%3D%221%22%20aria-setsize%3D%22-1%22%20data-aria-posinset%3D%224%22%20data-aria-level%3D%221%22%3E%3CSPAN%20data-contrast%3D%22auto%22%3E5.1%3A%26nbsp%3B%3C%2FSPAN%3E%3CA%20href%3D%22https%3A%2F%2Fdocs.microsoft.com%2Fen-us%2Fpowershell%2Fmodule%2Fmicrosoft.powershell.utility%2Fconvertto-json%3Fview%3Dpowershell-5.1%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3EConvertTo-Json%20(Microsoft.PowerShell.Utility)%20-%20PowerShell%20%7C%20Microsoft%20Docs%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B134233279%26quot%3B%3Atrue%2C%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FLI%3E%0A%3C%2FUL%3E%0A%3CUL%3E%0A%3CLI%20data-leveltext%3D%22-%22%20data-font%3D%22Calibri%22%20data-listid%3D%221%22%20aria-setsize%3D%22-1%22%20data-aria-posinset%3D%221%22%20data-aria-level%3D%221%22%3E%3CSPAN%20data-contrast%3D%22auto%22%3E7.1%3A%26nbsp%3B%3C%2FSPAN%3E%3CA%20href%3D%22https%3A%2F%2Fdocs.microsoft.com%2Fen-us%2Fpowershell%2Fmodule%2Fmicrosoft.powershell.utility%2Ftest-json%3Fview%3Dpowershell-7.1%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3ETest-Json%20(Microsoft.PowerShell.Utility)%20-%20PowerShell%20%7C%20Microsoft%20Docs%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B134233279%26quot%3B%3Atrue%2C%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FLI%3E%0A%3CLI%20data-leveltext%3D%22-%22%20data-font%3D%22Calibri%22%20data-listid%3D%221%22%20aria-setsize%3D%22-1%22%20data-aria-posinset%3D%222%22%20data-aria-level%3D%221%22%3E%3CA%20href%3D%22https%3A%2F%2Fdocs.microsoft.com%2Fen-us%2Frest%2Fapi%2Fazure%2F%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3EAzure%20REST%20API%20reference%20documentation%20%7C%20Microsoft%20Docs%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B134233279%26quot%3B%3Atrue%2C%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FLI%3E%0A%3CLI%20data-leveltext%3D%22-%22%20data-font%3D%22Calibri%22%20data-listid%3D%221%22%20aria-setsize%3D%22-1%22%20data-aria-posinset%3D%223%22%20data-aria-level%3D%221%22%3E%3CA%20href%3D%22https%3A%2F%2Fdocs.microsoft.com%2Fen-us%2Fazure%2Fgovernance%2Fpolicy%2Foverview%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3EOverview%20of%20Azure%20Policy%20-%20Azure%20Policy%20%7C%20Microsoft%20Docs%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B134233279%26quot%3B%3Atrue%2C%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FLI%3E%0A%3CLI%20data-leveltext%3D%22-%22%20data-font%3D%22Calibri%22%20data-listid%3D%221%22%20aria-setsize%3D%22-1%22%20data-aria-posinset%3D%224%22%20data-aria-level%3D%221%22%3E%3CA%20href%3D%22https%3A%2F%2Fdocs.microsoft.com%2Fen-us%2Fazure%2Fazure-resource-manager%2Ftemplates%2F%22%20target%3D%22_blank%22%20rel%3D%22noopener%20noreferrer%22%3E%3CSPAN%20data-contrast%3D%22none%22%3EARM%20template%20documentation%20%7C%20Microsoft%20Docs%3C%2FSPAN%3E%3C%2FA%3E%3CSPAN%20data-ccp-props%3D%22%7B%26quot%3B134233279%26quot%3B%3Atrue%2C%26quot%3B201341983%26quot%3B%3A0%2C%26quot%3B335559739%26quot%3B%3A160%2C%26quot%3B335559740%26quot%3B%3A259%7D%22%3E%26nbsp%3B%3C%2FSPAN%3E%3C%2FLI%3E%0A%3C%2FUL%3E%3C%2FLINGO-BODY%3E%3CLINGO-TEASER%20id%3D%22lingo-teaser-2768721%22%20slang%3D%22en-US%22%3E%3CP%3E%3CSPAN%20class%3D%22TextRun%20SCXW83336271%20BCX8%22%20data-contrast%3D%22auto%22%3E%3CSPAN%20class%3D%22NormalTextRun%20SCXW83336271%20BCX8%22%3EQ%3A%20I%20try%20to%20parse%20my%20JSON%20with%20the%20handy%20dandy%20%E2%80%9C%3C%2FSPAN%3E%3CSPAN%20class%3D%22NormalTextRun%20SpellingErrorV2%20SCXW83336271%20BCX8%22%3EConvertFrom%3C%2FSPAN%3E%3CSPAN%20class%3D%22NormalTextRun%20SCXW83336271%20BCX8%22%3E-JSON%E2%80%9D%20cmdlet%20but%20it%20only%20works%20in%20PowerShell%207%2C%20not%20in%20my%20good%20old%20PowerShell%205.1.%26nbsp%3B%20How%20do%20I%20get%20it%20to%20work%20everywhere%3F%3C%2FSPAN%3E%3C%2FSPAN%3E%3C%2FP%3E%3C%2FLINGO-TEASER%3E%3CLINGO-LABS%20id%3D%22lingo-labs-2768721%22%20slang%3D%22en-US%22%3E%3CLINGO-LABEL%3EPaulHarrison%3C%2FLINGO-LABEL%3E%3C%2FLINGO-LABS%3E
Sep 21 2021 10:14 AM
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK