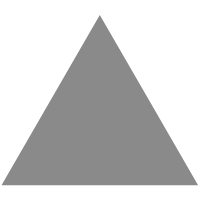
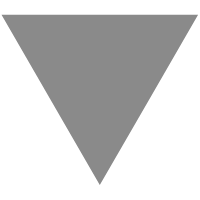
Professional C# and .NET – 2021 Edition
source link: https://csharp.christiannagel.com/2021/09/13/professionalcsharp2021/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
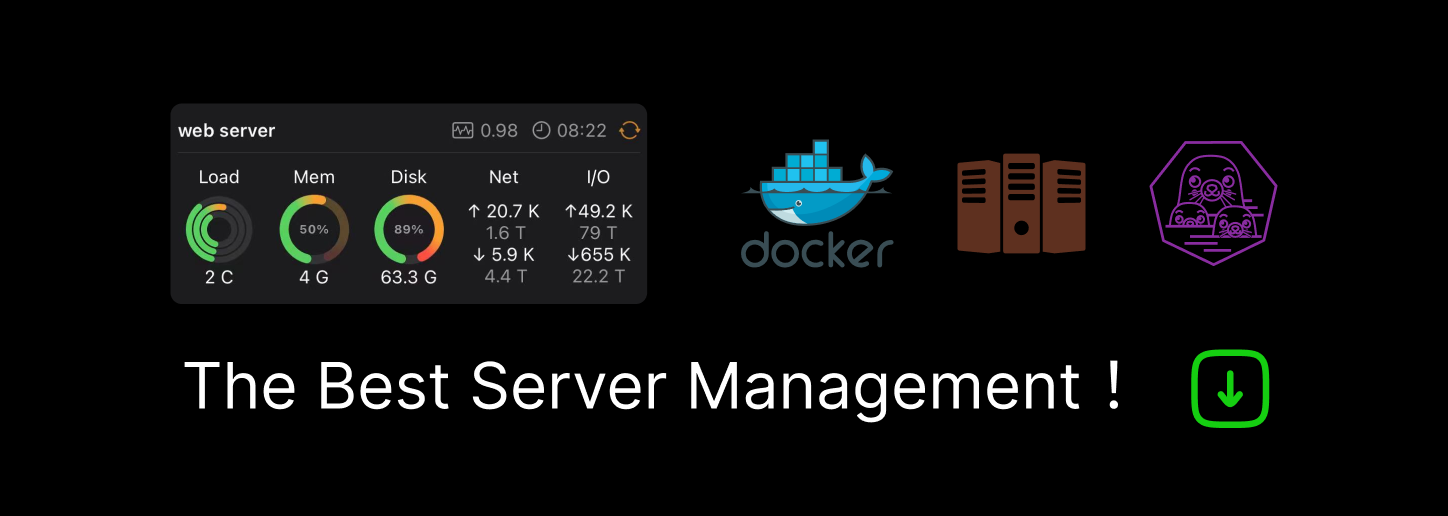
Professional C# and .NET – 2021 Edition – csharp.christiannagel.comSkip to content
The new edition of my book is available – covering .NET, C#, WinUI, ASP.NET Core, EF Core, and more! Compared to the previous edition, it not only covers the newest editions of C# and .NET, you don’t have to carry a book with 1500 pages – the number of pages have been reduced – but I made sure that all the important topics are covered, you shouldn’t miss anything important topics needed to develop applications with .NET, C#, and Microsoft Azure.
This book is great for developers moving from other programming languages to C# and .NET, but also for developers already working with .NET to read information on new features.
Let’s get into the details on what’s in this book!
Part I – The Language
The first part of the book covers all the aspects of the C# programming language – including new features such as top level statements, records, pattern matching, source generators, and more. All the code samples use nullable reference types.
Chapter 1: .NET Applications and Tools
This chapter covers using the tools to create .NET applications including the .NET CLI.
Chapter 2: Core C#
Chapter 2 dives into core C# features and gives you details on top-level statements and information on declaration of variables and data types. This chapter covers target-typed new expressions, explains nullable reference types, and defines a program flow that includes the new switch expression.
Chapter 3: Classes, Records, Structs, and Tuples
Chapter 3 gives you information on the differences between reference and value types, and covers features of C# 9 records. Here information is covered about members of a type including methods, properties, fields, and more.
Chapter 4: Object-Oriented Programming in C#
This chapter goes into details on object-oriented techniques with C# and covers inheritance with classes, using interfaces, and how you can use inheritance with records. It helps to already know object-orientation before reading this chapter. This chapter covers dependency injection with interfaces.
Chapter 5: Operators and Casts
Chapter 5 explaines the C# operations, and you also learn how to overload standard operations for custom types.
Chapter 6: Arrays
The chapter Arrays doesn’t stop with simple arrays; you learn using multidimensional arrays and jagged arrays, use the Span
type to access arrays, and use the new index and range operators.
Chapter 7: Delegates, Lambdas, and Events
Chapter 7 covers .NET pointers to methods, lambda expresions with closures, and .NET events.
Chapter 8: Collections
The Collections chapter dives into different kind of collections, such as lists, queues, stacks, dictionaries, and immutable collections. This chapter also gives you the information you need to decide which collection to use in which scenario.
Chapter 9: Language Integrated Query
Langugae Integrated Query (LINQ) gives you the C# integrated query features to query data from your collections – using the query and the method syntax. You also learn how to use multiple CPU cores with a query and what’s behind expression trees that are used when you use LINQ to access your database with Entity Framework Core.
Chapter 10: Errors and Exceptions
Errors and Exceptions covers how you should deal with errors, throw and catch exceptions, and filter exceptions when catching them.
Chapter 11: Tasks and Asynchronous Programming
Tasks and Asynchronous Programming shows the C# keywords async
and await
in action— not only with the task-based async pattern but also with async streams.
Chapter 12: Reflection, Metadata, and Source Generators
Reflection, Metadata, and Source Generators covers using and reading attributes with C#. The attributes will not just be read using reflection, but you’ll also see the functionality of source generators that allow creating source code during compile time.
Chapter 13: Managed and Unmanaged Memory
Managed and Unmanaged Memory is the last chapter of Part I, which not only shows
using the IDisposable
interface with the using
statement and the new using
declaration but also
demonstrates using the Span
type with managed and unmanaged memory. You can read about using
Platform Invoke both with Windows and with Linux environments
Part II – Libraries
Part II starts with creating custom libraries and NuGet packages, but the major topics covered with Part II are for using .NET core libraries that are important for all application types.
Chapter 14: Libraries, Assemblies, Packages, and NuGet
Libraries, Assemblies, Packages, and NuGet explains the differences between assemblies and NuGet packages. In this chapter, you learn how to create NuGet packages and are introduced to a new C# feature, module initializers, which allow you to run initial code in a library
Chapter 15: Dependency Injection and Configuration
Dependency Injection and Configuration gives detail about how the Host
class is used to configure a dependency injection container and the built-in options to retrieve configuration information from a .NET application with different configuration providers, including Azure App Configuration and user secrets.
Chapter 16: Diagnostics and Metrics
Diagnostics and Metrics continues using the Host class to configure logging options. You also learn about reading metric information that’s offered from some NET providers, using Visual Studio App Center, and extending logging for distributed tracing with OpenTelemetry.
Chapter 17: Parallel Programming
Parallel Programming covers myriad features available with .NET for parallelization and synchronization. Chapter 11 shows the core functionality of the Task
class. In Chapter 17, more of the
Task
class is shown, such as forming task hierarchies and using value tasks. The chapter goes into issues of parallel programming such as race conditions and deadlocks, and for synchronization, you learn
about different features available with the lock
keyword, the Monitor
, SpinLock
, Mutex
, Semaphore
classes, and more.
Chapter 18: Files and Streams
Files and Streams not only covers reading and writing from the file system with new stream APIs that allow using the Span
type but also covers the new .NET JSON serializer with classes in the System.Text.Json
namespace.
Chapter 19: Networking
In chapter Networking you learn about foundational classes for network programming, such as the Socket
class and how to create applications using TCP and UDP. You also use the HttpClient
factory pattern to create HttpClient
objects with automatic retries if transient errors occur.
Chapter 20: Security
Security gives you information about cryptography classes for encrypting data, explains how to use the new Microsoft.Identity
platform for user authentication, and provides information on web security and what you need to be aware of with encoding issues as well as cross-site request forgery attacks
Chapter 21: Entity Framework Core
Entity Framework Core covers reading and writing data from a database—including the many features offered from EF Core, such as shadow properties, global query filters, many-to-many relations, and what metric information is now offered by EF Core—and reading and writing to Azure Cosmos DB with EF Core
Chapter 22: Localization
With Localization you learn to localize applications using techniques that are important both for Windows and web applications.
Chapter 23: Tests
Tests covers creating unit tests, analyzing code coverage with the .NET CLI, using a mocking library when creating unit tests, and what features are offered by ASP.NET Core to create integration tests
Part III – Web Applications and Services
Part III of this book is dedicated to ASP.NET Core technologies for creating web applications and services, no matter whether you run these applications and services in your on-premises environment or in the cloud making use of Azure App Services, Azure Static Web Apps, or Azure Functions.
Chapter 24: ASP.NET Core
This chapter gives you the foundation of ASP.NET Core. Based on the dependency injection container you learned about in Part II, this chapter shows how ASP.NET Core makes use of middleware to add functionality to every HTTP request and define routes with ASP.NET Core endpoint routing.
Chapter 25: Services
Services dives into creating microservices using different technologies such as ASP.NET Core as well as using Azure Functions and gRPC for binary communication.
Chapter 26: Razor Pages and MVC
This chapter is about interacting with users with ASP.NET Core technologies. It covers Razor pages, Razor views, and functionality such as tag helpers and view components.
Chapter 27: Blazor
Blazor is about the newest enhancement of ASP.NET Core with Razor components, which allows you to implement C# code running either on the server or in the client using WebAssembly. You learn about the differences between Blazor Server and Blazor WebAssembly, what the restrictions are with these technologies, and the built-in components available.
Chapter 28: SignalR
This chapter covers the real-time functionality available with ASP.NET Core to send information to a group of clients and how you can use C# async streams with SignalR.
Part IV: Apps
Part IV of this book is dedicated to XAML code and creating Windows applications with the native UI platform for Windows 10: WinUI. Much of the information you get here can also be applied to WPF applications and to .NET MAUI and developing XAML-based applications for mobile platforms.
Chapter 29: Windows Apps
Windows Apps gives you foundational information on XAML, including dependency properties and attached properties. You learn how to create custom markup extensions and about the control categories available with WinUI, including advanced techniques such as adaptive triggers and deferred loading.
Chapter 30: Patterns with XAML Apps
This chapter gives you the information you need to use the MVVM pattern and how you can share as much code as possible between different XAML-based technologies such as WinUI, WPF, and .NET MAUI.
Chapter 31: Styling Windows Apps
Styling Windows Apps explains XAML shapes and geometry elements, dives into styles and control templates, gives you information on creating animations, and explains how you can use the Visual State Manager with your XAML-based applications.
The Source Code
The complete source code is available both on Wiley, as well as on GitHub. The source code on GitHub is updated more often than the source code on the Wiley website.
Issues and Discussions
In case you have some questions on the samples, please write messages to discussions. Looking forward to see questions and probably also answers from readers. In case you find problems with source code samples, report an issue. I will try to answer/fix it as fast as my time allows. You’re also free to submit a pull request with changes on code samples if you find some issues (the first reader who did a pull request was @LuohuaRain – thanks for that!).
It would be great if you Star the GitHub repo. You are also welcome to fork it to your GitHub account to easier work with the existing code samples.
Updates
With C# and .NET we’ll have continuous updates, new features are added to the C# programming language and to libraries. The code samples will be updated as well. Before C# 10 and .NET 6 is released, you’ll find source code updates in dotnet6 branches. After .NET 6 is released, the main branch will contain C# 10 and .NET 6 code samples. The code samples as written in the printed book will stay to be available in the dotnet5 branch.
New code samples will be explained in my blog. Every book chapter has a readme file. This file will contain information to updated and new code samples, including links to blog articles.
Buying Professional C# and .NET – 2021 Edition
You can buy the e-book or print edition of the book directly from Wiley. Of course you can buy the book from Amazon for Kindle or a Paperback edition and many other distributors!
Enjoy reading the book and checking the code samples, learning C# and .NET!
Christian
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK