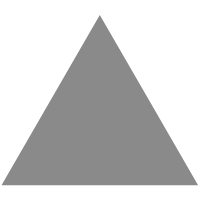
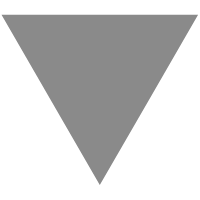
Arrow functions don’t have access to the arguments object as regular functions
source link: http://www.js-craft.io/blog/arrow-functions-dont-have-access-to-the-arguments-object-as-regular-functions/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
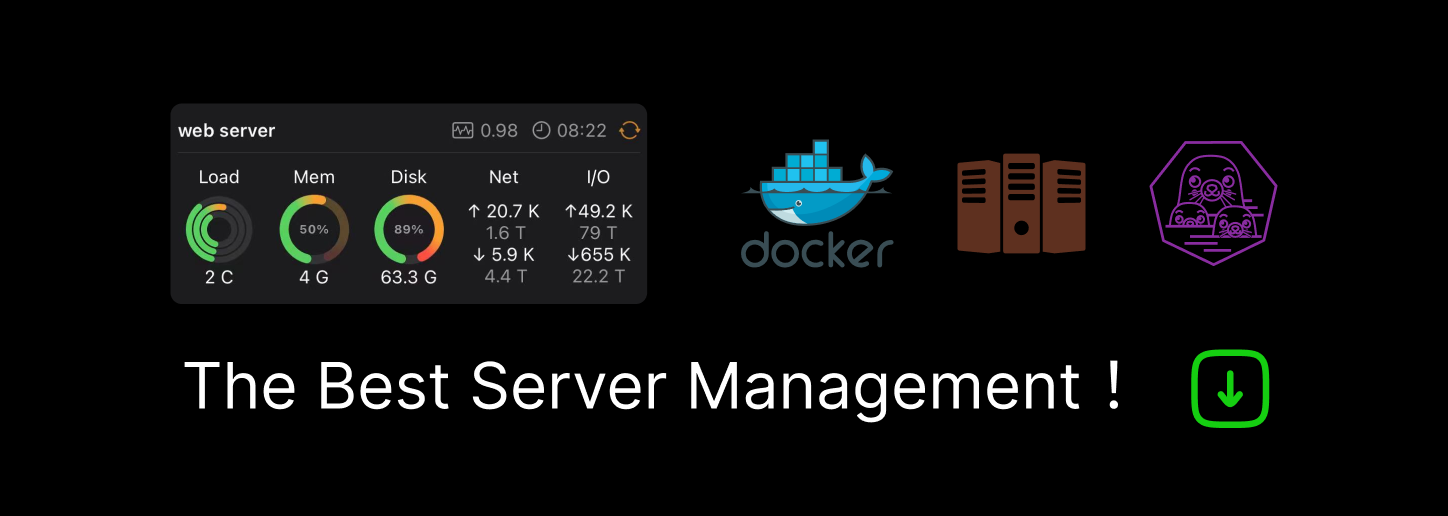
Arrow functions don’t have access to the arguments object as regular functions
While with a normal function you can use the arguments implicit object to see the given params:
function myFunction() {
console.log(arguments);
}
myFunction('a', 'b');
// logs { 0: 'a', 1: 'b', length: 2 }
In an arrow function this will end up with an unexpected result:
function myFunction() {
const arrowF = () => console.log(arguments);
arrowF('c', 'd');
}
myRegularFunction('a', 'b');
// will log { 0: 'a', 1: 'b', length: 2 }
// insead of the expected { 0: 'c', 1: 'd', length: 2 }
This happens because of the fact that that arrow functions don't have an arguments
object. They also don't have their own this context and therefore the arguments
is the one from the myRegularFunction
context.
However, we can use a rest parameter to spread the arguments in an arrow function:
function myRegularFunction() {
const arrowF = (...args) => console.log(args);
arrowF('c', 'd');
}
myRegularFunction('a', 'b');
// logs ['c', 'd']
And we can even make some cool stuff like combining regular parameters with a rest parameter:
function multiplyTheSum(multiplier, ...numbers) {
console.log(multiplier); // 2
console.log(numbers); // [10, 20, 30]
const sum = numbers.reduce((s, n) => s + n);
return multiplier * sum;
}
multiplyTheSum(2, 10, 20, 30); // => 120
Btw, if you are interested you can read more also about the function declarations vs the function expressions.
I hope you have enjoyed this article and if you would like to get more articles about React and frontend development you can always sign up for my email list.
Newsletter subscribe:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK