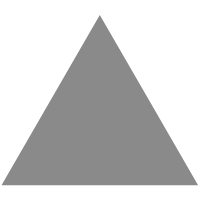
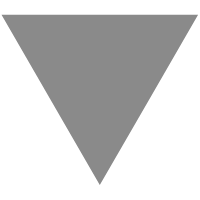
How to Store Data in Two Different Databases Simultaneously with Axios and APIs
source link: https://hackernoon.com/how-to-store-data-in-two-different-databases-simultaneously-with-axios-and-apis
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
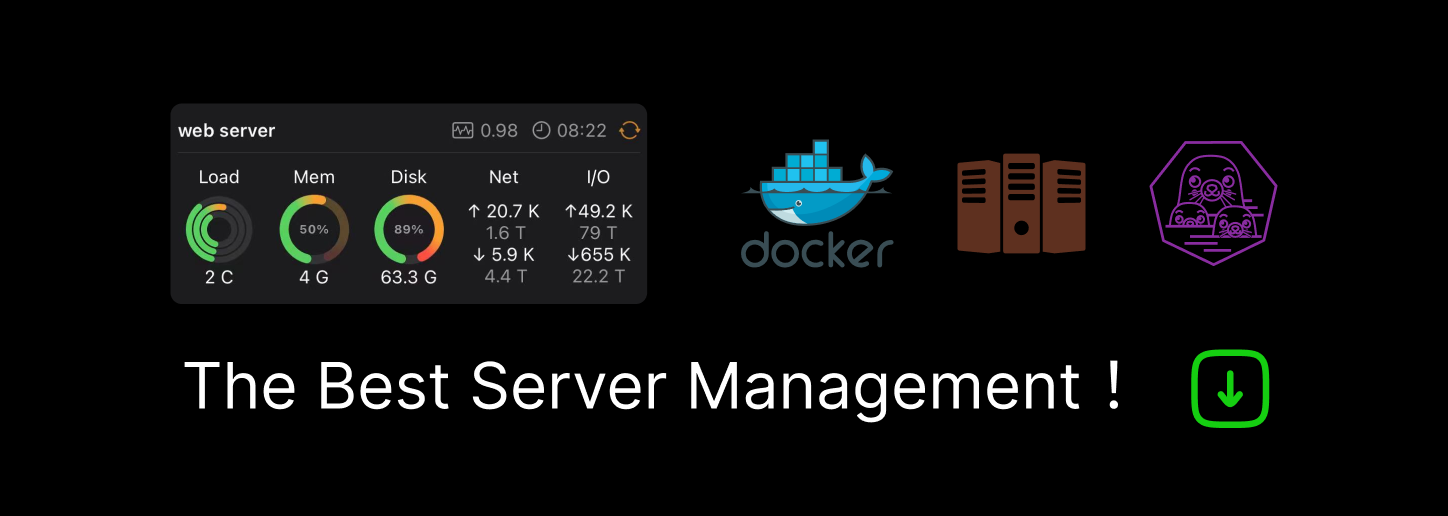
@sankalp1122Sankalp Swami
I m a self learnt Node JS Developer from India.
Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that runs on the V8 engine and executes JavaScript code outside the web. When it comes to scalability, Node.js is the best in the business. Working on Node.js for me is as beautiful as watching this man.
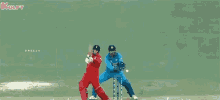
Dhoni
What is Axios?
Axios is a javascript library that is used to make HTTP requests from browsers and node.js. Axios requests and provides responses in JSON data. It intercepts requests and responses.
How to install Axios:
$ npm install axios
The Scene
When I was working for a startup as a Node.js Dev, there was a requirement for software that will save data to two different databases simultaneously. Actually, it was an Internet Service Providing Company and they were using some CRM application for which they were paying.
They wanted to develop the same application with advanced features but they didn’t want to stop using the other application for their own reasons. Now, I had a task to develop an API endpoint, which was for new customer creation and that data should be stored in two different databases i.e., in our own built application and in the one for which they were paying.
How I Did It
Of course, Axios came to the rescue. After googling things, I settled with Axios because it was the one with the most convenient way to do it. This was my POST
request controller.
add: (req, res) => {
mongoose.connect(
connUri,
{
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false,
useCreateIndex: true,
},
(err) => {
let result = {};
let status = 201;
if (!err) {
const firstName = req.body.firstName;
const lastName = req.body.lastName;
const email = req.body.email;
const lco = new LCO({
firstName,
lastName,
email
});
lco.save((err, lco) => {
if (!err) {
result.status = status;
result.result = lco;
} else {
status = 500;
result.status = status;
result.error = err;
}
res.status(status).send(result);
// Close the connection after saving
mongoose.connection.close();
});
} else {
status = 500;
result.status = status;
result.error = err;
res.status(status).send(result);
mongoose.connection.close();
}
}
);
}
So now my challenge was to store the same data in different databases simultaneously with the same POST request.
Let’s see how an Axios POST
request looks:
axios.post('{url}', {data}, {options});
You can add headers options to the Axios request. I added my headers options in config
object.
const config = {
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
}
After the config
object, I went for another object named data
, for passing it to the Axios POST
request.
const data = {
firstName: firstName,
lastName: lastName,
email: email
}
Now, it’s time for the Axios part. I added the Axios code snippet.
axios.post('/http://127.0.0.1:8000/api/v1/users', qs.stringify(data), config)
.then((result) => {
console.log(result);
}).catch((err) => {
console.log(err);
});
qs.stringify
converts JSON objects into query strings. Now My code looks like this -
add: (req, res) => {
mongoose.connect(
connUri,
{
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false,
useCreateIndex: true,
},
(err) => {
let result = {};
let status = 201;
if (!err) {
const config = {
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
}
const firstName = req.body.firstName;
const lastName = req.body.lastName;
const email = req.body.email;
const lco = new LCO({
firstName,
lastName,
email
});
const data = {
firstName: firstName,
lastName: lastName,
email: email
}
lco.save((err, lco) => {
if (!err) {
result.status = status;
result.result = lco;
axios.post('/http://127.0.0.1:8000/api/v1/users', qs.stringify(data), config)
.then((result) => {
console.log(result);
}).catch((err) => {
console.log(err);
});
} else {
status = 500;
result.status = status;
result.error = err;
}
res.status(status).send(result);
// Close the connection after saving
mongoose.connection.close();
});
} else {
status = 500;
result.status = status;
result.error = err;
res.status(status).send(result);
mongoose.connection.close();
}
}
);
}
Yep, this works very efficiently. I had a great experience learning Axios when working on this project. Thanks for reading, keep learning, Peace, and Bubbyeye.
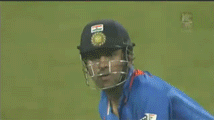
Dhoni
This article was first published at: https://dev.to/sankalpswami1122/how-i-used-axios-to-store-data-in-two-different-databases-simultaneously-51ia
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK