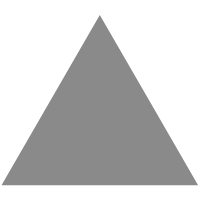
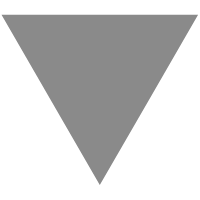
Best Practices for TDD in JavaScript/TypeScript
source link: https://stackoverflow.com/questions/69140302/best-practices-for-tdd-in-javascript-typescript/69141655#69141655
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Questions
- What is the correct/acceptable way to access all JavaScript/TypeScript functions in a module for unit testing?
- Is there a (good) reason why I shouldn't just export all my functions?
Context
Please forgive my ignorance; I have spent the majority of my professional career writing Python code using the Test Driven Development (TDD) approach. Now, I am finding myself learning ReactJS/TypeScript and figuring out how to implement unit tests, but I quickly found out that functions are only accessible if you export them. As most of you know, Python is very permissive; there really is no concept of privacy, so it's just a matter of importing the module and being respectful of what you have access to. But JavaScript only imports the functions of a module that have been explicitly exported, thus providing a modest barrier.
I've been told that it's not a great idea to export all my functions for testing, but I'm not aware of another way to actually be able to test them.
Examples
What I've been told is "correct/better":
Sample1.js:
const func1 = () => {
//code that does stuff
return "stuff";
};
const func2 = () => {
//code that does other stuff
return "otherStuff";
};
export { func1 };
Sample1.test.js:
// executed via Jest framework
import * as sample from "./Sample1.js"
describe("Unit Tests for Sample1", () => {
test("Unit Test - func1", () => {
// code that tests stuff
};
test("Unit Test - func2", () => {
// can't test func2 because it's not exported
};
};
What I'm doing to be able to test all functions:
Sample2.js:
const func1 = () => {
//code that does stuff
return "stuff";
};
const func2 = () => {
//code that does other stuff
return "otherStuff";
};
export { func1, func2 };
Sample2.test.js:
// executed via Jest framework
import * as sample from "./Sample2.js"
describe("Unit Tests for Sample2", () => {
test("Unit Test - func1", () => {
// code that tests stuff
};
test("Unit Test - func2", () => {
// code that tests other stuff because it's exported
};
};
For the Record
I've looked around on the internet and Stack Overflow specifically for standards and best practices, but I haven't found much that answers this specific question. The closest I found was this SO question, but it's not really what I'm after.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK