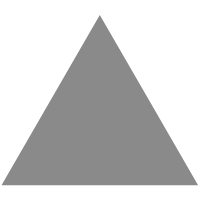
8
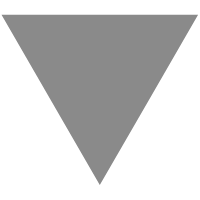
Android基础之最新正则表达式
source link: http://www.androidchina.net/8387.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
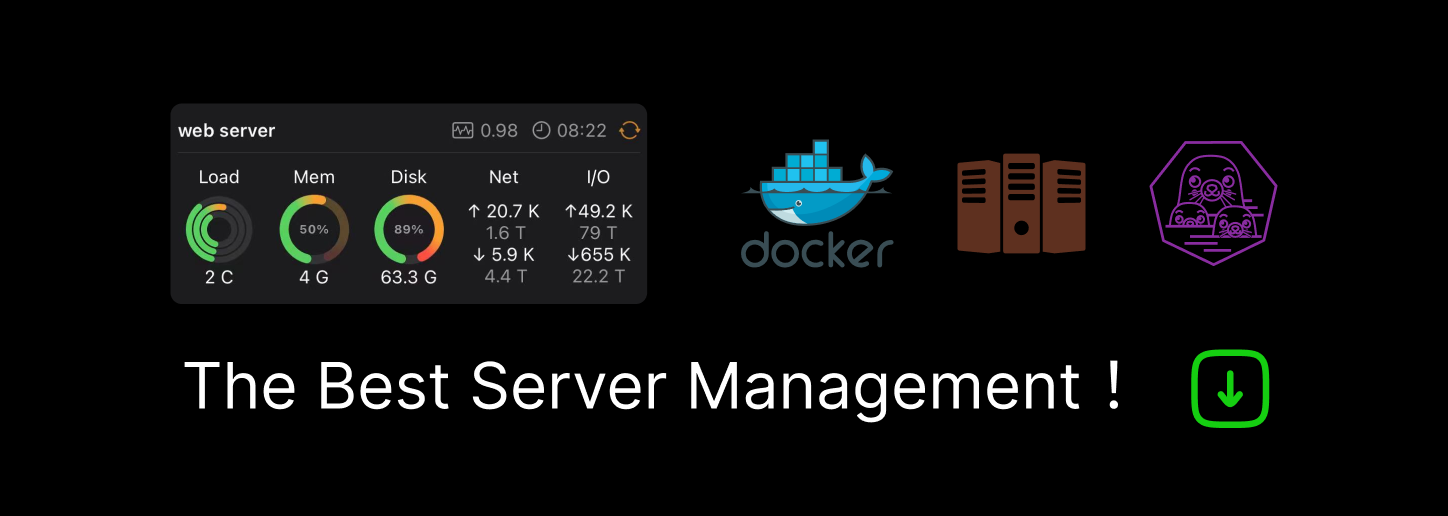
1 正则表达式解析json数据
public class RegularExpression {
public static void main() {
// String json = "{name:\"guan\",age:18}";
String json = "{name:\"guan1\",age:18,obj:{name:\"guan2\"}}";
//name:"guan"
//age:18
//\ 将下一个字符标记为一个特殊字符
//+ 匹配前面的子表达式一次或多次。例如,'zo+' 能匹配 "zo" 以及 "zoo",但不能匹配 "z"。
//* 匹配前面的子表达式零次或多次。
//\w 匹配包括下划线的任何单词字符。
//\d 匹配一个数字字符。等价于[0-9]。
//( ) 标记一个子表达式的开始和结束位置。
//^ 匹配输入字符串的开始位置。
//$ 匹配输入字符串的结束位置。
//x|y 匹配 x 或 y。
//\S 匹配任何非空白字符。
//\"\\w+\" 字符串属性{
// Pattern p = Pattern.compile("\\w+:(\"\\w+\"|\\d*)");
Pattern p = Pattern.compile("\\w+:(\"\\w+\"|\\d*|\\{\\S+\\})");
Matcher m = p.matcher(json);
while(m.find()){
String text = m.group();
int dotPos= text.indexOf(":");
String key = text.substring(0, dotPos);
String value = text.substring(dotPos+1, text.length());
//替换字符串的开始、结束的双引号 ^\"|\"$ == ^\\\"|\\\"$
value = value.replaceAll("^\"|\"$", "");
Log.e("Task", key);
Log.e("Task", value);
}
}
//测试结果
// E/Task: name
// E/Task: guan1
// E/Task: age
// E/Task: 18
// E/Task: obj
// E/Task: name
// E/Task: guan2
}
2 手机号正则表达式
(1) 手机号开头集合
176,177,178,
180,181,182,183,184,185,186,187,188。,189。
145,147
130,131,132,133,134,135,136,137, 138,139
150,151, 152,153,155,156,157,158,159,
(2) 正则表达式
/**
* 验证手机号码是否合法
*/
public static boolean validatePhoneNumber(String mobiles) {
String telRegex = "^((13[0-9])|(15[^4])|(18[0-9])|(17[0-8])|(147,145))\\d{8}$";
return !TextUtils.isEmpty(mobiles) && mobiles.matches(telRegex);
}
3 身份证号码正则表达式
/**
* 验证身份证号码是否合法
*/
public static boolean validateIDCardNumber(String number) {
return (number.length() == 15 && number.matches("^\\d{15}"))
|| (number.length() == 18 && (number.matches("^\\d{17}[x,X,\\d]")));
}
4 正则表达式匹配URL
(1) 判断是否是完整的域名
public static boolean isCompleteUrl(String text) {
Pattern p = Pattern.compile("((http|ftp|https)://)(([a-zA-Z0-9\\._-]+\\.[a-zA-Z]{2,6})|([0-9]{1,3}\\.[0-9]{1,3}\\.[0-9]{1,3}\\.[0-9]{1,3}))(:[0-9]{1,4})*(/[a-zA-Z0-9\\&%_\\./-~-]*)?", Pattern.CASE_INSENSITIVE);
Matcher matcher = p.matcher(text);
return matcher.find();
}
(2)判断是否是缺少前缀的域名
/**
* 是否是缺少前缀的域名
*/
public static boolean isHalfCompleteUrl(String text) {
Pattern p = Pattern.compile("(([a-zA-Z0-9\\._-]+\\.[a-zA-Z]{2,6})|([0-9]{1,3}\\.[0-9]{1,3}\\.[0-9]{1,3}\\.[0-9]{1,3}))(:[0-9]{1,4})*(/[a-zA-Z0-9\\&%_\\./-~-]*)?", Pattern.CASE_INSENSITIVE);
Matcher matcher = p.matcher(text);
return matcher.find();
}
5 其他的正则表达式
/**
* 验证工具类
*/
public class ValidateUtil {
private static final int PASS_LOW_LIMIT = 6;
private static final int PASS_HIGH_LIMIT = 16;
/**
* 验证手机号码是否合法
*/
public static boolean validatePhoneNumber(String mobiles) {
String telRegex = "^((13[0-9])|(15[^4])|(18[0-9])|(17[0-8])|(147,145))\\d{8}$";
return !TextUtils.isEmpty(mobiles) && mobiles.matches(telRegex);
}
/**
* 验证密码是否合法 6-16位
*/
public static boolean validatePass(String password) {
return password.length() >= PASS_LOW_LIMIT && password.length() <= PASS_HIGH_LIMIT;
}
/**
* 验证身份证号码是否合法
*/
public static boolean validateIDCardNumber(String number) {
return (number.length() == 15 && number.matches("^\\d{15}"))
|| (number.length() == 18 && (number.matches("^\\d{17}[x,X,\\d]")));
}
/**
* 判断是不是英文字母
*/
public static boolean isECharacter(String codePoint) {
return codePoint.matches("^[A-Za-z]$");
}
}
转载请注明:Android开发中文站 » Android基础之最新正则表达式
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK