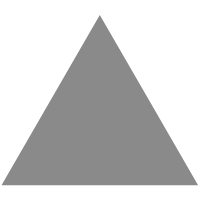
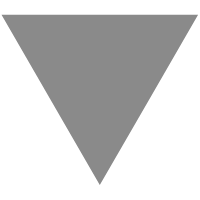
How to Use Optional Function Parameters in JavaScript
source link: https://code.tutsplus.com/tutorials/how-to-use-optional-function-parameters-in-javascript--cms-37376
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
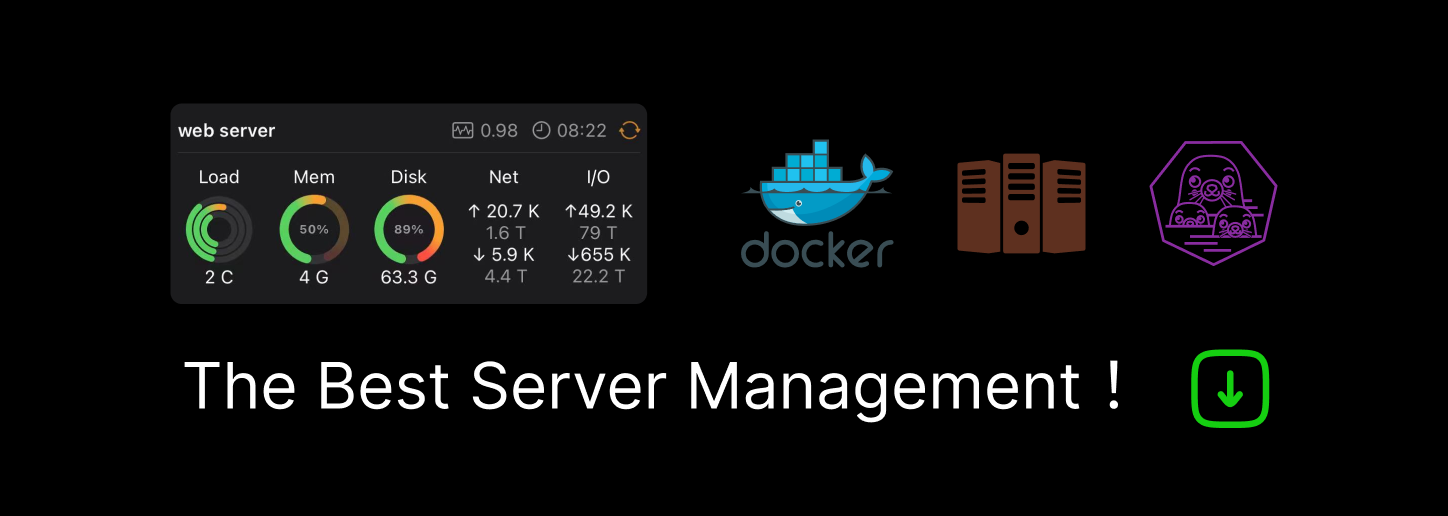
How to Use Optional Function Parameters in JavaScript
In this quick article, we’ll discuss how you can use optional function parameters in JavaScript.
JavaScript is one of the core technologies of the web. The majority of websites use it, and all modern web browsers support it without the need for plugins. In this series, we’re discussing different tips and tricks that would help you in your day-to-day JavaScript development.
In JavaScript coding, you often need to make function parameters optional. When you’re using JavaScript functions, there are two types of parameters: mandatory parameters and optional parameters. In case of mandatory parameters, you must pass those parameters, otherwise JavaScript throws an error. In case of optional parameters, though, if you don't pass them they will be initialized to some default value.
Today, we’ll discuss the basics of optional function parameters in JavaScript and how to use them.
How to Use Optional Function Parameters in ES5 and Before
In this section, we’ll discuss a solution which works even with older browsers. This was used frequently until the JavaScript ES5 era, when there was no built-in support available to make function parameters optional.
Let’s go through the following example to understand how it works.
function
getFullImagePath(imagePath, imageBaseUrl) {
imageBaseUrl = imageBaseUrl || 'https:
//code.tutsplus.com/’;
var
fullImagePath = imageBaseUrl + imagePath;
return
fullImagePath;
}
In the above example, the getFullImagePath
function takes two arguments, imagePath
and imageBaseUrl
. We want to make the second imageBaseUrl
parameter optional, so you can skip passing it if you want to use the default parameter value for it. To make it optional, we’ve used the following statement.
Basically, we’re checking if the imageBaseUrl
variable is defined, if it’s defined and evaluates to TRUE
, we assume that the second parameter is available, and we’ll use it. On the other hand, if the imageBaseUrl
parameter is undefined or evaluates to FALSE
, we’ll use the https://code.tutsplus.com/
value as the default value of this parameter. It’s important that the optional parameters should always come at the end on the parameter list.
Note that this approach won't work for numeric values because it will override the value 0
. Similarly if you want to be able to pass the value null
. If you want to be able to pass 0
or null
into your function, you'll have to explicitly check if the parameter is undefined.
function
getFullImagePath(imagePath, imageBaseUrl) {
var
fullImagePath = imageBaseUrl + imagePath;
return
fullImagePath;
}
In this case, we’ve explicitly checked if the value of the imageBaseUrl
parameter is undefined
to decide if it's an optional parameter. This is a more foolproof method to decide if the parameter is optional.
So that’s how you can make function parameters optional in browsers that don’t support the ES6+ editions. In the next section, we’ll discuss it in the context of modern browsers.
How to Use Optional Function Parameters in JavaScript ES6
In this section, we’ll discuss the method that you could use in modern browsers that support the ES6 edition of JavaScript. Let’s go through the following example to understand how it works. We’ll rewrite the example which we discussed in the previous section with its ES6 counterpart.
var
fullImagePath = imageBaseUrl + imagePath;
return
fullImagePath;
}
If you’ve worked with other programming languages, the above approach to define optional function parameters may look familiar to you. In this case, the optional parameter is assigned the default value in the function declaration statement itself.
Also, you can have multiple optional parameters as shown in the following snippet as long as you define them at the end on the parameter list.
function
foo(a, b=0, c=10) {
//...
}
So as you can see, the JavaScript ES6 syntax is much simpler and easier to write than the old method.
Conclusion
Today, we discussed how you can use optional function parameters in JavaScript along with a couple of real-world examples.
Here's a comparison of the different ways of coding optional function parameters in JavaScript:
Method Notes arg = arg || defaultValue
A common idiom before ES6, but 0
and null
will be overridden with the default value.
arg = (typeof arg === 'undefined') ? defaultValue : arg
The most fool-proof way of implementing optional parameters before ES6.
function something(arg=defaultValue) { }
The best method to use for ES6 and newer versions of JavaScript.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK