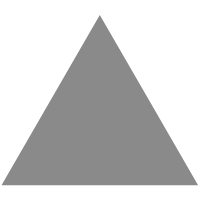
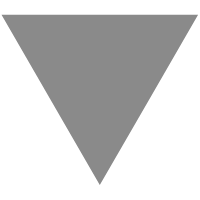
5 tips in PHP 🐘
source link: https://dev.to/saymon/5-tips-in-php-46n
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
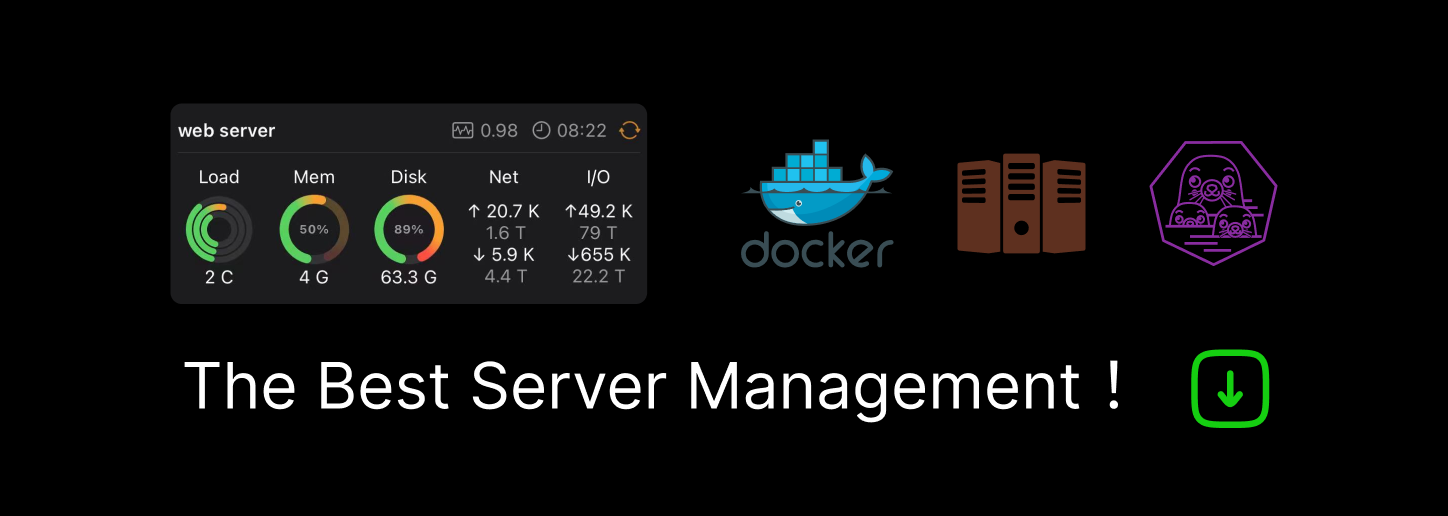
In this article, I’ll show you 10 tips that can improve your PHP code 🔍 🐘
In order to make this article easier to read, I’ll write some pieces of code that I’ve encountered and introduce a commented solution if needed, as you know there are 4242424242424 ways to do the same thing ✨ and It’s OK to disagree with my solution 😅
👀 For my examples I use PHP 7.4
🏁 🔥
1 - In this use case, just return the expression 👌
if($foo === 42) {
return true;
}
return false;
// can be
return $foo === 42;
2 - Null coalescing operator for the win 😍
if($foo !== null) {
return $foo;
}
return $bar;
// can be
return $foo ?? $bar;
💡 It’s very handy to avoid exceptions when you want to access a key that doesn’t exist
3 - Straight to the point 🥅
$this->user = ['firstname' => 'smaine', 'mail' => '[email protected]'];
return $this->user;
// can be
return $this->user = ['firstname' => 'smaine', 'mail' => '[email protected]'];
4 - Initialize your helper once 😛
class FooTransformer
{
private $propertyAccessor;
public function transform(array $data): Foo
{
$accessor = $this->getAccessor();
// stuff
}
private function getAccessor(): PropertyAccess
{
return $this->propertyAccessor ??= PropertyAccess::createPropertyAccessor(); // @lito
}
}
5 - Unpack an array 🎩
$users = [
['smaone', 'php'],
['baptounet', 'javascript'],
];
foreach($users as [$username, $language]) {
// $username contains the 1st key "smaone" for the 1st iteration
// $language contains the 2nd key "php" for the 1st iteration
}
In the same way, we can also assign variables from an array, it is useful if a function returns an array 🚀
function getFullname(int $id): array
{
$statement = $pdo->prepare("SELECT id, lastname, firstname FROM user WHERE id = ?");
$statement->execute([$id]);
$result = $statement->fetch();
// suppose $result contains [42, 'luke', 'lucky'];
return $result;
}
// main.php
[$id, $lastname, $firstname] = getFullname(42);
// $id contains 42
// $lastname contains luke
// $firstname contains lucky
I hope you enjoyed the read!
Feel free to follow me on GitHub, LinkedIn and DEV for more!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK