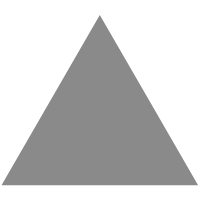
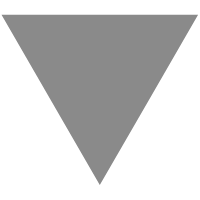
Sample Ruby Code Using DreamObjects S3-compatible API
source link: https://help.dreamhost.com/hc/en-us/articles/215253498-Sample-Ruby-Code-Using-DreamObjects-S3-compatible-API
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Sample Ruby Code Using DreamObjects S3-compatible API
Ruby AWS::SDK Examples (aws-sdk-v1 gem)
This article lists several Ruby code examples to interact with DreamObjects.
Creating a Connection
Create a connection so you can interact with DreamObjects.
AWS.config( :s3_endpoint => 'objects-us-east-1.dream.io', :access_key_id => 'my-access-key', :secret_access_key => 'my-secret-key' )
Instantiate a client object.
s3 = AWS::S3.new
Listing Owned Buckets
Get a list of Buckets you own and print the name.
s3.buckets.each do |bucket| puts bucket.name end
The output will look something like this:
mahbuckat1 mahbuckat2 mahbuckat3
Creating a Bucket
Create a new bucket called my-new-bucket.
s3.buckets.create('my-new-bucket')
Listing a Bucket’s Content
Get a list of hashes with the contents of each object This also prints out each object’s name, the file size, and last modified date.
new_bucket = s3.buckets['my-new-bucket'] new_bucket.objects.each do |obj| puts "#{obj.key}\t#{obj.content_length}\t#{obj.last_modified}" end
The output will look something like this if the bucket has some files:
myphoto1.jpg 251262 2011-08-08 21:35:48 -0400 myphoto2.jpg 262518 2011-08-08 21:38:01 -0400
Deleting a Bucket
The Bucket must be empty, otherwise, it won’t work.
bucket = s3.buckets['my-new-bucket'] bucket.delete
Forced Delete for Non-empty Buckets
bucket = s3.buckets['my-new-bucket'] bucket.delete!
Creating an Object
Create a file hello.txt
with the string "Hello World!"
bucket = s3.buckets['my-new-bucket'] obj = bucket.objects.create( 'hello.txt', 'Hello World!', )
Upload a file test.txt
, set the content-type, and make it publicly readable.
obj = s3.buckets['my-new-bucket'].objects['test.txt'] obj.write(File.open('path/to/test.txt', 'rb') :content_type => 'text/plain', :acl => 'public_read', )
Change an Object’s ACL
Make the object hello.txt
publicly readable and secret_plans.txt
to be private.
bucket = s3.buckets['my-new-bucket'] bucket.objects['hello.txt'].acl = :public_read bucket.objects['secret_plans.txt'].acl = :private
Download an Object (to a file)
Download the object poetry.pdf
and save it in /home/username/documents/.
Make sure to change username to your Shell user.
bucket = s3.buckets['my-new-bucket'] File.open('/home/username/documents/poetry.pdf', 'w') do |file| bucket.objects['poetry.pdf'].read do |chunk| file.write(chunk) end end
Delete an Object
Delete the object goodbye.txt.
bucket = s3.buckets['my-new-bucket'] bucket.objects.delete('goodbye.txt')
Generate Object Download URLs (signed and unsigned)
This generates an unsigned download URL for hello.txt
. This works because hello.txt
was made public by setting the ACL above. This then generates a signed download URL for secret_plans.txt
that will work for 1 hour. Signed download URLs will work for the time period even if the object is private (when the time period is up, the URL will stop working).
puts s3.buckets['my-new-bucket'].objects['hello.txt'].public_url puts s3.buckets['my-new-bucket'].objects['secret_plans.txt'].url_for( :read, :expires => 60 * 60 )
The output of this will look something like:
https://objects-us-east-1.dream.io/my-bucket-name/hello.txt https://objects-us-east-1.dream.io/my-bucket-name/secret_plans.txt?Signature=XXXXXXXXXXXXXXXXXXXXXXXXXXX&Expires=1316027075&AWSAccessKeyId=XXXXXXXXXXXXXXXXXXX
Ruby AWS::S3 Examples (aws-s3 gem)
Creating a Connection
Create a connection so you can interact with the server.
AWS::S3::Base.establish_connection!( :server => 'objects-us-east-1.dream.io', :use_ssl => true, :access_key_id => 'my-access-key', :secret_access_key => 'my-secret-key' )
Listing Owned Buckets
Get a list of AWS::S3::Bucket objects you own. This also prints out the bucket name and creation date of each bucket.
AWS::S3::Service.buckets.each do |bucket| puts "#{bucket.name}\t#{bucket.creation_date}" end
The output will look something like this:
mahbuckat1 2011-04-21T18:05:39.000Z mahbuckat2 2011-04-21T18:05:48.000Z mahbuckat3 2011-04-21T18:07:18.000Z
Creating a Bucket
Create a new bucket called my-new-bucket.
AWS::S3::Bucket.create('my-new-bucket')
Listing a Bucket’s Content
Get a list of hashes with the contents of each object This also prints out each object’s name, the file size, and last modified date.
new_bucket = AWS::S3::Bucket.find('my-new-bucket') new_bucket.each do |object| puts "#{object.key}\t#{object.about['content-length']}\t#{object.about['last-modified']}" end
The output will look something like this if the bucket has some files:
myphoto1.jpg 251262 2011-08-08T21:35:48.000Z myphoto2.jpg 262518 2011-08-08T21:38:01.000Z
Deleting a Bucket
The Bucket must be empty, otherwise it won’t work.
AWS::S3::Bucket.delete('my-new-bucket')
Forced Delete for Non-empty Buckets
AWS::S3::Bucket.delete('my-new-bucket', :force => true)
Creating an Object
Create a file hello.txt
with the string "Hello World!"
AWS::S3::S3Object.store( 'hello.txt', 'Hello World!', 'my-new-bucket', :content_type => 'text/plain' )
Change an Object’s ACL
Make the object hello.txt
publicly readable and secret_plans.txt
to be private.
policy = AWS::S3::S3Object.acl('hello.txt', 'my-new-bucket') policy.grants = [ AWS::S3::ACL::Grant.grant(:public_read) ] AWS::S3::S3Object.acl('hello.txt', 'my-new-bucket', policy) policy = AWS::S3::S3Object.acl('secret_plans.txt', 'my-new-bucket') policy.grants = [] AWS::S3::S3Object.acl('secret_plans.txt', 'my-new-bucket', policy)
Download an Object (to a file)
Download the object poetry.pdf
and saves it in /home/username/documents/.
Make sure to change username to your Shell user.
open('/home/username/documents/poetry.pdf', 'w') do |file| AWS::S3::S3Object.stream('poetry.pdf', 'my-new-bucket') do |chunk| file.write(chunk) end end
Delete an Object
Delete the object goodbye.txt.
AWS::S3::S3Object.delete('goodbye.txt', 'my-new-bucket')
Generate Object Download URLs (signed and unsigned)
This generates an unsigned download URL for hello.txt
. This works because hello.txt
was made public by setting the ACL above. This then generates a signed download URL for secret_plans.txt
that will work for 1 hour. Signed download URLs will work for the time period even if the object is private (when the time period is up, the URL will stop working).
puts AWS::S3::S3Object.url_for( 'hello.txt', 'my-new-bucket', :authenticated => false ) puts AWS::S3::S3Object.url_for( 'secret_plans.txt', 'my-new-bucket', :expires_in => 60 * 60 )
The output of this will look something like:
https://objects-us-east-1.dream.io/my-bucket-name/hello.txt https://objects-us-east-1.dream.io/my-bucket-name/secret_plans.txt?Signature=XXXXXXXXXXXXXXXXXXXXXXXXXXX&Expires=1316027075&AWSAccessKeyId=XXXXXXXXXXXXXXXXXXX
Recommend
-
2
Arq is a Mac and Windows app that allows you to make online backups. Arq back...
-
7
Using a script to backup your website and database to DreamObjects Overview Using a simple shell script, you can automate website and database backups to DreamObjects. This script...
-
1
How to manage accounts in DreamObjects Overview The Account tab on the DreamObjects page helps you revie...
-
8
Overview ownCloud is a free and open source file hosting application that provides universal file access, synchronization, and sharing of your data (using a single interface), regardless of w...
-
3
How to monitor usage and costs of DreamObjects Overview The Usage Report tab in the panel provides billing and storage details relating to your current DreamObjects service plan.
-
3
How to upload files into DreamObjects Overview There are a wide variety of ways to store files in DreamObjects, mainly depending on your preferences and skills. Uplo...
-
3
Sample Java Code Using DreamObjects S3-compatible API This article lists several Java code examples to interact with DreamObjects. Setup The following e...
-
3
This article lists several C# code examples to interact with DreamObjects. Creating a Connection Create a connection so you can interact with the server. using System; using Amazon; using Amazon.S3; usin...
-
6
How To Use DreamObjects S3-compatible API DreamObjects RADOS S3 API DreamObjects supports the Amazon S3 API, so it’s easy to use your existing apps. Howe...
-
13
This article lists several Perl code examples to interact with DreamObjects. Creating a Connection Create a connection so you can interact with the server. use Amazon::S3; my $access_key = 'put your access key...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK