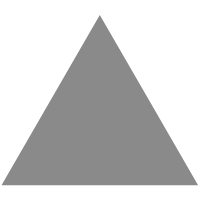
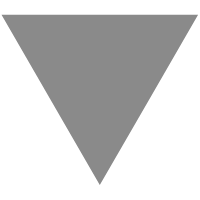
Learning Python- Intermediate course: Day 6, Math Exercises
source link: https://dev.to/aatmaj/learning-python-intermediate-course-day-6-math-exercises-12ge
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
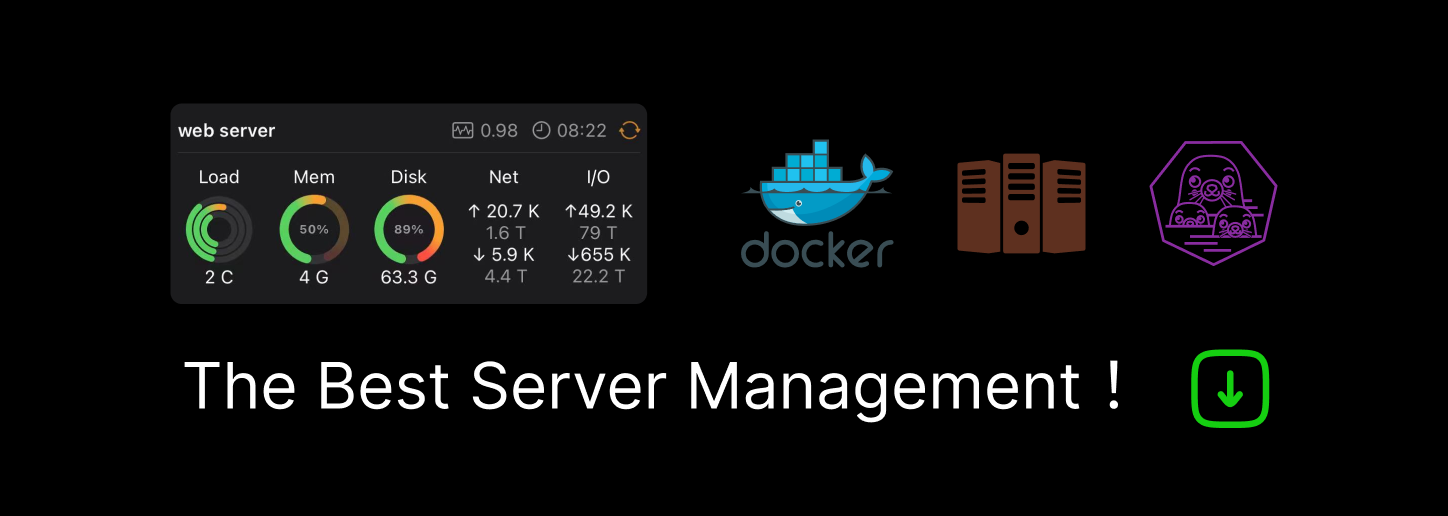
In the last part we covered the math module. In this part, we will solve some questions related to it.
Sample questions.
- Write a program to check if a number is an "Armstrong number" or not
def armstrong(x):
a=str(x) #convert int to string to make it iteratable
counter=0
Sum=0
for i in a:
counter=counter+1
for i in a:
Sum=Sum+pow(int(i),counter)
if(Sum==x):
return True
return False
print(armstrong(153))
print(armstrong(1723))
True
False
Alternative
def armstrong(x):
n = 0
i=x
while (i != 0):
n = n + 1
i = i // 10
temp = x
Sum = 0
while (temp != 0):
r = temp % 10
Sum = Sum + pow(r, n)
temp = temp // 10
return (Sum == x) #shorthand for if Sum==x return True else return False
print(armstrong(153))
print(armstrong(1723))
True
False
- Write the function power which returns the number raise to a power without using the math function
pow()
def power(x, y):
if (y == 0):
return 1
if (y % 2 == 0):
return power(x, y // 2) * power(x, y // 2)
return x * power(x, y // 2) * power(x, y // 2)
print(power(2,3))
print(pow(2,3))
8
8
Logic The function power is a function which makes use of recursion in Python. The power is raised using recursive multiplication.
Alternative-
def power(a, b):
temp=a
for i in range(1,b):
temp=temp*a
return temp
print(power(3,4))
print(pow(3,4))
81
81
Exercise.
Write the following functions without using their predefined math versions.
hypot()
sqrt()
asinh()
Answers in the Learning Python Repository
So friends that's all for this part. 😊 Hope you all are enjoying.😎 Please let me know in the comment section if you liked it or not. 🧐 And don't forget to like the post if you did. 😍 I am open to any suggestions or doubts. 🤠 Just post in the comments below or Gmail me. 😉
Thank you for being so patient.👍
Also please visit the Learning-Python repo made especially for this course and don't forget to star it too!!!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK