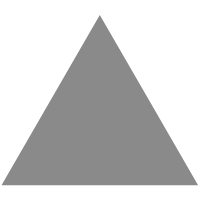
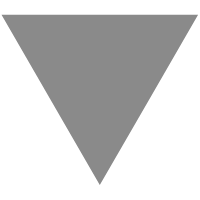
Lambda Expressions: An Introduction in Java 8
source link: https://blog.knoldus.com/lambda-expressions-an-introduction-in-java-8/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
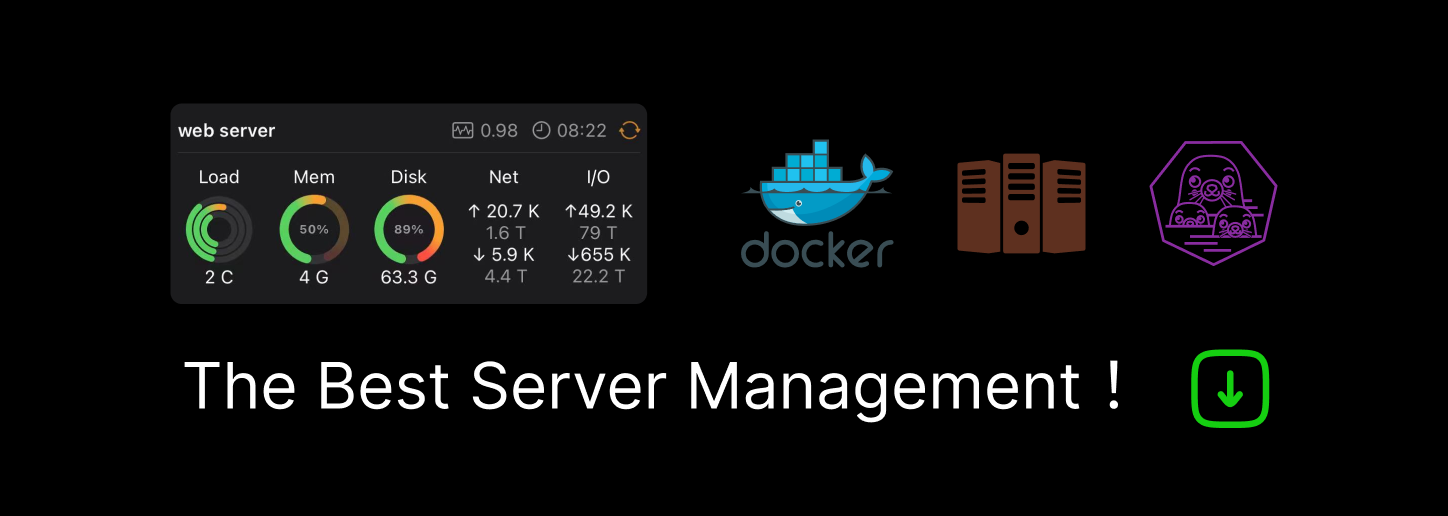
Lambda Expressions: An Introduction in Java 8
Reading Time: 2 minutes
Lambda expressions were one of the new features that was introduced in Java 8.
They help clean up verbose code by providing a concise and local way to reduce redundancy by keeping code short and self-explanatory.
In addition to saving code, Java’s lambda expressions are important in functional programming. They allow developers to write in a functional style by acting as functions without belonging to any class.
Lambda expressions allow us to implement functional interfaces by converting them into instances of functional interfaces. This provides a reference to the function. For example, a lambda passed as a parameter is indistinguishable to calling an implemented interface method). Thus, the references are used effectively as a function type object that can be passed and called on.
Syntax
([argument-list]) -> {body}
Lambda expressions have an optional argument list and a body
Functional Interfaces
- A functional interface is an interface with only one abstract method
- Lambdas are matched to functional interfaces, allowing the compiler to interpret the type of the variables in the argument list
- The parameters of the lambda expression must match the parameters of the single method and the return type of the lambda must match the return type of the single method
@FunctionalInterface
public interface Comparator<T> {
int compare(T o1, T o2);
}
Anonymous classes were used as a workaround for passing references to functions, that is, compare in this case
Comparator<Person> descName = new Comparator<Person>() {
@Override
int compare(Person o1, Person o2) {
return o1.getName().compareTo(o2.getName());
}
}
Lambda expressions have allowed us to replace the verbose anonymous classes with short, concise, and functional expressions that serve the same purpose.
Comparator<Person> descName =
(o1, o2) -> o1.getName().compareTo(o2.getName());
Note: (o1, o2) and o1.getName().compareTo(o2.getName()) matches up with the parameter list and return type of the compare method in the Comparator<Person> interface.
Method References as Lambdas
If a lambda expression just calls another method then a more concise way to write the call is to use a method reference.
public interface MyPrinter{
public void print(String s);
}
MyPrinter myPrinter = System.out::println;
The :: signals a method reference, where the object/class comes before it and the method that belongs to that class comes after.
Hopefully these tips provide you with a useful introduction to coding functional Java programs. Happy coding and happy blogging!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK