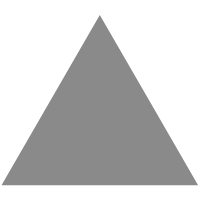
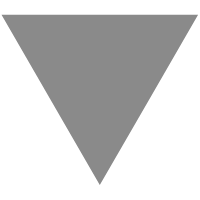
实现SpringBoot + Redis缓存的源码与教程
source link: https://www.jdon.com/57033
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
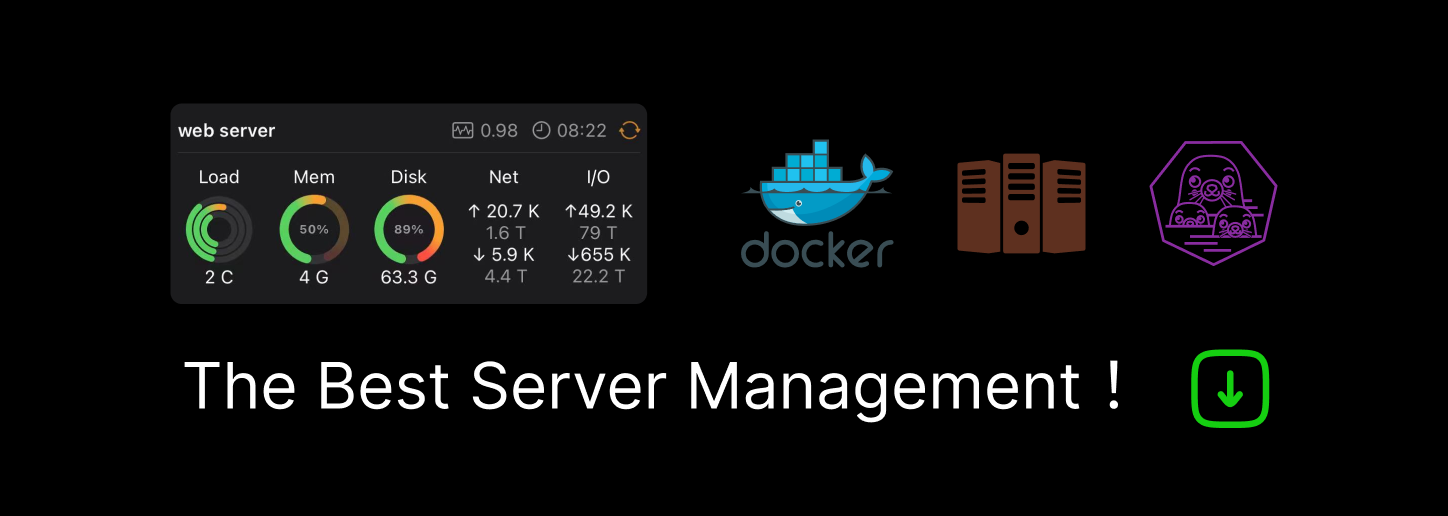
总结了Redis的基础知识以及如何使用Redis在Spring Boot中集成缓存。
首先是依赖:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency>
使用MySQL作为我的主要数据库,所以我使用 MySQL 连接器,还有 Spring Data Redis (access+driver) 依赖项在我们的项目中用作 Java Redis 客户端,这允许我们的应用程序启用 Redis 缓存机制。
现缓存时常用的注解如下:@CachePut、@CacheEvict、@Cacheable、@Caching,我们将看到我们在哪里以及如何使用这些注解。
@Entity public class User implements Serializable{ @Id @GeneratedValue(strategy = GenerationType.AUTO) private Integer id; private String name; private String email; private Integer age; public User() { super(); } public User(Integer id, String name, String email, Integer age) { super(); this.id = id; this.name = name; this.email = email; this.age = age; }
启用
@SpringBootApplication @EnableCaching public class RedisCacheApplication { public static void main(String[] args) { SpringApplication.run(RedisCacheApplication.class, args); } }
只要通过@EnableCaching注释启用缓存支持,Spring Boot 就会自动配置缓存基础结构。
查询
在控制器中,我们必须在每个 API 之上添加不同的注释来缓存特定记录。
@GetMapping("/{id}") @Cacheable(value= "Users", key="id") public User getUser(@PathVariable Integer id) { log.info(">> User Controller: get user by id: " + id); return userService.getUserById(id); }
在 GET 方法之上,我们使用@Cacheablewhich 表示每次调用该方法时,都会应用缓存行为,检查是否已经为给定的参数调用了该方法。
value="{category}"指定数据需要存储在哪个类别下。对于不同的控制器,我们可以使用不同的值。
一个合理的默认值只是使用方法参数来计算键,但我们也可以指定键以及使用key="#{value}"哪个将用于识别特定记录。
如果在缓存中找不到计算键或指定键的值,则将调用目标方法并将返回值存储在关联的缓存中。返回类型会自动处理,如果存在,它们的内容存储在缓存中。
保存
@PutMapping @CachePut(value="users", key="user", unless="result == null") public User updateUser(@RequestBody User user) { log.info(">> User Controller: update user: " + user.toString()); return userService.update(user); }
在PUT 方法之上,我们使用@CachePut. 我们也可以使用@CachePut本身来保存新用户,即POST 方法。
如果condition() 和unless() 表达式相应地匹配,则它始终会导致调用该方法并将其结果存储在关联的缓存中。Condition() 是 Spring 表达式语言 (SpEL) 表达式,用于有条件地进行缓存放置操作。由于 put 操作的性质,该表达式在调用方法后计算,因此可以引用方法的调用结果。
删除
@DeleteMapping("/{id}") @CacheEvict(value= "Users", allEntries = false, key="id") public void removeUser(@PathVariable Integer id) { log.info(">> User Controller: delete user: " + id); userService.delete(id); }
在 DELETE 方法之上,我们使用@CacheEvict它允许我们删除 Redis 中特定键的数据。allEntries指定是否应删除缓存中的所有条目。默认情况下,仅删除关联键下的值。请注意,不允许将此参数设置为 true 并指定键。
应用程序属性
我们可以通过不同的属性在我们的应用程序中配置 Redis 缓存,我使用了以下属性,如下所示:
spring.redis.host=localhost spring.redis.port=6379 spring.cache.redis.time-to-live=60000 spring.cache.cache-names=users,product,order
默认情况下,会根据需要创建缓存,但您可以通过设置该cache-names属性来限制可用缓存的列表。
我们可以用来在Spring中配置 Redis 缓存的属性如下所示:
要记住的要点
- 定义 TTLs :生存时间 (TTL),是您的缓存将删除条目的时间跨度。如果您只想每分钟获取一次数据,只需使用 @Cacheable Annotation 保护它并将 TTL 设置为 1 分钟。
- 实现 Serializable:如果你在 Redis 缓存中添加一个对象,那么该对象应该实现一个 Serializable 接口。
- Redis 缓存限制:当缓存大小达到内存限制时,旧数据将被删除,为新数据腾出空间。尽管 Redis 速度非常快,但在 64 位系统上存储任何数量的数据仍然没有限制。它只能在 32 位系统上存储 3GB 的数据。
- 永远不要从同一个类中调用可缓存方法:原因是 Spring 代理了对这些方法的访问,以使缓存抽象工作。当你在同一个类中调用它时,这个代理机制不会启动。通过这个,你基本上绕过了你的缓存并使其无效。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK