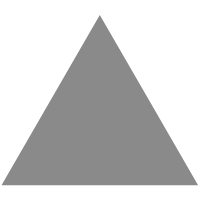
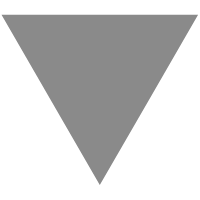
Akka HTTP Route Testing
source link: https://blog.knoldus.com/akka-http-route-testing/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
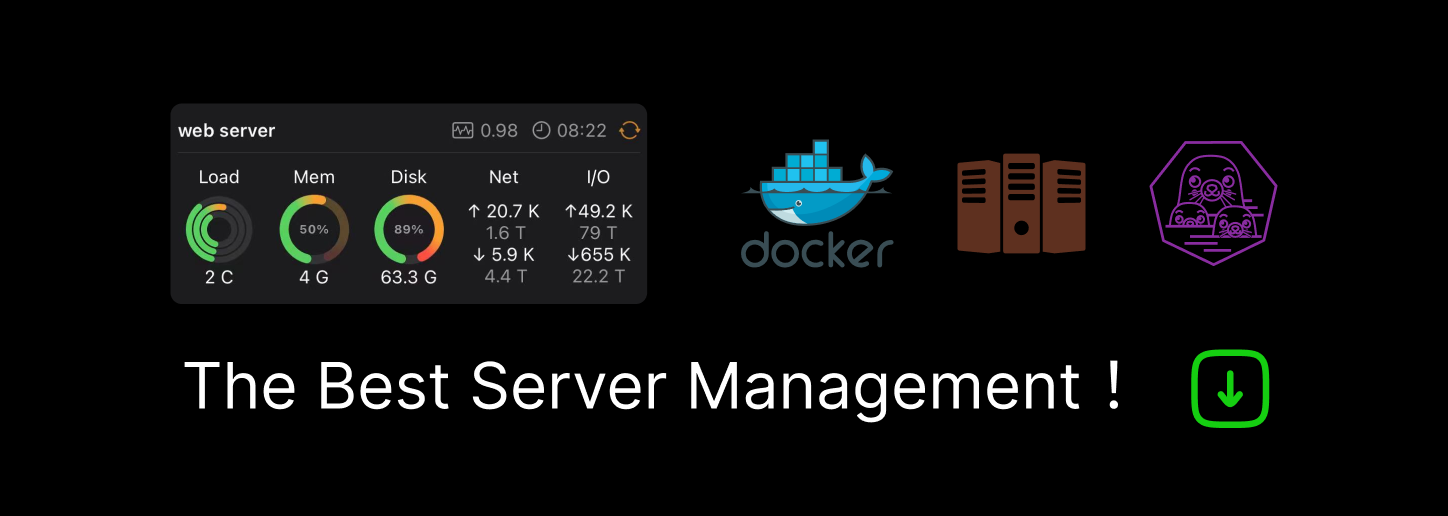
Akka HTTP puts a lot of focus on testability of code. It has a dedicated module akka-http-testkit for testing rest api’s. When you use this testkit you are not need to run external web server or application server to test your rest API’s. It will do all needed the stubbing and mocking for you which greatly simplifies the testing process.
Adding dependency
You need to add akka-http-testkit library to test your rest services.
<dependency>
<groupId>com.typesafe.akka</groupId>
<artifactId>akka-stream-testkit_2.12</artifactId>
<version>2.5.26</version>
</dependency>
<dependency>
<groupId>com.typesafe.akka</groupId>
<artifactId>akka-http-testkit_2.12</artifactId>
<version>10.1.12</version>
</dependency>
Note : Since version 10.1.6, akka-stream-testkit is a provided dependency, please remember to add it to your build dependencies.
Testing Rest API
1. Create Spec with ScalatestRouteTest
class RestSpec extends WordSpec with Matchers with ScalatestRouteTest{
}
The above code uses scala-test for testing. In our spec, we mix ScalatestRouteTest which comes from akka-http-testkit library. It provides the actor system and flow materializer for test environment. ‘
2. Create the routes to test
val smallRoute: Route =
get {
concat(
pathSingleSlash {
complete {
"List all comments!"
}
},
path("comment") {
complete("Show Comment")
}
)
} ~
post {
path("/comment")
entity(as[String])
{ comment => complete(s"added the comment '$comment' to the tutorial")
}}
3. Test Route
Once we have the request, we can send the request using ~> operator as below code.
"The service" should {
"return all comments for GET requests to the root path" in {
// tests:
Get() ~> smallRoute ~> check {
responseAs[String] shouldEqual "List all comments"
}
}
"return a 'Show Comment' response for GET requests to /comment" in {
// tests:
Get("/comment") ~> smallRoute ~> check {
responseAs[String] shouldEqual "Show Comment"
}
}
"add comments to a tutorial" in {
Post("/comment", "new comment") ~> smallRoute ~> check {
responseAs[String] shouldBe "added the comment 'new comment' to the
tutorial" }}
The basic structure of a test built with the testkit is this (expression placeholder in all-caps):
REQUEST ~> ROUTE ~> check {
ASSERTIONS
}
Conclusion
Akka HTTP’s routing DSL might seem confusing at first, but after overcoming some bumps it just clicks. After a while it’ll come naturally and it can be very powerful.
We learned how to structure our routes, but more importantly, we learned how to create that structure guided by tests which will make sure we don’t break them at some point in the future.
Even though we only worked on three endpoints, we ended up with a somewhat complicated and deep structure.
Reference
https://doc.akka.io/docs/akka-http/current/routing-dsl/testkit.html
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK