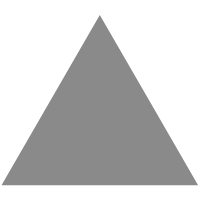
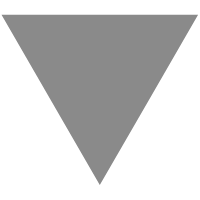
Python Basics, Python's 101!
source link: https://dev.to/namaiyian/python-basics-python-s-101-1m3i
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
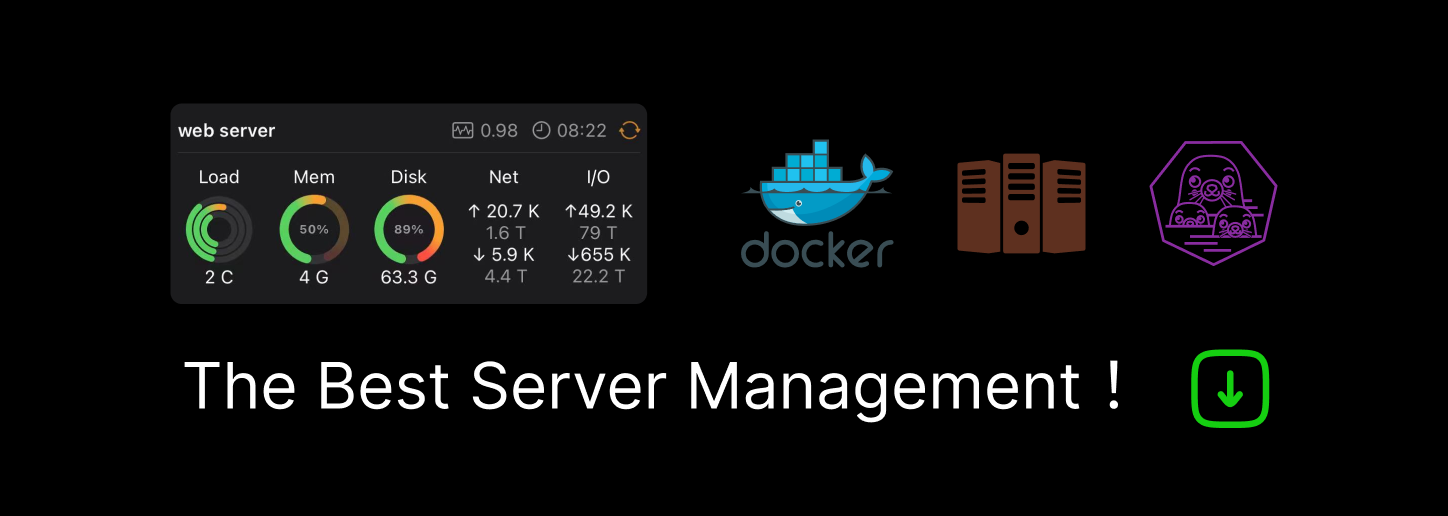
Python Basics, Python's 101!
Jul 24
・5 min read
In the recent years, Python has become one of the most popular programming languages worldwide. It is a high-level and general-purpose programming language.
This article will focus on the basic syntax, data types, control flow among other concepts that are important to all beginner Python programmers.
REASONS WHY PYTHON IS A POPULAR PROGRAMMING LANGUAGE
- Python is easy to learn and use, due to its simplified syntax.
- Python has a large community, where new developers can grow and mature optimally in their careers.
- Python is cross-platform, hence it can be used in any kind of environment and still produce maximum results.
APPLICATIONS OF PYTHON PROGRAMMING
Python has a large number of modules, packages and frameworks that allow it to be applied in the following areas, among many others:
- Web development
- Machine Learning and Artificial Intelligence
- Data Science
- Automation
- Game development
FUNDAMENTALS OF PYTHON PROGRAMMING
Whitespace and Indentation
The general structure of Python code mainly consists of whitespace and indentation, unlike other languages that mostly use semicolons and curly braces. Let's take a look at an example:
if __name__ == "__main__":
print("Hello Python programmers!")
From the code snippet above, we can conclude that indentation and whitespace make it easier for the programmer to know where a block of code begins and where it ends, thereby enhancing readability of code.
Comments
Python programming also involves the use of comments, which are statements that are written in a program to explain the purpose of a specific line or block of code. They are ignored by the Python interpreter. Here's an example of a comment:
#displaying a child's age
Identifiers
Another important part of python syntax is identifiers. These are words that are used to name objects such as variables, functions and classes. Python identifiers begin with either a letter or underscore(_).The subsequent characters can be either underscores or alphanumeric characters. For example, class_teacher can be an identifier, but 2student cannot be used as an identifier.
Keywords
Python also has several keywords, which are basically words with special meaning, and that may be used in performing specific tasks. They should not be used as identifiers. They include the following:
if
else
True
VARIABLES
A variable is a storage location for a value. It is declared as follows:
name = "John"
print(name)
The variable "name" stores the value "John". When naming variables, we should note that they cannot start with numbers or contain spaces. For example, num_1 can be a variable name, but num 1 cannot be a variable name. The names of variables should be as concise and descriptive as possible.
DATA TYPES IN PYTHON
I) STRINGS
A string is a series of characters. Strings in Python are usually enclosed with quotation marks. For example:
city = 'New York'
country = "Kenya"
The declarations above are of single-line strings. Python also allows multi-line strings, where we use triple quotation marks:
customer1 = '''Name: John Doe
ID: 224
Address: Summer Beach House, 110'''
print(customer1)
II) NUMBERS
In Python programming, various types of numbers are supported, for example integers, floats and complex numbers. Integers are numbers like 1, 3 and 45. Floats are numbers with a decimal point. They are also known as floating-point numbers. An example of number declaration is shown below:
num1 = 20
num2 = 5.5
num3 = complex(5, 6)
From the above examples, num1 is assigned an integer, num2 is assigned a floating-point number and num3 is assigned a complex number.
III) BOOLEAN DATA TYPE
In Python, the Boolean data type usually represents True or False. It is often used to check if a condition is true or not and perform a specific action based on the result.
For example:
is_allowed = True
is_full = False
Constants
Sometimes when writing our code, we may need to store values in variables and desire not to change the values. Python does not directly allow us to declare constants, but we can declare the variables with capital letters to differentiate them from other values that may not be constants. Here's an example:
TIME_LIMIT = 2
OPERATORS IN PYTHON
I) Assignment Operator
It is used to assign a value to a variable. An example is shown below:
basic_salary = 100000
Other examples include +=, -=, /= etc.
II) Arithmetic Operators
In Python, we have operators that are user to perform arithmetic operations such as addition, subtraction, multiplication and division. Some of these operations are shown in the code below:
a = 55
b = 5
c = a + b # Addition
d = a - b # Subtraction
e = a * b # Multiplication
f = a / b # Division
print("55 + 5 = " + c)
print("55 - 5 = " + d)
print("55 * 5 = " + e)
print("55 / 5 = " + f)
III) Relational Operators
These are also called comparison operators and are used to compare values. For example:
25 < 30
5 == "a"
The first statement will return True, while the second statement will return False.
IV) Logical Operators
They are used to check multiple conditions at the same time. They include the 'and', 'or' and 'not' operators. For example:
age = 24
age < 40 and age > 5
The expression returns True since both conditions are true.
V) Membership Operators
These include the 'in' and 'not in' operators. For example:
4 in [1, 2, 3, 4, 5, 6]
The statement returns True because 4 is present in the list.
VI) Identity Operators
They check whether any two values are identical. For example:
4 is 4.000
The expression evaluates to False.
VII) Bitwise Operators
These are the operators that operate on any given values bit by bit. They include bitwise and, bitwise or etc.
CONTROL FLOW IN PYTHON
I) If Statement
The if statement is used to execute a block of code based on a specific condition. If the condition is true, the block of code gets executed. Otherwise, it is ignored.
II) If...else Statement
This is used when we want to perform a specific action when the condition is true and a different action when the condition turns out to be false.
III) If...elif...else Statement
This is used to check more than two conditions and perform different actions according to whether they are true or false. Here is a short example:
subject = input("Enter the subject you wish to register: )
if subject == "Mathematics":
print("Available")
elif subject == "Physics":
print("Check next week")
else:
print("Unavailable")
IV) For loop
A for loop is used when we need to execute a block of code for a specific number of times.
The syntax could be as follows:
for index in range(r):
statement
V) While loop
This structure allows us to execute a block of code for as long as the condition remains true. An example in syntax would be:
while x < 10:
statement
x += 1
In conclusion, these are the most basic concepts that we need to understand and master in our journey to become exceptional developers. Happy coding!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK