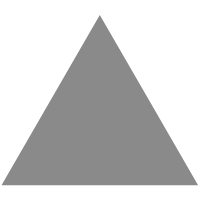
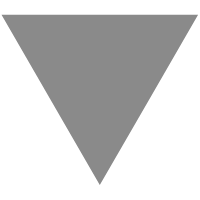
Maven git commit id plugin
source link: https://mydeveloperplanet.com/2018/02/22/maven-git-commit-id-plugin/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
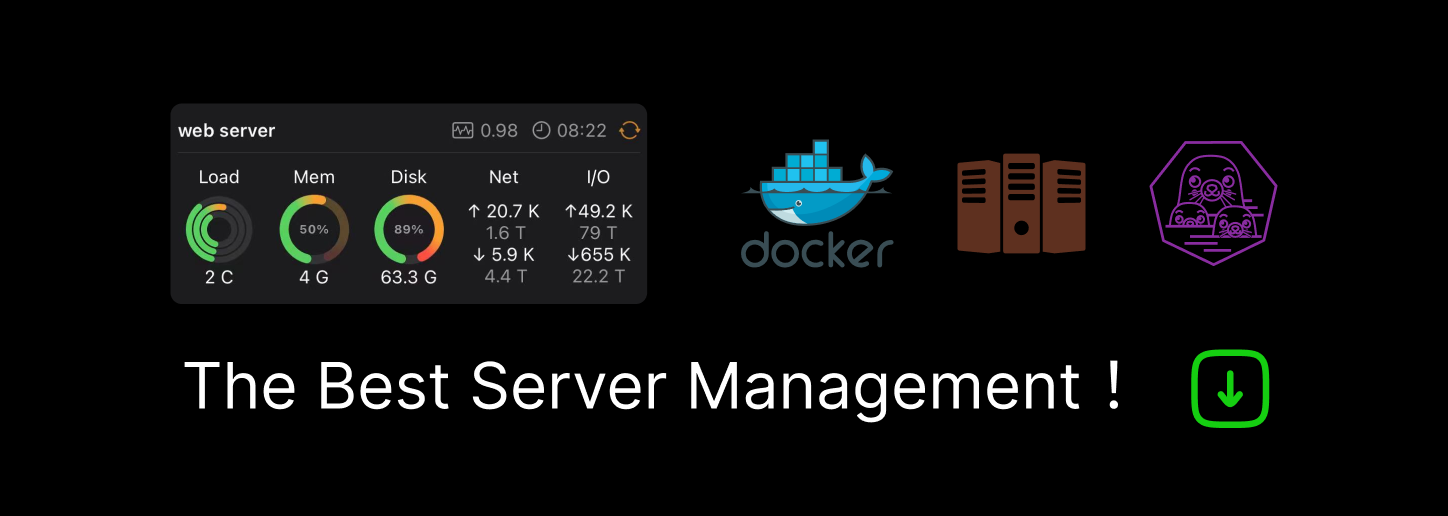
Maven git commit id plugin
Did you ever had the problem that you did not know which version of your application was deployed on e.g. a test environment? Or you had to manually adapt version information for each release in order to make it available in an About-dialog? Then the Maven git commit id plugin comes to the rescue! In this post, we will build a Spring Boot application with a RESTful webservice for retrieving versioning information. The only thing we will have to do, is to configure the Maven git commit id plugin and create the webservice. After this, versioning information is automatically updated during each build!
Create Spring Boot application
First, we create a basic Spring Boot application with a RESTful webservice for retrieving manually entered version information. JDK9 and modules are used, we will encounter some ‘problems’ along the way, these will be tackled also
We create the VersionController class which has hard-coded version information in it. We will replace it later on with the information retrieved from the git commit id plugin.
package com.mydeveloperplanet.mygitcommitidplanet; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import static org.springframework.web.bind.annotation.RequestMethod.GET; @RestController public class VersionController { @RequestMapping("/version", method= GET) public String versionInformation() { return "Version 1.0-SNAPSHOT"; } }
Add the entry point for the Spring Boot application in an Application.java class:
package com.mydeveloperplanet.mygitcommitidplanet; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
Build the application with Maven targets clean install. The module-info.java must be the following:
module com.mydeveloperplanet.mygitcommitidplanet { requires spring.web; requires spring.boot; requires spring.boot.autoconfigure; }
Building the application gave the following error:
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-jar-plugin:2.6:jar (default-jar) on project mygitcommitidplanet: Execution default-jar of goal org.apache.maven.plugins:maven-jar-plugin:2.6:jar failed: An API incompatibility was encountered while executing org.apache.maven.plugins:maven-jar-plugin:2.6:jar: java.lang.ExceptionInInitializerError: null
This is being solved by using version 3.0.2 of the maven jar plugin. Add the following to the plugin section of the pom:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <version>3.0.2</version> </plugin>
Run the application with the following command from the root of the project:
java -jar target/mygitcommitidplanet-1.0-SNAPSHOT.jar
Starting the application, the following warning is shown:
WARNING: An illegal reflective access operation has occurred WARNING: Illegal reflective access by org.springframework.cglib.core.ReflectUtils$1...
It appears to be a known problem, see SPR-15859
Go to your browser and enter the url http://localhost:8080/version. As expected, the hard-coded value is returned:
Version 1.0-SNAPSHOT
Git commit id plugin
Now that we have everything in place, we can start adding the git commit id plugin. Detailed information of all properties can be found on the GitHub repository of the git commit id plugin.
Enable git commit id
Add the following to the plugin section of the pom file:
<plugin> <groupId>pl.project13.maven</groupId> <artifactId>git-commit-id-plugin</artifactId> <version>2.2.4</version> <executions> <execution> <id>get-the-git-infos</id> <goals> <goal>revision</goal> </goals> </execution> </executions> <configuration> <dotGitDirectory>${project.basedir}/.git</dotGitDirectory> <prefix>git</prefix> <verbose>false</verbose> <generateGitPropertiesFile>true</generateGitPropertiesFile> <generateGitPropertiesFilename>${project.build.outputDirectory}/git.properties</generateGitPropertiesFilename> <format>json</format> <gitDescribe> <skip>false</skip> <always>false</always> <dirty>-dirty</dirty> </gitDescribe> </configuration> </plugin>
Run the Maven build. In the directory target/classes the git.properties file is added with the version information in json format.
{ "git.branch" : "master", "git.build.host" : "LT0265", "git.build.time" : "2018-01-21T17:34:26+0100", "git.build.user.email" : "[email protected]", "git.build.user.name" : "Gunter Rotsaert", "git.build.version" : "1.0-SNAPSHOT", "git.closest.tag.commit.count" : "", "git.closest.tag.name" : "", "git.commit.id" : "6f592254e2e08d99a8145f1295d4ba3042310848", "git.commit.id.abbrev" : "6f59225", "git.commit.id.describe" : "6f59225-dirty", "git.commit.id.describe-short" : "6f59225-dirty", "git.commit.message.full" : "Created basic Spring Boot application with webservice for retrieving hard-coded version information", "git.commit.message.short" : "Created basic Spring Boot application with webservice for retrieving hard-coded version information", "git.commit.time" : "2018-01-21T17:33:13+0100", "git.commit.user.email" : "[email protected]", "git.commit.user.name" : "Gunter Rotsaert", "git.dirty" : "true", "git.remote.origin.url" : "https://github.com/mydeveloperplanet/mygitcommitidplanet.git", "git.tags" : "" }
Now take a closer look at the mygitcommitidplanet-1.0-SNAPSHOT.jar file. In directory BOOT-INF/classes, the file git.properties is available. At this point, we already have what we want: versioning information is included in our deliverable. We can always look inside the jar file to find out what the exact version information of the sources was for this deliverable.
Add version information to RESTful webservice
The next step is to replace the hard-coded version information from our RESTful webservice with the contents of the git.properties file. Since the git.properties file is already in json format, the only thing we have to do, is to read the contents of the file and return it in our webservice.
Our VersionController.java file becomes the following:
@RequestMapping(value = "/version", method= GET) public String versionInformation() { return readGitProperties(); } private String readGitProperties() { ClassLoader classLoader = getClass().getClassLoader(); InputStream inputStream = classLoader.getResourceAsStream("git.properties"); try { return readFromInputStream(inputStream); } catch (IOException e) { e.printStackTrace(); return "Version information could not be retrieved"; } } private String readFromInputStream(InputStream inputStream) throws IOException { StringBuilder resultStringBuilder = new StringBuilder(); try (BufferedReader br = new BufferedReader(new InputStreamReader(inputStream))) { String line; while ((line = br.readLine()) != null) { resultStringBuilder.append(line).append("\n"); } } return resultStringBuilder.toString(); }
Build with Maven and run the application. Again, go to the url http://localhost:8080/version. The contents of our git.properties file is shown.
This is exactly what we wanted: the version information can be retrieved at any moment and is always up-to-date. If you have a client application, e.g. a browser application, it is easy to use this information in an About-section.
Validating git properties
It is also possible to add validation on the git.properties when you want to restrict the format. The build will fail if not met the validation configuration. We are now going to add a validation in order to let the build fail when the repository is dirty.
First, we add a ValidationProperties section to the configuration section in our pom.xml file:
<validationProperties> <!-- verify that the current repository is not dirty --> <validationProperty> <name>validating git dirty</name> <value>${git.dirty}</value> <shouldMatchTo>false</shouldMatchTo> </validationProperty> </validationProperties>
Secondly, we have to activate the validation. This is done in the executions section of the git-commit-id plugin:
<execution> <id>validate-the-git-infos</id> <goals> <goal>validateRevision</goal> </goals> <phase>package</phase> </execution>
I did not commit the changes I have made to the pom.xml file yet, so my repository is dirty. Build the application with Maven. As expected, the build fails with the following error:
Validation 'validating git dirty' failed! Expected 'true' to match with 'false'!
If we now change the shouldMatchTo into true and run the build again, the build is successful.
Summary
The Maven git commit id plugin is indispensible and should be added to each Maven project. It automatically creates the versioning information during a build in order that you are able to verify the versioning information when an application is deployed. No discussions anymore about which version of the software is running on an environment.
Share this:
Related
Recommend
-
60
README.md vim-maven-plugin This plugin provides convenient functions to Apache Maven project. Main features: Detects your editing...
-
24
English | 简体中文 造轮子目的 使用yml配置来简化MyBatis Generator默认使用的xml配置 支持注释生成、lombok、swagger...
-
21
jetty-maven-plugin静态文件保存不了 妙音 posted @ 2014年12月31日 11:08 in java , 29856 阅读 ...
-
9
Apache Maven SCM Publish Plugin The maven-scm-publish-plugin is a utility plugin to allow publishing Maven website to any supported SCM. The primary goal was to have an utility plugin to allow Apache projects to publish Maven website...
-
13
Various tips for using this plugin URL format You must use a scm url format: scm:<scm_provider><delimiter><provider_specific_part> Example for svn: scm...
-
10
Usage The maven-scm-publish-plugin submits modified content to SCM, sites being the most classical case. The standard use for sites is: mvn -Preporting site site:stagemvn scm-publish:publis...
-
14
Files Permalink Latest commit message Commit time
-
9
Reporting Code Coverage using Maven and JaCoCo plugin1. INTRODUCTION Code coverage is a metric indicating which percentage of lines of code are executed when running automated tests.
-
7
Splitting Unit and Integration Tests using Maven and Surefire plugin Orlando L Otero | Apr 8, 2019 | integration testing
-
1
SpringBoot使用git-commit-id-maven-plugin打包 - Naylor - 博客园 git-commit-id-maven-plugin 是一个maven 插件,用来在打包的时候...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK